Java Networking
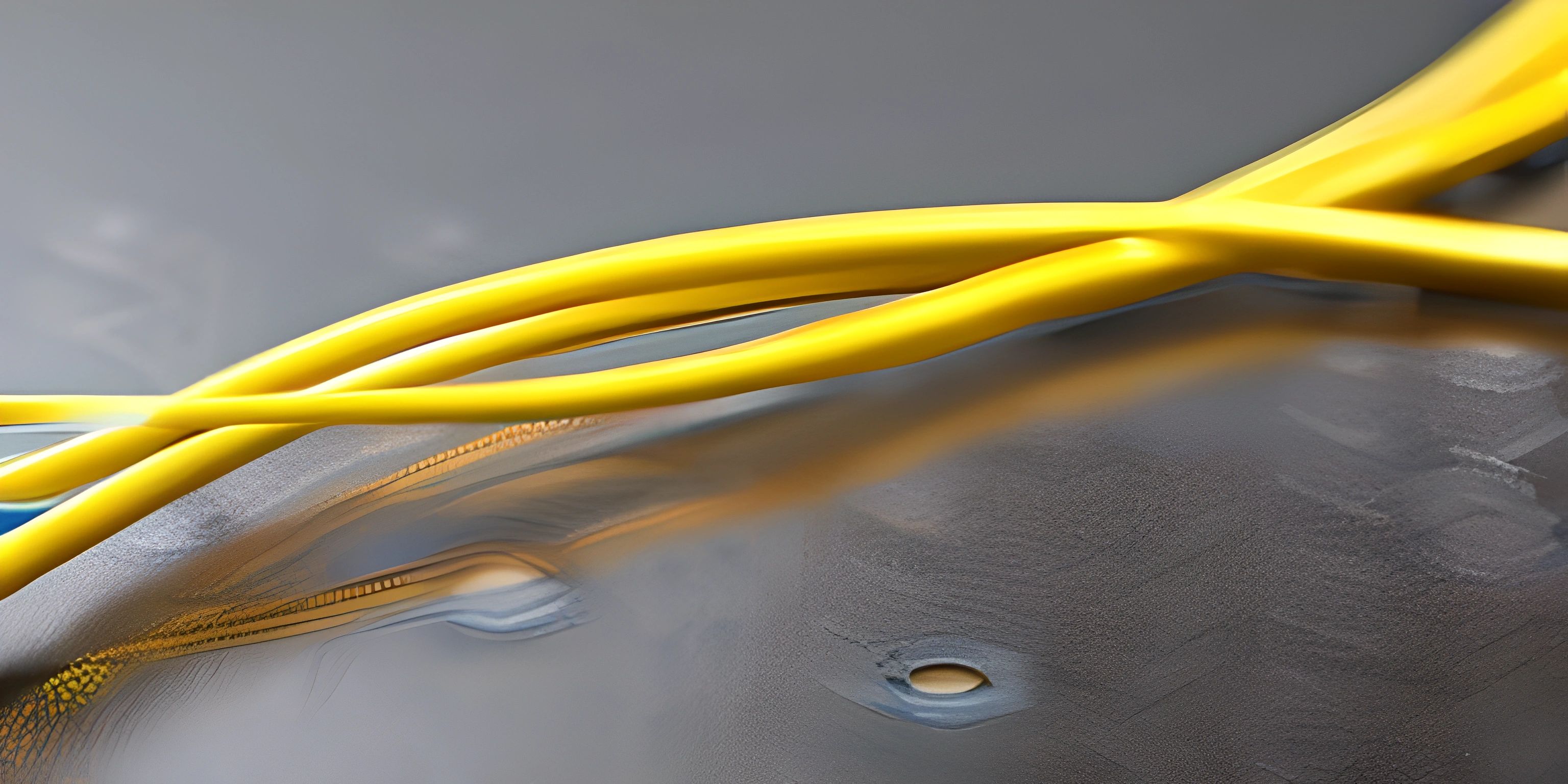
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Networking is the foundation of many applications, allowing them to communicate and exchange data with other programs and systems. In Java, creating network-based applications is straightforward, thanks to its robust set of built-in libraries and classes. Strap in, and let's dive into the world of Java networking!
Java Networking Basics
Java networking revolves around the concept of sockets. A socket is an endpoint for communication between two devices, usually over the Internet. Java provides classes for both server-side and client-side sockets to facilitate communication.
InetAddress
The InetAddress
class represents an Internet Protocol (IP) address. It's used with sockets to identify devices on a network. Here's an example of getting the IP address of a domain using InetAddress
:
import java.net.InetAddress; public class IPAddressExample { public static void main(String[] args) { try { InetAddress address = InetAddress.getByName("www.example.com"); System.out.println("IP Address: " + address.getHostAddress()); } catch (Exception e) { e.printStackTrace(); } } }
Sockets and ServerSockets
The Socket
class is used for creating client-side sockets to establish a connection with a server. The ServerSocket
class is responsible for creating server-side sockets and listening for incoming client connections. Let's create a simple client-server example using Java sockets.
Server:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.io.PrintWriter; import java.net.ServerSocket; import java.net.Socket; public class ChatServer { public static void main(String[] args) { try { ServerSocket serverSocket = new ServerSocket(12345); System.out.println("Server is waiting for clients..."); Socket clientSocket = serverSocket.accept(); System.out.println("Client connected!"); BufferedReader input = new BufferedReader(new InputStreamReader(clientSocket.getInputStream())); PrintWriter output = new PrintWriter(clientSocket.getOutputStream(), true); String message; while ((message = input.readLine()) != null) { System.out.println("Client: " + message); output.println("Server: " + message.toUpperCase()); } clientSocket.close(); serverSocket.close(); } catch (Exception e) { e.printStackTrace(); } } }
Client:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.io.PrintWriter; import java.net.Socket; public class ChatClient { public static void main(String[] args) { try { Socket socket = new Socket("localhost", 12345); BufferedReader consoleInput = new BufferedReader(new InputStreamReader(System.in)); BufferedReader serverInput = new BufferedReader(new InputStreamReader(socket.getInputStream())); PrintWriter serverOutput = new PrintWriter(socket.getOutputStream(), true); String message; while ((message = consoleInput.readLine()) != null) { serverOutput.println(message); System.out.println(serverInput.readLine()); } socket.close(); } catch (Exception e) { e.printStackTrace(); } } }
In this example, the server and client communicate by sending and receiving messages. The server converts the messages to uppercase and sends them back to the client.
URL and HttpURLConnection
The URL
class represents a Uniform Resource Locator, commonly used for accessing web resources. The HttpURLConnection
class is used for connecting to a URL and performing HTTP requests like GET and POST. Here's an example of fetching the content of a web page using URL
and HttpURLConnection
:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class URLExample { public static void main(String[] args) { try { URL url = new URL("https://www.example.com/"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); BufferedReader input = new BufferedReader(new InputStreamReader(connection.getInputStream())); String line; StringBuilder content = new StringBuilder(); while ((line = input.readLine()) != null) { content.append(line); } input.close(); connection.disconnect(); System.out.println(content.toString()); } catch (Exception e) { e.printStackTrace(); } } }
Conclusion
Java provides a comprehensive set of classes for networking, making it easy to create network-based applications. By utilizing classes like InetAddress
, Socket
, ServerSocket
, URL
, and HttpURLConnection
, you can create feature-rich applications that communicate and exchange data over the Internet. With these basics under your belt, you're well on your way to mastering Java networking!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).