Java Polymorphism and Abstract Classes
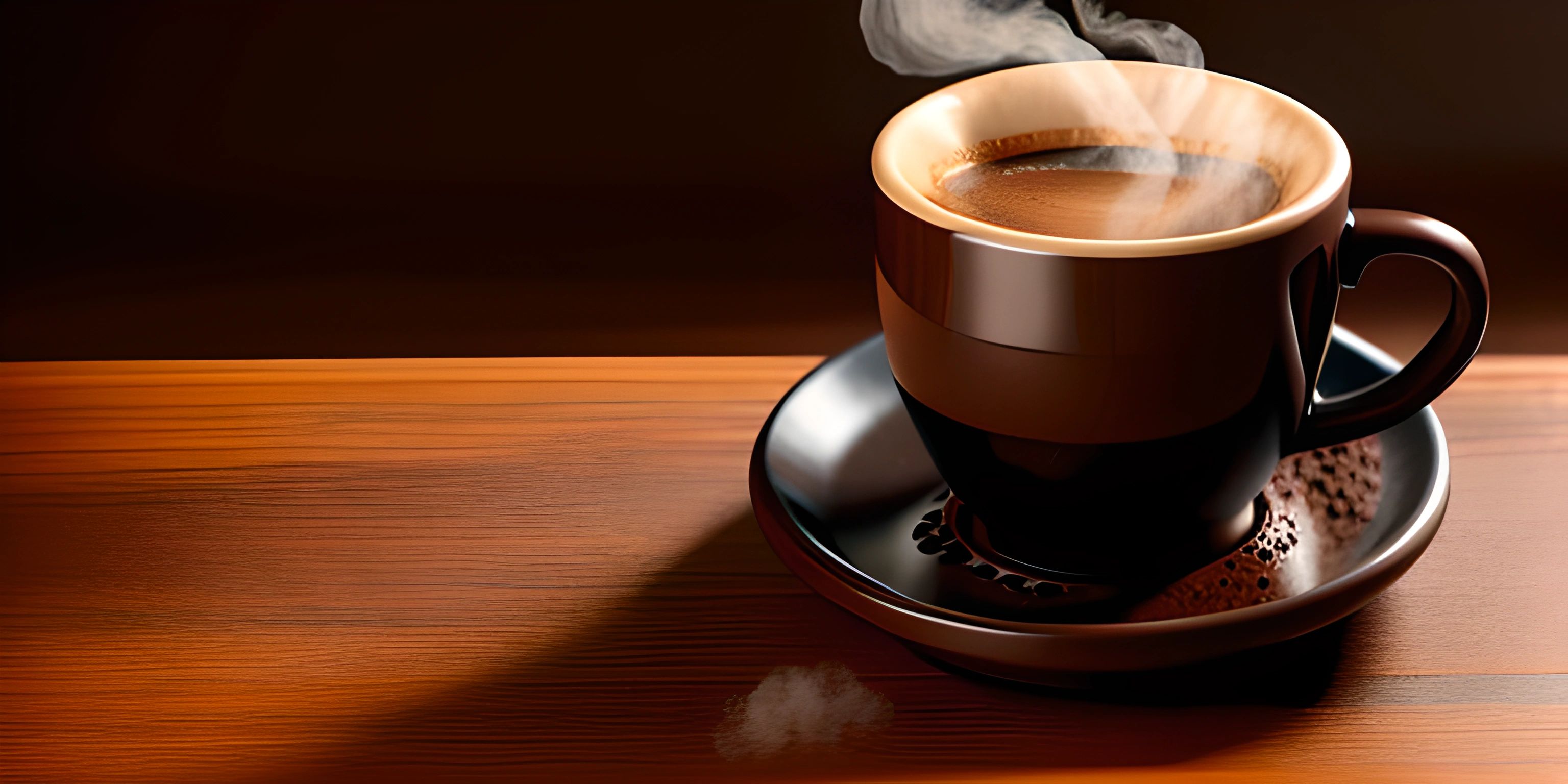
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of Java, polymorphism is like a chameleon changing colors to adapt to its environment. It allows objects to take on different forms and exhibit multiple behaviors, making code more flexible and easier to maintain. One way to achieve this magical feat is through abstract classes. Buckle up, and let's dive into the fascinating world of polymorphism and abstract classes in Java!
Polymorphism in Java
Polymorphism is one of the four fundamental principles of object-oriented programming (OOP), alongside encapsulation, inheritance, and abstraction. In Java, polymorphism allows objects of different classes to be treated as objects of a common superclass. This makes it possible to write reusable code that can work with multiple types, providing flexibility and reducing repetition.
Imagine you run a zoo, and you want to create a program to keep track of all the animals and their daily activities. You have a wide variety of animals, including lions, elephants, and penguins. All these animals share some common traits, like eating, sleeping, and making sounds, but the way they do these things is different. Polymorphism allows you to handle these animals in a unified way, based on their shared traits, without caring about the specific animal type.
Abstract Classes
Abstract classes in Java are like blueprints for creating concrete classes. They can have fields, methods, and constructors, just like any other class, but they cannot be instantiated directly. Instead, they serve as a base for other classes to inherit from and implement the abstract methods.
To declare an abstract class, you simply use the abstract
keyword before the class
keyword. Abstract methods are also declared using the abstract
keyword, and they have no body – just a semicolon at the end.
Here's an example of an abstract class representing a generic animal:
public abstract class Animal { // Instance variables private String name; // Constructor public Animal(String name) { this.name = name; } // Getter for name public String getName() { return name; } // Abstract methods public abstract void eat(); public abstract void sleep(); public abstract void makeSound(); }
Implementing Polymorphism with Abstract Classes
Now that we have our Animal
abstract class, let's see how to use it to implement polymorphism.
First, we'll create concrete classes for different animals, like a Lion
class, an Elephant
class, and a Penguin
class. Each of these classes will extend the Animal
class and provide their own implementation of the abstract methods.
Here's an example of the Lion
class:
public class Lion extends Animal { // Constructor public Lion(String name) { super(name); } // Implementing abstract methods public void eat() { System.out.println(getName() + " the lion is eating meat."); } public void sleep() { System.out.println(getName() + " the lion is sleeping under a tree."); } public void makeSound() { System.out.println(getName() + " the lion roars!"); } }
With the concrete animal classes in place, we can now use polymorphism to work with different animal types in a unified way. For example, let's create an array of Animal
objects and loop through them, calling the eat()
method on each one:
public static void main(String[] args) { // Create an array of Animal objects Animal[] animals = { new Lion("Simba"), new Elephant("Dumbo"), new Penguin("Pingu") }; // Loop through the array and call the eat() method on each animal for (Animal animal : animals) { animal.eat(); } }
This code will produce the following output:
Simba the lion is eating meat. Dumbo the elephant is eating leaves. Pingu the penguin is eating fish.
As you can see, polymorphism through abstract classes allows us to work with different animal types using their shared traits, while still allowing each animal to exhibit its unique behavior. This makes our code more flexible, modular, and easier to maintain. Now go forth and unleash the power of polymorphism in your Java projects!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).