Understanding the x86 Assembly Language
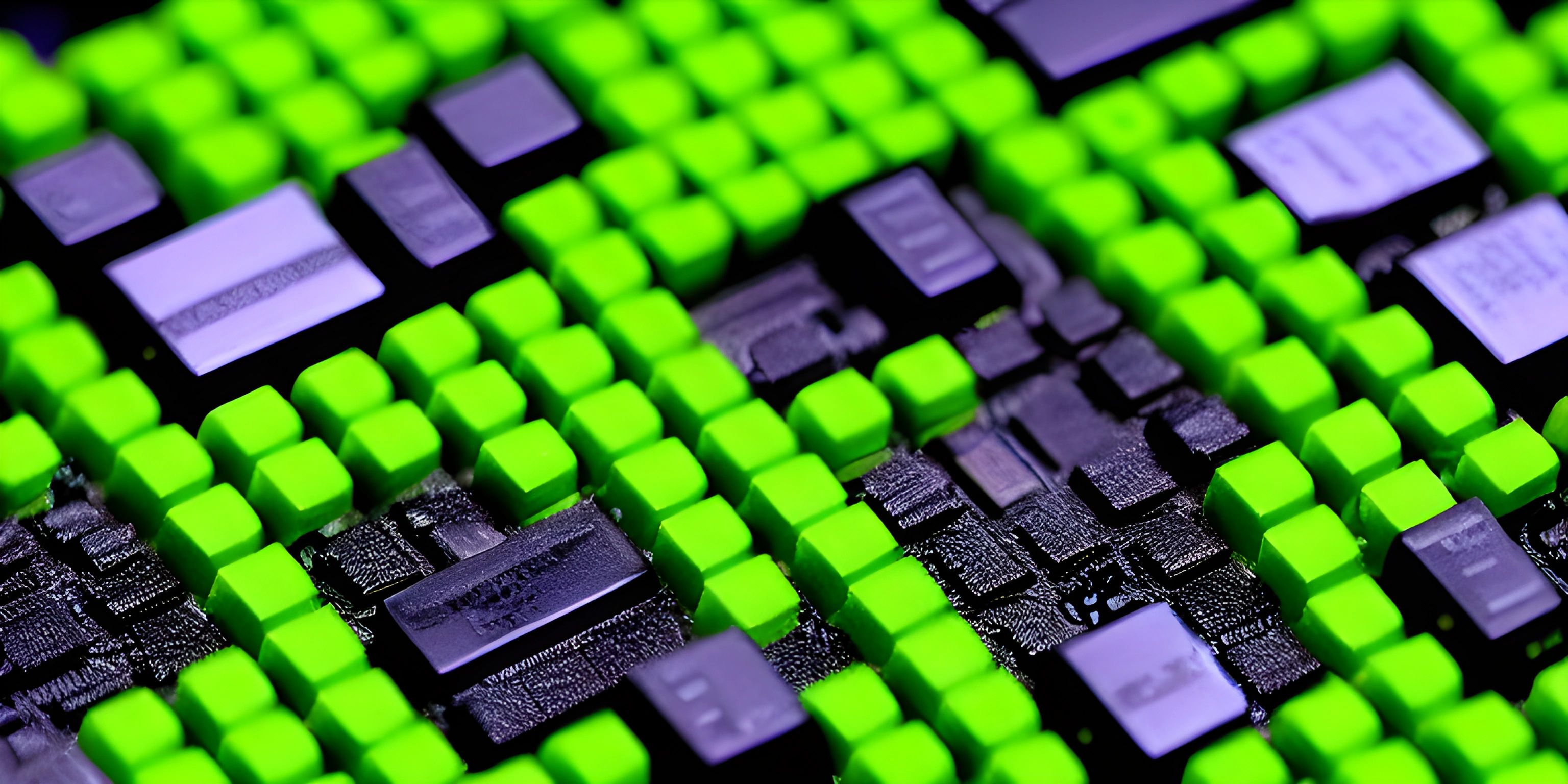
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to understanding how a computer operates at its most fundamental level, there's no better place to start than assembly language. No, we're not talking about a school assembly or IKEA furniture instructions, but x86 assembly language - the language your computer's CPU speaks!
So, grab your virtual screwdriver as we delve into the nuts and bolts of the x86 assembly language. But don't worry, we won't be taking apart any physical computers here, just the abstract ones in our minds.
Getting Acquainted with Assembly
Assembly language is a type of low-level programming language that is specific to a particular computer architecture. In our case, we're focusing on the x86 architecture - a widely used architecture in personal computers.
Assembly language is like the "machine's native tongue". It's how you talk directly to the processor, with no translator in the middle.
Instructions
One of the basic building blocks of assembly language is the instruction. Instructions are like the verbs of the language, they indicate what action the CPU should take. Here's a simple example:
MOV EAX, 10
This instruction tells the CPU to move the value 10 into the EAX register. Think of it as telling your computer, "Hey, remember the number 10 for me in your EAX memory slot, okay?"
Registers
Speaking of registers, they're like the computer's short-term memory. In the x86 assembly, we have several registers like EAX, EBX, ECX, and more. Registers are the places where all the magic happens, they're like the CPU's workbench where it carries out the instructions.
Variables
In higher-level languages, we have variables. Assembly language has something similar, but instead of variables, we use memory addresses. It's like saying, "Hey computer, can you remember this data for me at this memory address?" Unfortunately, the computer doesn't have a catchy name for every memory location like we do with variables, so we have to remember the exact address.
Control Flow
Just like other programming languages, assembly language also has control flow statements. We can use JMP, JE, and JNE instructions to control the flow of the program. Control flow is like a traffic cop in your program, directing the flow of instructions based on certain conditions.
CMP EAX, 10 JE label
This code compares the value in the EAX register to 10. If they are equal (JE stands for "Jump if Equal"), it will jump to the location marked as "label".
Working with assembly language requires patience, precision, and a good memory. But don't worry, with practice, you'll become fluent in this esoteric language and gain a deeper understanding of how your computer works.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the x86 assembly language?
The x86 assembly language is a low-level programming language specific to the x86 computer architecture. It consists of a set of instructions that directly communicate with the CPU. It's like the "native tongue" of the computer.
What are instructions in assembly language?
Instructions in assembly language are like the verbs of the language. They dictate what action the CPU should perform. Examples include MOV (move), ADD (add), SUB (subtract), and CMP (compare).
What are registers in the context of assembly language?
Registers are like the computer's short-term memory. They're where the CPU performs most of its operations. In the x86 assembly, we have several registers like EAX, EBX, ECX, and more.
How does control flow work in assembly language?
Control flow in assembly language is managed using JMP (jump), JE (jump if equal), and JNE (jump if not equal) instructions. These instructions allow the program to branch and execute different code paths based on certain conditions.
How does assembly language handle variables?
In assembly language, instead of variables, we use memory addresses. So, instead of giving a piece of data a name, we tell the computer to store it at a specific location in memory.