Common Java Runtime Exceptions and Solutions
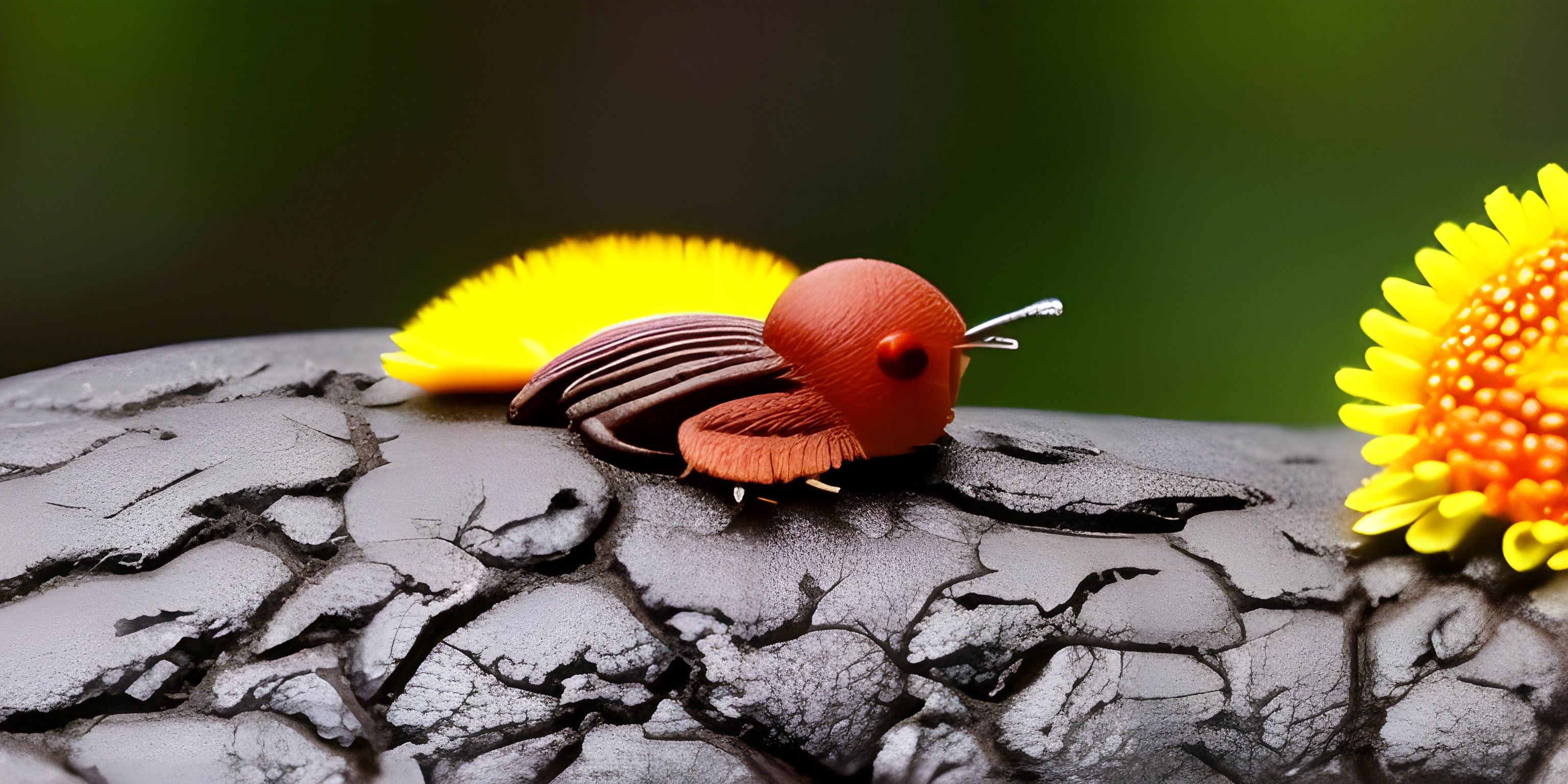
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
While working with Java, you'll likely come across various runtime exceptions that can be frustrating and time-consuming to resolve. Runtime exceptions occur during the execution of a program and are usually caused by logic errors in your code. To save you from pulling your hair out, we've compiled a list of common Java runtime exceptions, their causes, and solutions to help you fix them quickly.
NullPointerException
The infamous NullPointerException occurs when you try to access a method or a field of an object that is null
. In other words, you're trying to use an object that hasn't been initialized yet.
Example
public class Main { public static void main(String[] args) { String myString = null; System.out.println(myString.length()); } }
Solution
To fix a NullPointerException, make sure to initialize your objects before using them or add a null-check to ensure you don't try to access methods or fields of a null
object.
public class Main { public static void main(String[] args) { String myString = "Hello, World!"; System.out.println(myString.length()); } }
ArrayIndexOutOfBoundsException
An ArrayIndexOutOfBoundsException occurs when you try to access an array element with an index that is either negative or greater than or equal to the array's length.
Example
public class Main { public static void main(String[] args) { int[] myArray = {1, 2, 3}; System.out.println(myArray[3]); } }
Solution
To fix an ArrayIndexOutOfBoundsException, ensure that you're using a valid index within the bounds of the array. You can use the .length
property of the array to avoid going out of bounds.
public class Main { public static void main(String[] args) { int[] myArray = {1, 2, 3}; System.out.println(myArray[2]); } }
ClassCastException
A ClassCastException occurs when you try to cast an object of one class to a different class that it cannot be cast to. This usually happens when you're using inheritance or implementing interfaces.
Example
public class Main { public static void main(String[] args) { Object myString = "Hello, World!"; Integer myInteger = (Integer) myString; } }
Solution
To fix a ClassCastException, make sure you're casting objects to their correct types. You can use the instanceof
operator to check if an object is an instance of a specific class before casting.
public class Main { public static void main(String[] args) { Object myString = "Hello, World!"; if (myString instanceof Integer) { Integer myInteger = (Integer) myString; } else { System.out.println("Cannot cast to Integer"); } } }
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a runtime exception in Java?
A runtime exception in Java is an exception that occurs during the execution of a program, usually due to logic errors in the code. These exceptions are not checked by the compiler, meaning they can cause your program to crash unexpectedly if not handled properly.
How can I fix a NullPointerException in Java?
To fix a NullPointerException in Java, make sure to initialize your objects before using them or add a null-check to ensure you don't try to access methods or fields of a null
object.
How can I avoid an ArrayIndexOutOfBoundsException in Java?
To avoid an ArrayIndexOutOfBoundsException in Java, ensure that you're using a valid index within the bounds of the array. You can use the .length
property of the array to avoid going out of bounds or use loops with proper iteration conditions.