Common JavaScript Errors, Explanations, and Solutions
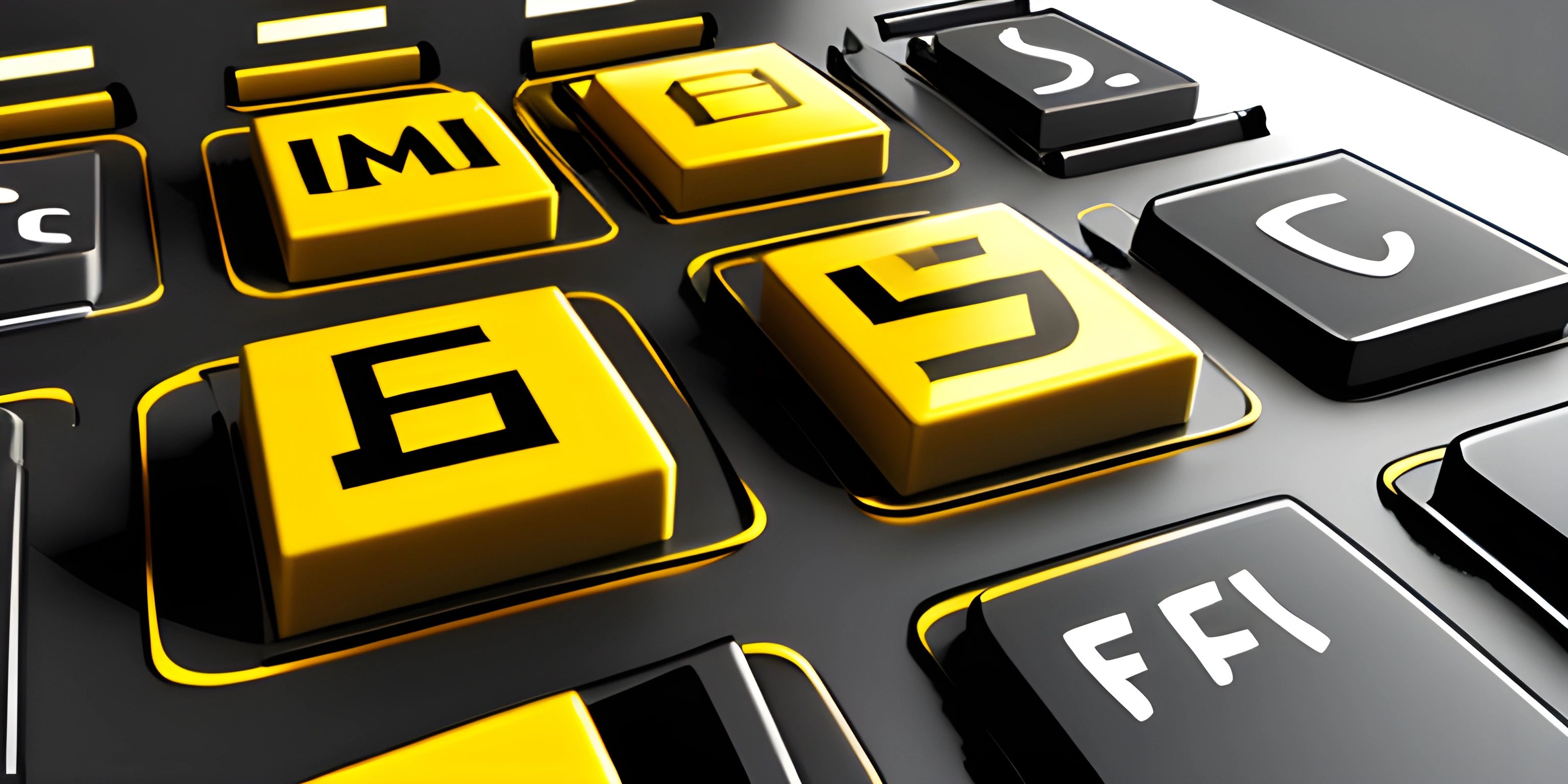
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming can be an exhilarating adventure, but sometimes we hit a bump in the road: errors. JavaScript, like any other language, has its fair share of common errors. But fear not, intrepid coder! We're here to help you navigate these murky waters and get you back on track.
ReferenceError: "X" is not defined
This error occurs when you attempt to use a variable or function that hasn't been defined yet.
Example:
console.log(message); let message = "Hello, world!";
Explanation: JavaScript is trying to access the message
variable before it's been assigned a value.
Solution: Make sure to declare and initialize your variables before using them.
let message = "Hello, world!"; console.log(message);
TypeError: "X" is not a function
This error pops up when you're trying to call something as a function, but JavaScript disagrees with your assessment of its functionhood.
Example:
let greeting = "Hello, world!"; greeting();
Explanation: greeting
is a string, not a function, so trying to call it like one results in a TypeError.
Solution: Double-check your variable assignments and ensure you're calling a function, not another data type.
function greeting() { console.log("Hello, world!"); } greeting();
SyntaxError: Unexpected token "X"
SyntaxErrors occur when the JavaScript interpreter encounters something it doesn't expect or can't understand.
Example:
function greeting() { console.log("Hello, world!"; }
Explanation: There's a missing closing parenthesis after the console.log
statement, causing a syntax error.
Solution: Ensure your code is properly formatted and all parentheses, brackets, and braces are correctly paired.
function greeting() { console.log("Hello, world!"); }
RangeError: Maximum call stack size exceeded
This error occurs when you've got too many function calls happening in a recursive loop, causing a stack overflow.
Example:
function countdown(n) { console.log(n); countdown(n - 1); } countdown(5);
Explanation: The countdown
function keeps calling itself without a base case to stop the recursion, leading to a stack overflow.
Solution: Implement a base case to stop the recursion when a certain condition is met.
function countdown(n) { if (n <= 0) { return; } console.log(n); countdown(n - 1); } countdown(5);
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is a ReferenceError in JavaScript?
A ReferenceError occurs when you try to use a variable or function that hasn't been defined yet. To fix it, make sure you declare and initialize your variables before using them.
What does the "TypeError: X is not a function" error mean?
This error occurs when you attempt to call something as a function, but it's not actually a function (e.g., a string, number, or object). To fix this, double-check your variable assignments and ensure you're calling a function, not another data type.
How can I fix a SyntaxError in JavaScript?
SyntaxErrors are caused by incorrect or unexpected syntax in your code. To fix them, carefully review your code for proper formatting, correct pairing of parentheses, brackets, braces, and any other syntax issues.
What causes a RangeError with the message "Maximum call stack size exceeded"?
This error occurs when there are too many function calls happening in a recursive loop, causing a stack overflow. To fix it, ensure you have a base case in your recursive function to stop the recursion when a certain condition is met.