Understanding Low-Level Programming Languages
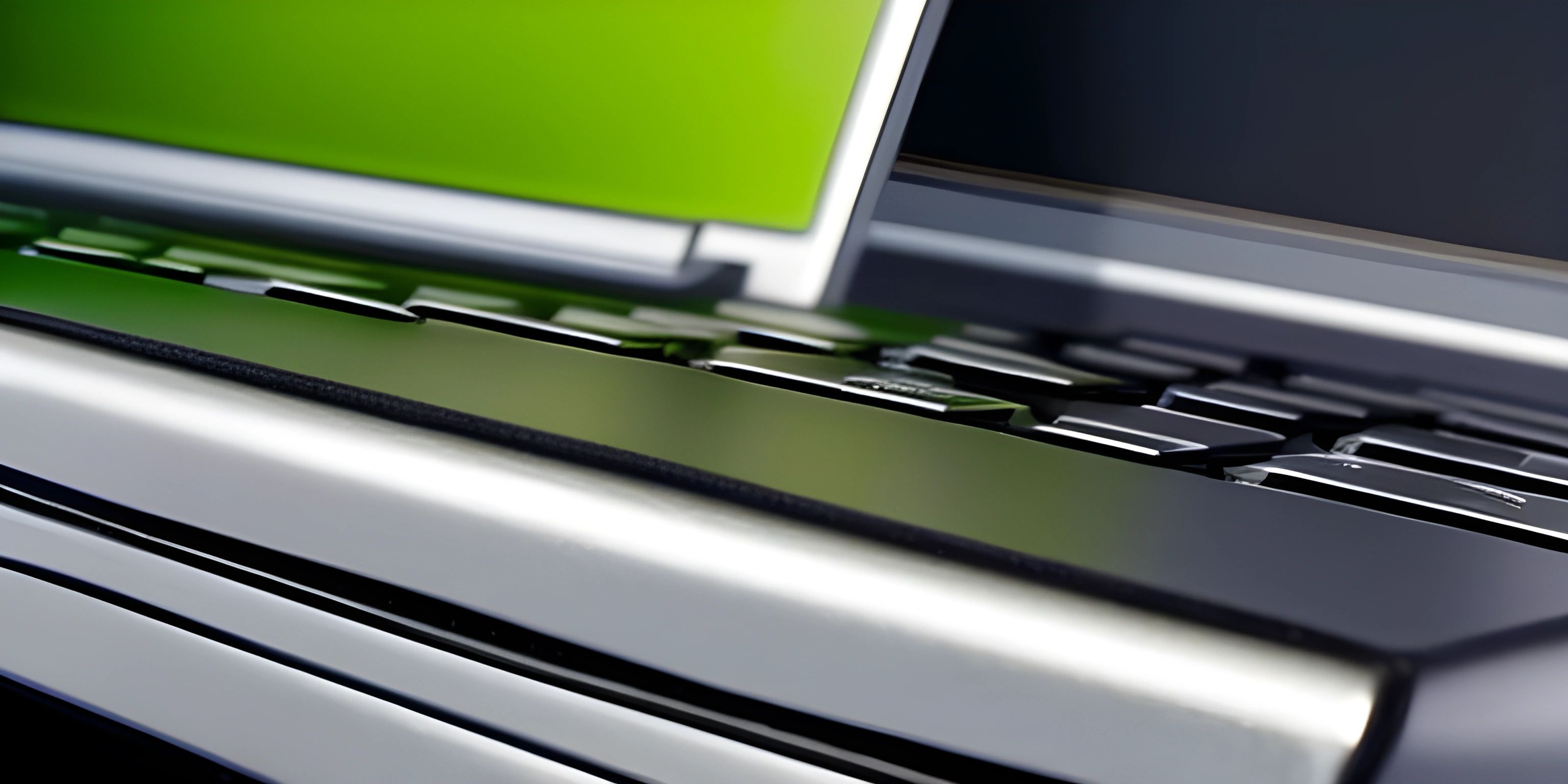
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Alright, fellow digital adventurers! Ready to dive into the depths of the programming ocean? Today, we're exploring low-level programming languages. Imagine you're sailing a ship. High-level languages (like Python or JavaScript) are your GPS and autopilot. In contrast, low-level languages are like navigating by the stars and manually steering the wheel. It's raw, it's real, and it's as close to the machine as you can get without cracking it open.
What Are Low-Level Programming Languages?
Low-level programming languages provide little to no abstraction from a computer's instruction set architecture. They are closer to machine code, the binary 1s and 0s that computers use to perform operations. The two main categories of low-level languages are machine language and assembly language.
Machine Language
Machine language, also known as machine code, is the lowest level of code that can be executed by a computer's central processing unit (CPU). It consists entirely of binary digits (bits), which are combinations of 0s and 1s. Writing in machine language is like speaking directly to the computer in its native tongue. Here's a bite-sized example:
10110000 01100001
This isn't exactly human-friendly, but it's the purest form of communication with a machine.
Assembly Language
Assembly language sits one level above machine code and uses mnemonics (symbolic names) for instructions and addresses. It makes writing code a bit easier by representing binary instructions in a more readable form. Here's an example using x86 assembly language:
MOV AL, 61h ; Move hexadecimal value 61 into register AL
This line of code moves the hexadecimal value 61h
into the AL register. Much more comprehensible than a string of 0s and 1s, right?
Why Use Low-Level Languages?
You might be wondering why anyone would choose to steer a ship manually when autopilot exists. There are several compelling reasons:
- Performance and Efficiency: Low-level code runs faster and uses fewer resources because it is closer to the hardware. This is crucial for performance-critical applications such as operating systems and embedded systems.
- Hardware Control: Low-level languages allow direct manipulation of hardware components. This is essential for systems programming and developing drivers, firmware, and more.
- Understanding and Learning: Writing in low-level languages can provide a deeper understanding of how computers work. It demystifies what happens under the hood and enhances problem-solving skills.
- Legacy Systems: Many older systems and software are written in low-level languages. Maintaining or updating these systems requires knowledge of these languages.
Examples of Low-Level Languages
Although there aren't many low-level languages compared to high-level ones, a few have stood the test of time due to their critical roles in computing.
Assembly Language
Assembly language is still widely used in systems programming, especially for developing and optimizing operating system kernels, device drivers, and embedded systems. Here's another example that demonstrates a simple addition operation:
MOV AX, 5 ; Move 5 into register AX ADD AX, 3 ; Add 3 to the value in AX
In this snippet, we move the value 5 into the AX register and then add 3 to it. The register AX now holds the value 8. It's like basic arithmetic but done directly on the CPU!
C Language
While not strictly low-level, C is often considered a "low-level high-level language" due to its ability to manipulate hardware directly, its minimal runtime, and its efficiency. C is used to write operating systems, embedded software, and performance-critical applications. Here's a quick example of a C program:
#include <stdio.h> int main() { int a = 5; int b = 3; int result = a + b; printf("Result: %d\n", result); // Output the result return 0; }
This C code adds two integers and prints the result. While it's not as close to the hardware as assembly, it still offers significant control and efficiency.
Use Cases for Low-Level Languages
Low-level languages shine in specific scenarios where performance, efficiency, and direct hardware control are paramount.
Operating Systems
Operating systems like Windows, Linux, and macOS have their kernels written in low-level languages. The kernel is the core part of the OS, managing resources and hardware communication. Low-level languages' efficiency and control make them ideal for this purpose.
Embedded Systems
Embedded systems are specialized computing systems that perform dedicated functions within larger mechanical or electrical systems. They are everywhere: in your microwave, car, and even your smartwatch. These systems often require highly efficient code to run on limited hardware resources, making low-level languages a perfect fit.
Game Development
In game development, performance is crucial. Low-level languages are used to write game engines and performance-critical components. They allow developers to optimize resource usage and manage hardware directly to achieve smooth gameplay.
Device Drivers and Firmware
Device drivers act as translators between hardware devices and the operating system. Firmware is software programmed into read-only memory, providing low-level control for a device's specific hardware. Both require precise control over hardware, which low-level languages provide.
When to Avoid Low-Level Languages
While low-level languages have their advantages, they aren't always the best choice. Here are some reasons to avoid them:
- Complexity: Writing low-level code is significantly more complex and error-prone than high-level code.
- Development Time: It takes longer to write, debug, and maintain low-level code.
- Portability: Low-level code is often platform-specific, making it harder to port to different systems.
- Readability: Low-level code can be difficult for others to read and understand, reducing collaboration efficiency.
Conclusion
Low-level programming languages offer a unique blend of performance, control, and efficiency. They are the backbone of many critical systems and applications. While not always the easiest to work with, they provide invaluable insights into the inner workings of computers and offer unparalleled control over hardware.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are low-level programming languages?
Low-level programming languages are languages that provide little to no abstraction from a computer's instruction set architecture. They are closer to machine code, the binary instructions that computers use to perform operations.
Why would someone use a low-level language?
Low-level languages are used for performance-critical applications, direct hardware control, understanding computer internals, and maintaining legacy systems. They offer efficiency and control that high-level languages cannot match.
Can you give an example of a low-level language?
Assembly language is a common example of a low-level language. It uses mnemonics to represent machine code instructions and allows direct hardware manipulation.
What are some use cases for low-level languages?
Low-level languages are used in operating systems, embedded systems, game development, and device drivers. They are ideal for scenarios requiring high performance and direct hardware control.
What are the drawbacks of using low-level languages?
The main drawbacks are complexity, longer development time, lower portability, and reduced readability. Low-level code can be challenging to write, debug, and maintain, making high-level languages more suitable for many applications.