Round-Robin Load Balancing
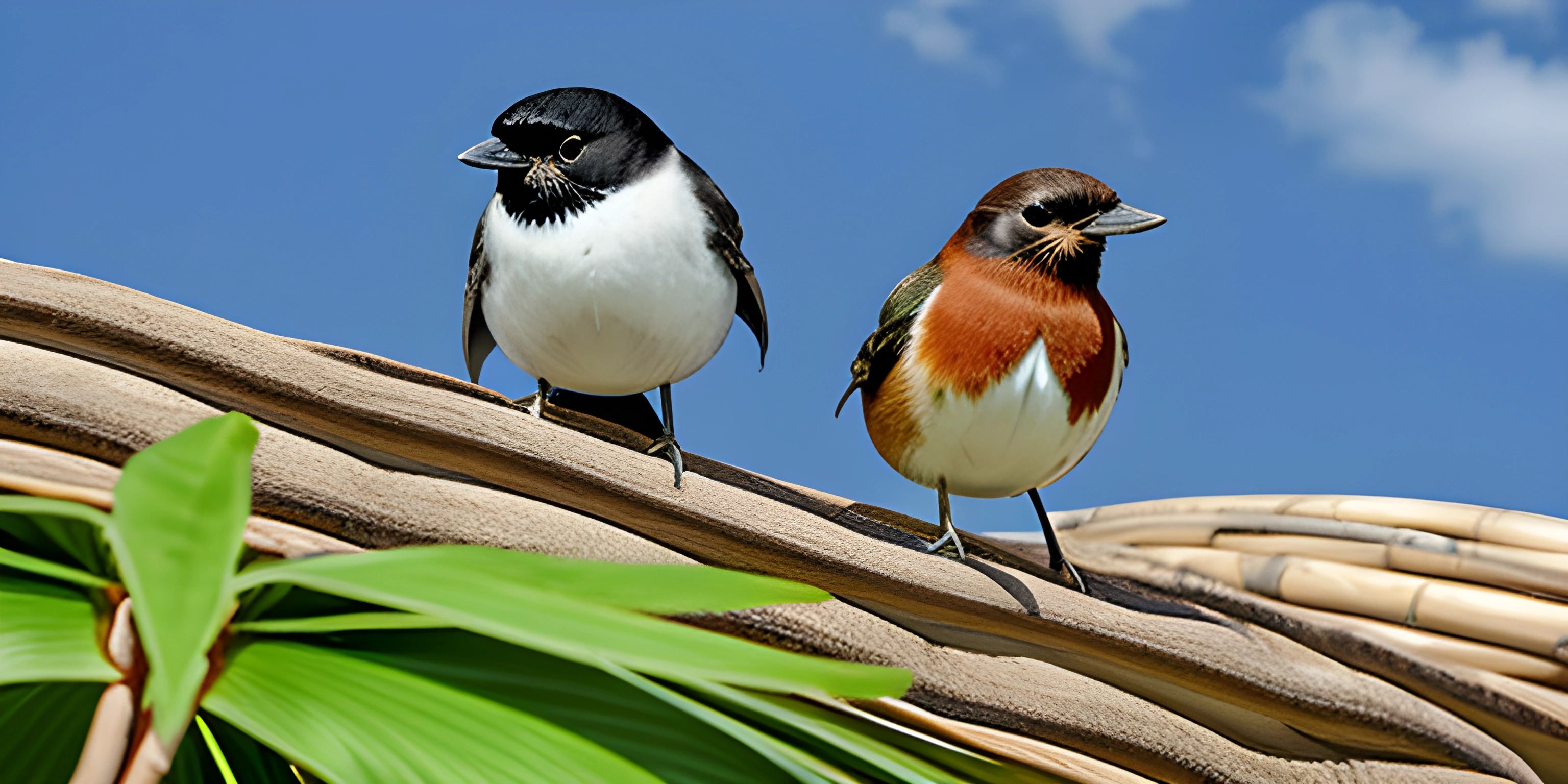
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a school teacher and you have a stack of papers to grade. You could do it all yourself, but that would take a lot of time and effort. So, you decide to distribute the papers amongst your colleagues, giving each one a paper in turn, until all the papers are divided equally. This is essentially how Round-Robin Load Balancing works; it's a simple yet effective method of distributing network load among multiple servers.
How It Works
The Round-Robin algorithm is one of the simplest load balancing methods. It works by distributing client requests across a group of servers on a rotating basis. The first request goes to the first server, the second to the second, and so on. When it reaches the last server in the list, it starts over again with the first server.
Let's put it in code terms, using the Python language:
def distribute_requests(requests, servers): for i in range(len(requests)): server = servers[i % len(servers)] server.handle(requests[i])
In this Python snippet, we distribute each request to a server in a round-robin fashion. The modulo operator (%
) ensures we loop back to the first server after reaching the end of the list.
Pros and Cons
Like every technique in computer science, Round-Robin Load Balancing has its strengths and weaknesses.
The good: It's simple to implement, transparent, and doesn't require complex data structures or algorithms. It's a democratic approach, treating all servers as equals, regardless of their individual capabilities.
The bad: It doesn't take into account the servers' actual load or capacity. What if one of the servers is a Raspberry Pi and another is a high-end server? The round-robin method would stress the Raspberry Pi with the same number of requests as the high-end server, likely overloading it.
When to use it?
Round-Robin Load Balancing is great when you have servers with similar capacities and the tasks they are handling are relatively uniform. However, for more complex scenarios where tasks vary greatly in resource requirements or servers have different processing capacities, a more robust load balancing method might be needed.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is Round-Robin Load Balancing?
Round-Robin Load Balancing is a simple method for distributing network load across multiple servers. It works by assigning client requests to servers in a rotating order, starting with the first server and moving to the next with each request. When it reaches the last server, it loops back to the first.
What are the advantages of Round-Robin Load Balancing?
The main advantages of Round-Robin Load Balancing are its simplicity, transparency, and fair distribution of load. It doesn't require complex data structures or algorithms to implement and treats all servers as equals, regardless of their individual capabilities.
What are the disadvantages of Round-Robin Load Balancing?
The main disadvantage of Round-Robin Load Balancing is that it doesn't consider the actual load or capacity of the servers. This can lead to problems if the servers have different capacities or the tasks they are handling vary greatly in resource requirements.
When should I use Round-Robin Load Balancing?
Round-Robin Load Balancing is most effective when you have servers with similar capacities and the tasks they are handling are relatively uniform. In more complex scenarios, where tasks vary greatly in resource requirements or servers have different processing capacities, a more advanced load balancing method might be more appropriate.