Dealing with Recursion on Large Inputs in JavaScript
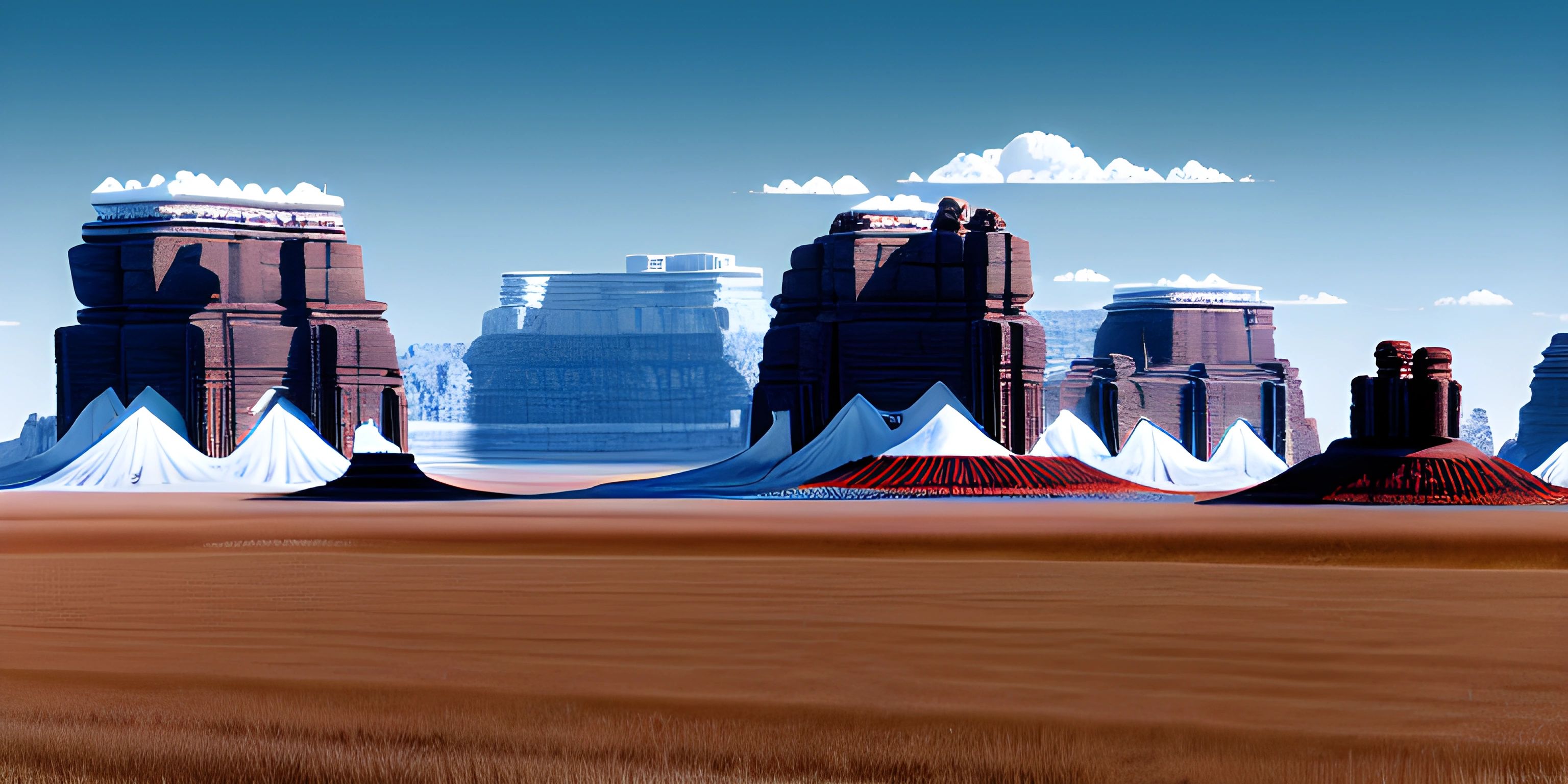
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever tried to climb a ladder that stretches up to the moon? Me neither, but I imagine it would feel similar to dealing with recursion on large inputs in JavaScript. It's a seemingly insurmountable task, with the risk of toppling over (or in JavaScript terms, a stack overflow). But fear not! With the right tools and techniques, we can safely navigate this challenge. So get your space helmet on, and let's dive in!
Recursion and The Call Stack
First, let's understand how recursion works in JavaScript. Recursion is a technique in which a function calls itself to solve a problem. It's like a Russian doll: a bigger problem contains smaller versions of the same problem within it.
In JavaScript, each recursive call adds a new frame to the call stack. This is a pile of function calls that the JavaScript engine needs to keep track of. It's like a stack of pancakes: the most recent pancake (function call) gets added to the top, and gets eaten (executed) first.
function countDownFrom(n) { if (n > 0) { console.log(n); return countDownFrom(n - 1); } return n; } countDownFrom(5); // outputs 5, 4, 3, 2, 1
But what happens when we try to eat too many pancakes (recursive calls)? That's right, we get a stomachache (stack overflow).
Stack Overflow and Large Inputs
A stack overflow occurs when there are too many function calls on the call stack. The JavaScript engine has a limited amount of memory to store these function calls, so if your recursive function has a large input, it can quickly fill up the call stack and cause an error.
countDownFrom(100000); // Uncaught RangeError: Maximum call stack size exceeded
Tail Call Optimization
Luckily, there's a technique to handle recursion with large inputs: tail call optimization. Here's the gist: if the last action of a function is to call itself, the JavaScript engine can clear the current stack frame before making the recursive call. This means the call stack won't grow with each recursive call.
This sounds like a magic trick, right? But there's a catch: JavaScript does not fully support tail call optimization. However, you can enable strict mode to use it.
"use strict"; function countDownFrom(n, acc = 0) { if (n <= 0) { return acc; } return countDownFrom(n - 1, acc + n); } countDownFrom(100000); // no stack overflow error
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is recursion in JavaScript?
In JavaScript, recursion is a technique where a function calls itself to solve a problem. It's typically used for tasks that can be divided into simpler sub-tasks of the same type.
What is a stack overflow?
A stack overflow occurs when there are too many function calls in the call stack. This error often happens when you try to use recursion on large inputs, as each recursive call adds a new frame to the call stack.
What is tail call optimization?
Tail call optimization is a technique that allows recursive calls to be handled more efficiently by the JavaScript engine. If the last action of a function is to call itself, the engine can clear the current stack frame before making the recursive call. This avoids increasing the call stack size and prevents stack overflow errors.
Why doesn't JavaScript fully support tail call optimization?
The lack of full support for tail call optimization in JavaScript is mainly due to compatibility issues. However, you can enable strict mode in your code to utilize tail call optimization.
Can recursion be used with all types of problems?
While recursion is a powerful technique, it's not suitable for all types of problems. It's best used for tasks that can be broken down into simpler sub-tasks of the same type. Additionally, due to the risk of stack overflow errors, recursion should be used carefully with large inputs.