JavaScript Variables
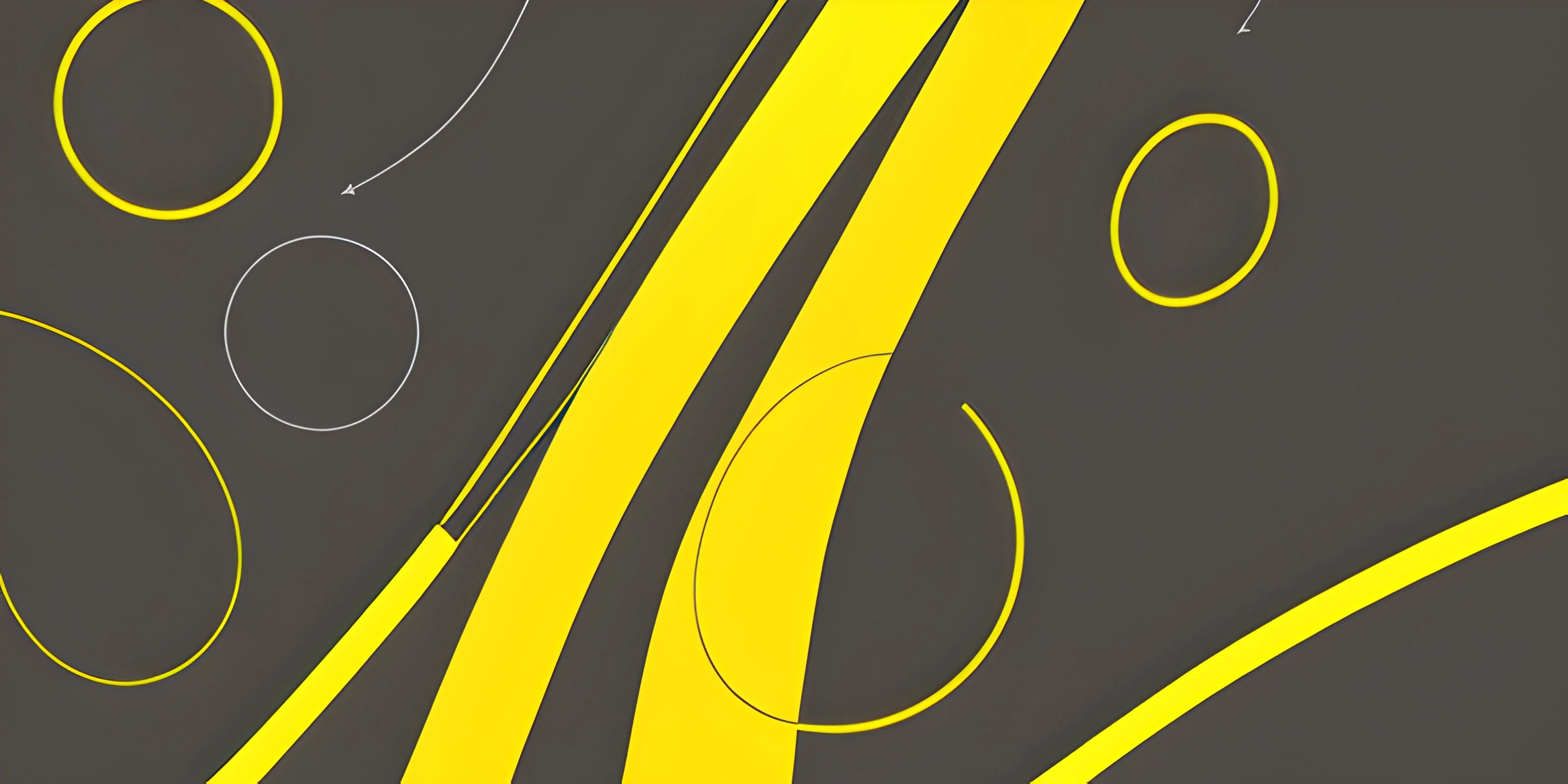
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
One of the core aspects of programming is dealing with data, and in JavaScript, variables are the containers that hold this data. In this article, we'll dive into the world of JavaScript variables and learn how to manipulate data effectively.
Variable Declaration
In JavaScript, there are three ways to declare a variable: var
, let
, and const
. Each method has its own quirks, but the most commonly used are const
and let
.
var
var
is the oldest method of declaring variables in JavaScript. Variables declared using var
are function-scoped, meaning they are accessible within the entire function they are declared in.
Here's an example:
function exampleFunction() { var x = "I am a variable!"; console.log(x); // Output: "I am a variable!" }
However, using var
can lead to some unexpected behavior, especially when it comes to hoisting (when JavaScript moves variable declarations to the top of their scope). This can result in variables being accessible even before they are declared:
function badExample() { console.log(y); // Output: undefined var y = "I am a different variable!"; }
Because of these quirks, it's generally recommended to use let
or const
instead.
let
let
is a more modern way of declaring variables in JavaScript. Unlike var
, variables declared with let
are block-scoped, which means they are only accessible within the block they are declared in. This can help prevent accidental manipulation of variables outside of their intended scope.
Here's an example using let
:
function betterExample() { let z = "I am a better variable!"; console.log(z); // Output: "I am a better variable!" }
And unlike var
, let
doesn't suffer from hoisting issues:
function noHoistingHere() { console.log(a); // ReferenceError: a is not defined let a = "No hoisting for me!"; }
const
const
is another modern way of declaring variables in JavaScript. Like let
, const
is block-scoped, but with one key difference: variables declared with const
cannot be reassigned. This makes const
great for declaring constant values or variables that should remain unchanged throughout the execution of your code.
An example with const
:
function constantExample() { const b = "I am a constant variable!"; console.log(b); // Output: "I am a constant variable!" b = "Can I change?"; // TypeError: Assignment to constant variable. }
Manipulating Data with Variables
Now that we've covered the basics of declaring variables, let's move on to manipulating the data stored in them. In JavaScript, you can perform various operations on variables to manipulate their values.
Arithmetic Operations
JavaScript supports basic arithmetic operations, such as addition, subtraction, multiplication, and division. You can perform these operations on variables as well:
let num1 = 10; let num2 = 20; let sum = num1 + num2; // 30 let difference = num1 - num2; // -10 let product = num1 * num2; // 200 let quotient = num1 / num2; // 0.5
String Concatenation
In JavaScript, you can concatenate strings using the +
operator:
let firstName = "John"; let lastName = "Doe"; let fullName = firstName + " " + lastName; // "John Doe"
Updating Variable Values
You can update the value of a variable using the assignment operator (=
) and any relevant operation:
let counter = 0; counter = counter + 1; // 1 counter += 1; // 2 counter++; // 3
Conclusion
JavaScript variables are essential building blocks for manipulating data in your code. By understanding the differences between var
, let
, and const
, and learning how to perform basic operations on variables, you'll be well on your way to mastering JavaScript programming. As you continue to learn, make sure to explore other data types and more advanced data manipulation techniques to further increase your programming prowess.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Ownership and Borrowing (psst, it's free!).
FAQ
What is a variable in JavaScript?
A variable in JavaScript is a container that holds a specific value, allowing you to store and manipulate data. Variables are essential building blocks in any programming language, and they can hold different types of values such as numbers, strings, and objects.
How do I declare a variable in JavaScript?
In JavaScript, you can declare a variable using the var
, let
, or const
keywords. var
is the old way of declaring variables, while let
and const
were introduced in ES6 (ECMAScript 2015). Here's an example of declaring variables with each keyword:
var oldVariable = "I'm old-fashioned!"; let newVariable = "I'm more modern!"; const constantVariable = "I can't be changed!";
What is the difference between `let` and `const`?
Both let
and const
are used to declare variables in modern JavaScript, but they have different behaviors. let
allows you to change the value of the variable later in your code, while const
creates a constant variable, which means its value cannot be changed once it's assigned. Here's a comparison:
let changeable = "I can change!"; changeable = "See? I just changed!"; const unchangeable = "I can't change!"; // This line would throw an error: // unchangeable = "Trying to change me will cause an error!";
How do I assign a value to a variable?
To assign a value to a variable in JavaScript, you use the equal sign =
. This is called the assignment operator. For example:
let myVariable; myVariable = "This value is now assigned to myVariable.";
Can a variable hold different types of values?
Yes! In JavaScript, variables are not restricted to a specific type of value. This means you can store different types of values in the same variable, such as numbers, strings, and objects. JavaScript is a dynamically-typed language, which means that the type of a variable is determined by the value it holds:
let versatileVariable = 42; versatileVariable = "Now I'm a string!"; versatileVariable = { property: "And now I'm an object!" };