Java Control Flow
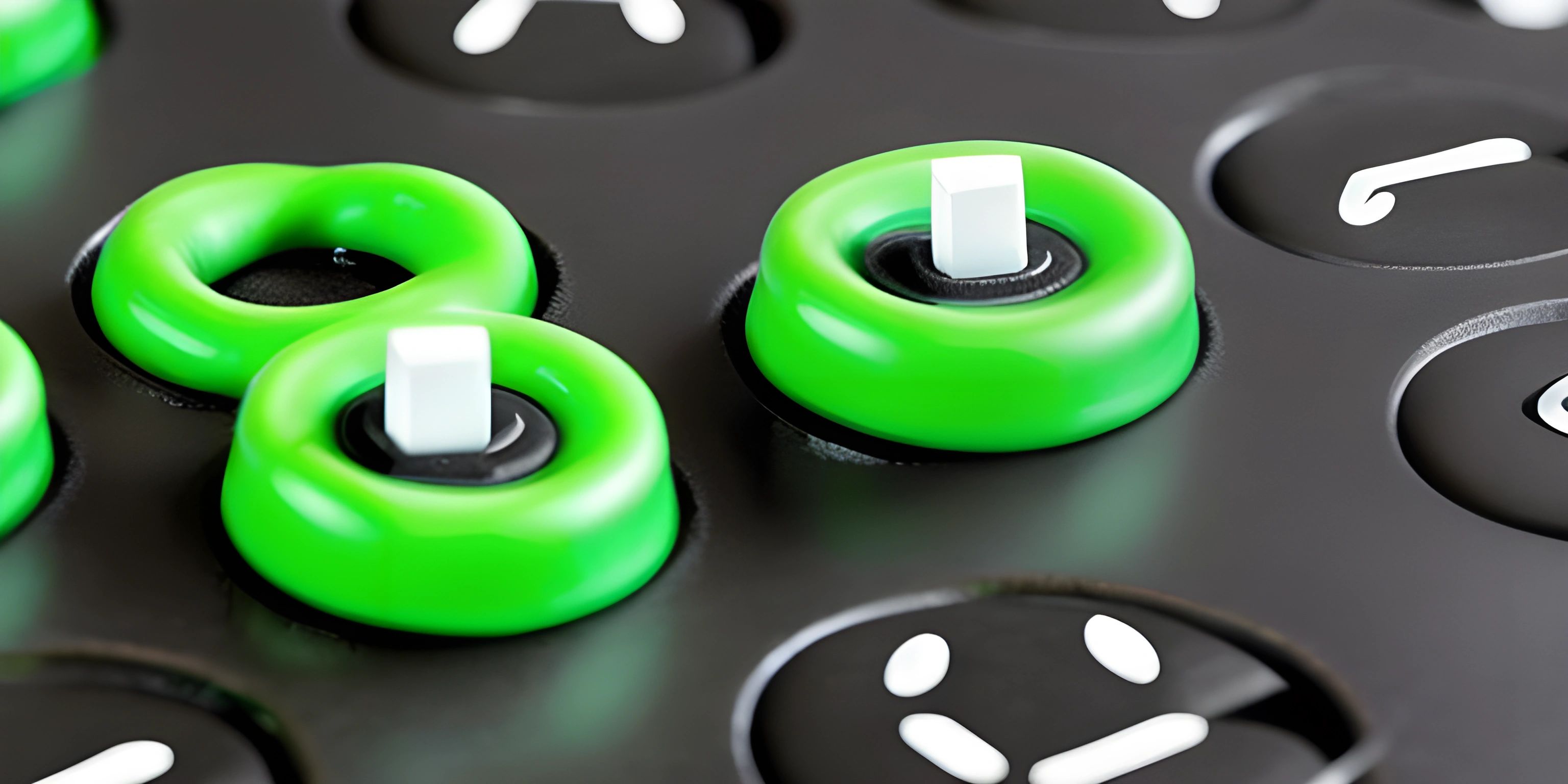
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Control flow structures are essential in programming, as they allow your code to make decisions and repeat tasks. In Java, there are several control flow structures, such as if
, else
, for
, and while
loops. Let's dive into each of these to see how they work and how to use them effectively.
If and Else
The if
statement is used to test a condition and execute a block of code if the condition is true. The else
statement can be added after an if
statement to execute a different block of code when the condition is false. Let's see an example in Java:
int age = 18; if (age >= 18) { System.out.println("You are an adult!"); } else { System.out.println("You are not an adult."); }
In this example, the if
statement checks whether the age is greater than or equal to 18. If the condition is true, it prints "You are an adult!", otherwise, it prints "You are not an adult.".
For Loop
A for
loop is used to repeatedly execute a block of code for a specific number of times. The loop consists of three parts: initialization, condition, and increment/decrement. Here's an example:
for (int i = 0; i < 5; i++) { System.out.println("Hello, " + i); }
In this example, the loop starts with i
equal to 0, runs as long as i
is less than 5, and increments i
by 1 after each iteration. This prints "Hello, 0", "Hello, 1", ..., "Hello, 4".
While Loop
A while
loop is similar to a for
loop but is used when the number of iterations is unknown or depends on a condition. The loop continues executing as long as the condition is true. Here's an example:
int counter = 0; while (counter < 5) { System.out.println("Counter: " + counter); counter++; }
In this example, the loop will continue to execute as long as the counter
variable is less than 5, printing "Counter: 0", "Counter: 1", ..., "Counter: 4".
Break and Continue
Sometimes, you may want to exit a loop prematurely or skip an iteration. You can use the break
statement to exit a loop, and the continue
statement to skip to the next iteration. Here's an example:
for (int i = 0; i < 10; i++) { if (i % 2 == 0) { continue; // skip even numbers } if (i == 7) { break; // exit the loop when i is 7 } System.out.println("Number: " + i); }
In this example, the loop prints odd numbers from 1 to 5, because it skips even numbers and exits when i
is equal to 7.
FAQ
What are the main control flow structures in Java?
The main control flow structures in Java are if
, else
, for
, while
, break
, and continue
.
How do you execute a block of code only when a condition is true?
You can use an if
statement to execute a block of code only when a condition is true.
What is the difference between a `for` loop and a `while` loop?
A for
loop is used when you want to repeat an action for a specific number of times, whereas a while
loop is used when you want to repeat an action as long as a condition is true.
How can you exit a loop early or skip an iteration?
You can use the break
statement to exit a loop early, and you can use the continue
statement to skip an iteration.