Stack Overflow in JavaScript
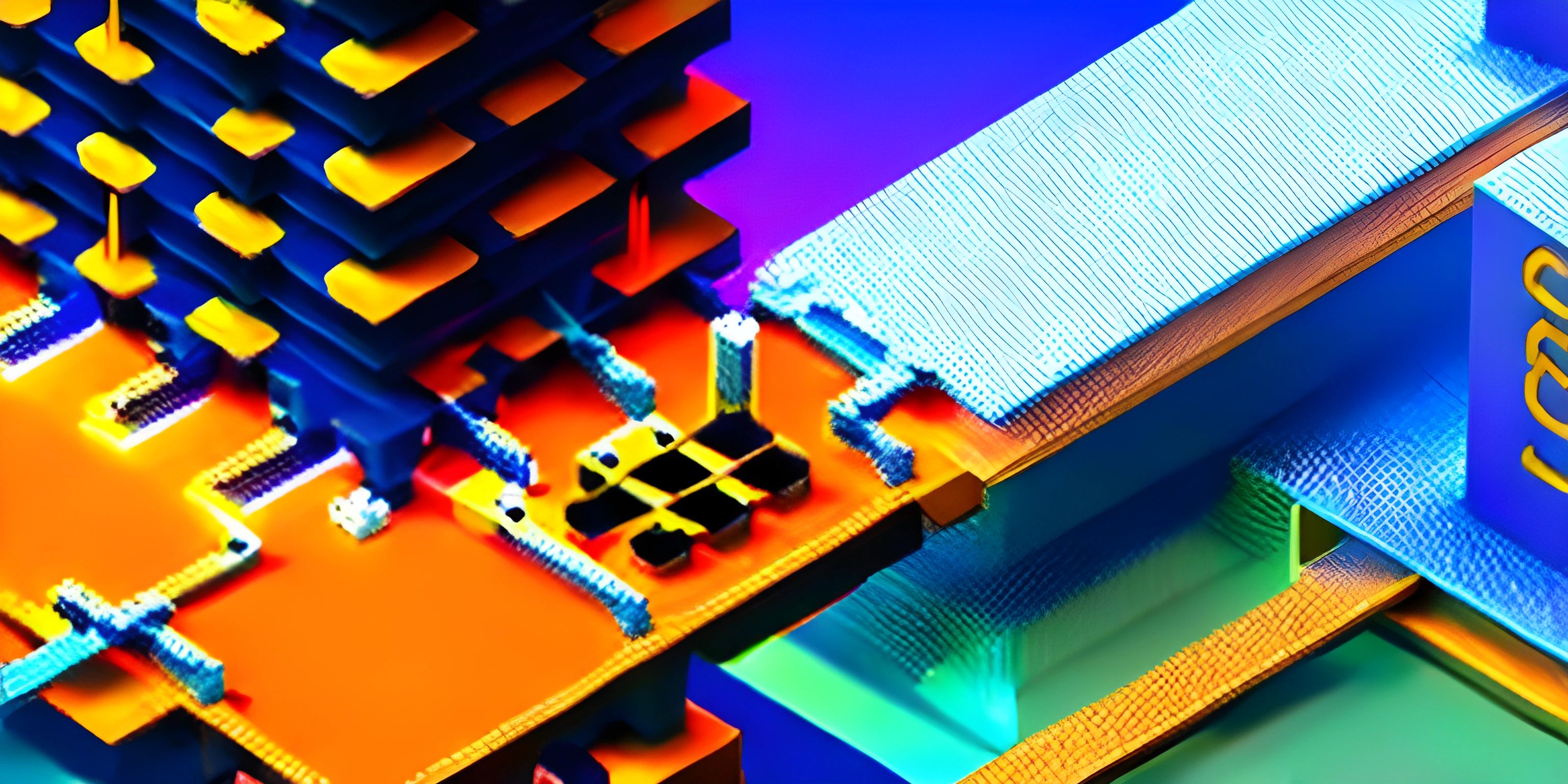
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
For any JavaScript programmer, stumbling upon a 'RangeError: Maximum call stack size exceeded' is both a rite of passage and a nightmare. This dreaded error message signals the birth of a Stack Overflow in your code. But fear not, intrepid coder! We're here to unravel the mystery of this beast.
What is a Stack Overflow?
To understand what a Stack Overflow is, you need to know about the call stack. In JavaScript, when functions are invoked, they are piled on the call stack. Once they're done running, they're popped off.
Imagine you're building a tower of lego blocks. You keep adding more and more blocks, the tower grows taller. But, if you keep adding blocks without ever taking any away, the tower becomes unstable and collapses. That's essentially a Stack Overflow - your call stack tower collapses under the weight of too many function calls.
The Trouble with Recursion
One common cause of Stack Overflow is uncontrolled recursion. Recursion is a method where a function calls itself to solve a problem. It's like a dog chasing its tail. It's fun to watch, but if the dog never catches its tail, it will keep spinning until it gets dizzy and falls over - a Stack Overflow.
Here's a classic example of a Stack Overflow-causing recursive function:
function stackOverflow() { stackOverflow(); } stackOverflow();
This function will keep calling itself without an exit condition, leading to a Stack Overflow. It's the equivalent of that dizzy, tail-chasing dog.
Fixing a Stack Overflow
So, how do we prevent our JavaScript dog from chasing its tail into oblivion? The key is to give it an exit condition.
function stackUnderflow(n) { if (n === 0) { return; } n--; stackUnderflow(n); } stackUnderflow(10);
In this example, the function stops calling itself when n
equals 0, preventing a Stack Overflow.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is a Stack Overflow?
In JavaScript, a Stack Overflow occurs when there are too many function calls piled on the call stack. This often happens when a function keeps calling itself, or there is a loop that triggers numerous function calls, without an exit condition.
How can I prevent a Stack Overflow?
Always ensure that recursive functions have an exit condition. This helps prevent the call stack from hitting its maximum limit. Additionally, be cautious with loops that trigger function calls, as they could rapidly fill up the call stack.
How can I identify a Stack Overflow?
A Stack Overflow in JavaScript typically throws a 'RangeError: Maximum call stack size exceeded' error. This indicates that too many function calls have piled onto the call stack, exceeding its limit.
What is the call stack?
The call stack in JavaScript is a data structure that records the function calls in your program. When a function is called, it's added to the call stack. When it's done executing, it's popped off the call stack.
What is recursion?
Recursion in programming is a method where a function calls itself to solve a problem. However, if a recursive function doesn't have a proper exit condition, it can lead to a Stack Overflow.