JavaScript Essentials
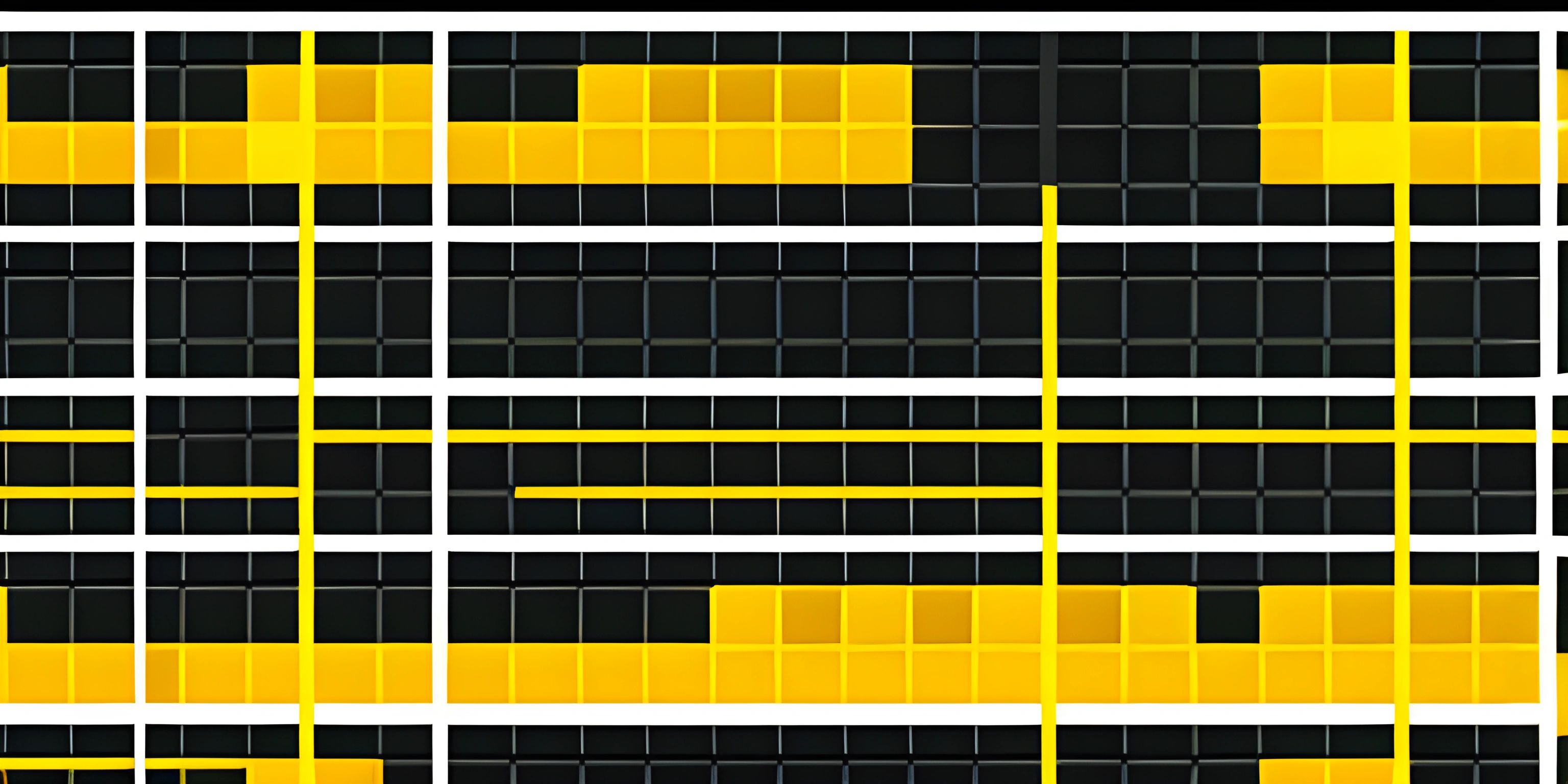
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JavaScript is the lifeblood of modern web development, bringing dynamic content and interactivity to websites. This guide will introduce you to the core concepts and techniques necessary to become a successful JavaScript developer. Fasten your seatbelts, and let's get started!
Variables and Data Types
JavaScript supports several data types, including strings, numbers, booleans, objects, and arrays. Variables are used to store and manipulate these data values. In JavaScript, you can declare a variable using the var
, let
, or const
keywords.
var myString = "Hello, world!"; let myNumber = 42; const myBoolean = true;
var
is an older way to declare variables with function scope, while let
and const
are block-scoped and recommended for modern JavaScript.
Functions
Functions are reusable blocks of code that you can define and call by name. Functions are essential for organizing and reusing code throughout your program. In JavaScript, you can define functions using the function
keyword or using arrow functions (=>
).
// Traditional function declaration function greet(name) { return "Hello, " + name + "!"; } // Arrow function const greet = (name) => "Hello, " + name + "!";
Objects and Arrays
Objects are collections of key-value pairs, called properties, where keys are strings and values can be of any data type. Arrays are ordered collections of elements, which can also include any data type. JavaScript provides various methods for manipulating and accessing these collections.
// Object const person = { name: "Alice", age: 30, hobbies: ["reading", "hiking", "photography"], }; // Accessing properties console.log(person.name); // Alice console.log(person["age"]); // 30 // Array const numbers = [1, 2, 3, 4, 5]; // Accessing elements console.log(numbers[0]); // 1
Control Flow
Control flow statements in JavaScript allow you to perform actions based on specific conditions. The most common control flow statements include if
statements, for
loops, and while
loops.
// If statement if (age > 18) { console.log("You're an adult."); } else { console.log("You're a minor."); } // For loop for (let i = 0; i < 5; i++) { console.log(i); } // While loop let count = 0; while (count < 5) { console.log(count); count++; }
Document Object Model (DOM)
The Document Object Model (DOM) is a representation of a web page's structure, allowing JavaScript to interact with and modify the page. You can access and manipulate the DOM using built-in functions, such as getElementById
, querySelector
, and addEventListener
.
// Accessing an element by ID const heading = document.getElementById("main-heading"); // Modifying the DOM heading.textContent = "Hello, JavaScript!"; // Adding an event listener heading.addEventListener("click", () => { alert("You clicked the heading!"); });
Mastering these core concepts sets you on the path to becoming a skilled JavaScript developer. Keep exploring and experimenting to strengthen your JavaScript prowess!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What are the primary data types in JavaScript?
JavaScript supports several data types, including strings, numbers, booleans, objects, and arrays.
How are functions defined in JavaScript?
Functions can be defined using the function
keyword or using arrow functions (=>
).
What are the common control flow statements in JavaScript?
The most common control flow statements in JavaScript include if
statements, for
loops, and while
loops.
How can you interact with a web page's structure using JavaScript?
You can interact with a web page's structure using the Document Object Model (DOM) and built-in functions like getElementById
, querySelector
, and addEventListener
.