Using FFI to Call Assembly Functions from Rust
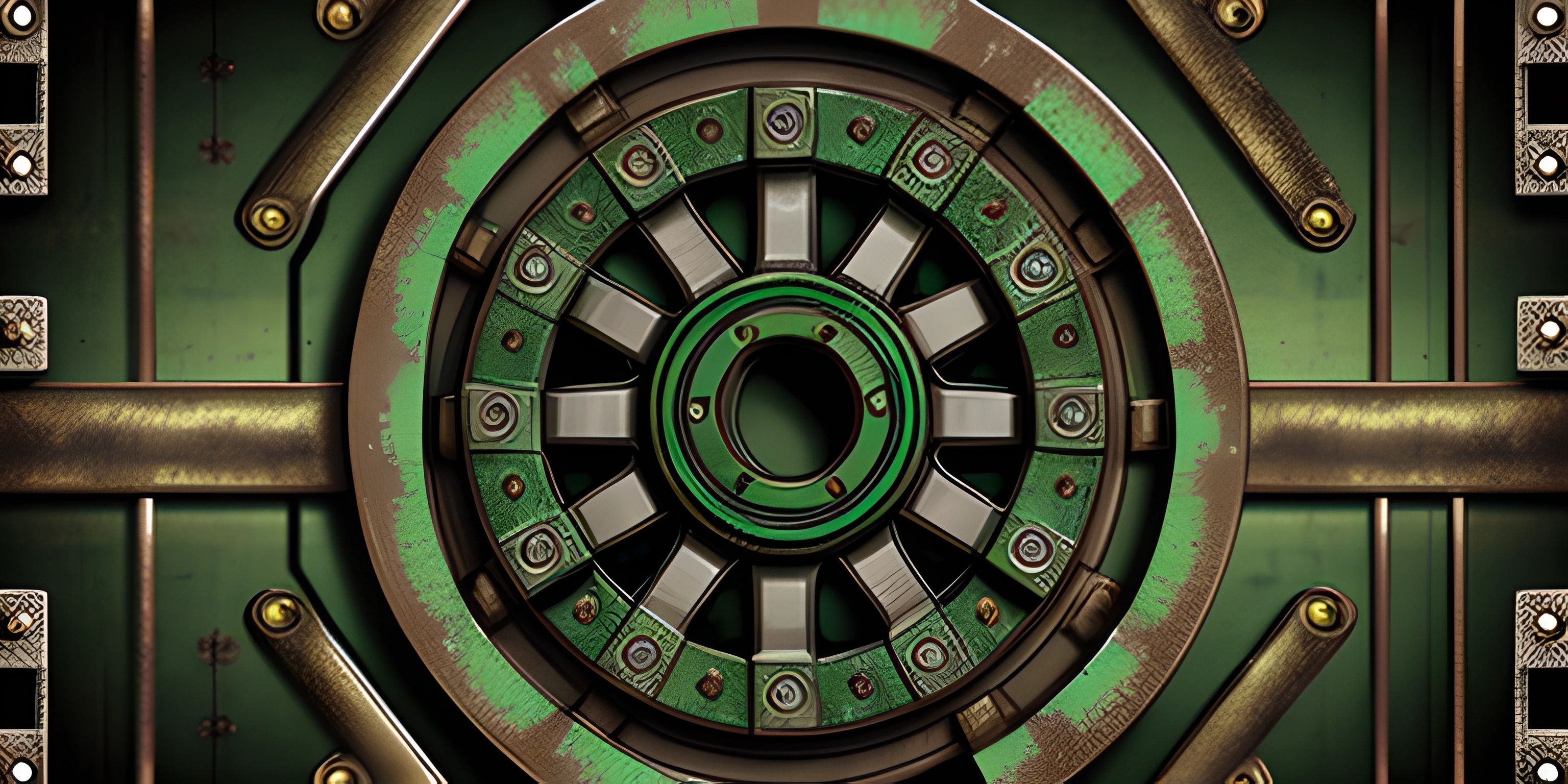
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Rust is a fantastic language that offers a balance of high-level abstractions and low-level control. However, sometimes you need to squeeze every last drop of performance from your code. That's when you turn to assembly, and Rust's foreign function interface (FFI) makes that possible.
Foreign Function Interface (FFI)
FFI allows you to call functions written in other languages from Rust, and vice versa. It's a powerful way to leverage existing code or to use lower-level languages for performance-critical functions.
Using FFI, we can call assembly functions from Rust. But first, let's create a simple assembly function that we want to use in our program.
Writing Assembly Functions
Let's say we want to write a function that adds two 32-bit integers together. Here's how that might look in x86 assembly:
; add_numbers.s .global add_numbers add_numbers: addl %edi, %esi movl %esi, %eax ret
This assembly code defines a function called add_numbers
that adds two 32-bit integers and returns the result. It uses x86 calling convention, which passes the first two arguments in the %edi
and %esi
registers, respectively.
Now, let's see how we can call this assembly function from Rust.
Calling Assembly Functions from Rust
First, we need to compile the assembly code into an object file. This can be done using an assembler like as
or nasm
. For our example, we'll use as
:
as -o add_numbers.o add_numbers.s
Next, we need to create a Rust extern
block that declares the assembly function. This tells Rust that the function is written in another language and will be linked during compilation:
// main.rs extern "C" { fn add_numbers(a: i32, b: i32) -> i32; }
Now, we can call the add_numbers
function just like any other Rust function:
fn main() { let a = 10; let b = 20; let result = unsafe { add_numbers(a, b) }; println!("Result: {}", result); }
Note that we have to use an unsafe
block to call the assembly function, as Rust can't guarantee any safety checks on foreign functions.
Finally, we need to compile and link the Rust and assembly code together. Here's how you can do that with rustc
and the gcc
linker:
rustc -C linker=gcc -o my_program main.rs add_numbers.o
Now, when you run my_program
, it will call the assembly function add_numbers
and print the result.
Conclusion
Using Rust's FFI to call assembly functions can be a powerful way to optimize your code and leverage low-level operations. Always remember that calling foreign functions requires unsafe
blocks, so be cautious and ensure the safety of your code. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is FFI in Rust and why would I want to use it?
FFI, or Foreign Function Interface, is a feature in Rust that allows you to call functions written in other programming languages, such as Assembly. This can be useful when you need to leverage low-level code or performance-critical functions that are better suited for languages like Assembly.
How do I declare an external function in Rust?
To declare an external function in Rust, you need to use the extern
keyword, followed by the desired ABI (application binary interface) in double quotes, and then a block containing the function signature. For example, to declare an external Assembly function named my_function
that takes two 32-bit integers and returns a 64-bit integer, you would write:
extern "C" { fn my_function(a: i32, b: i32) -> i64; }
What is an ABI and why is it important when using FFI in Rust?
An ABI (Application Binary Interface) is a set of conventions that dictate how functions should be called and data should be passed between different programming languages. When using FFI in Rust, it's crucial to specify the correct ABI to ensure that Rust can properly communicate with the external functions. The most commonly used ABI is the C ABI, denoted by "C"
.
How do I write an Assembly function to be called from Rust?
Writing an Assembly function to be called from Rust involves a few steps. First, you need to ensure that your Assembly code follows the desired ABI (such as the C ABI) for function signatures and data handling. Next, you need to assemble and link your Assembly code into a shared library (e.g., a .so
file on Linux or a .dll
file on Windows). Finally, you need to tell Rust to link with the shared library by using the #[link(name = "library_name")]
attribute. Here's an example of an Assembly function written in NASM that can be called from Rust:
; my_function.asm global my_function section .text my_function: ; Assembly code for the function ret
And the corresponding Rust code:
// main.rs #[link(name = "my_function")] extern "C" { fn my_function(a: i32, b: i32) -> i64; } fn main() { let result = unsafe { my_function(1, 2) }; println!("Result: {}", result); }
Are there any safety concerns when using FFI in Rust?
Yes, there are safety concerns when using FFI in Rust, primarily because you're interfacing with code that might not adhere to Rust's safety guarantees. To mitigate potential risks, Rust requires you to call FFI functions within an unsafe
block. This explicitly signals that you're aware of the potential dangers and have taken the necessary precautions. It's crucial to thoroughly review any external code you interface with and ensure it's safe to use with Rust.