Assembly Language Primer
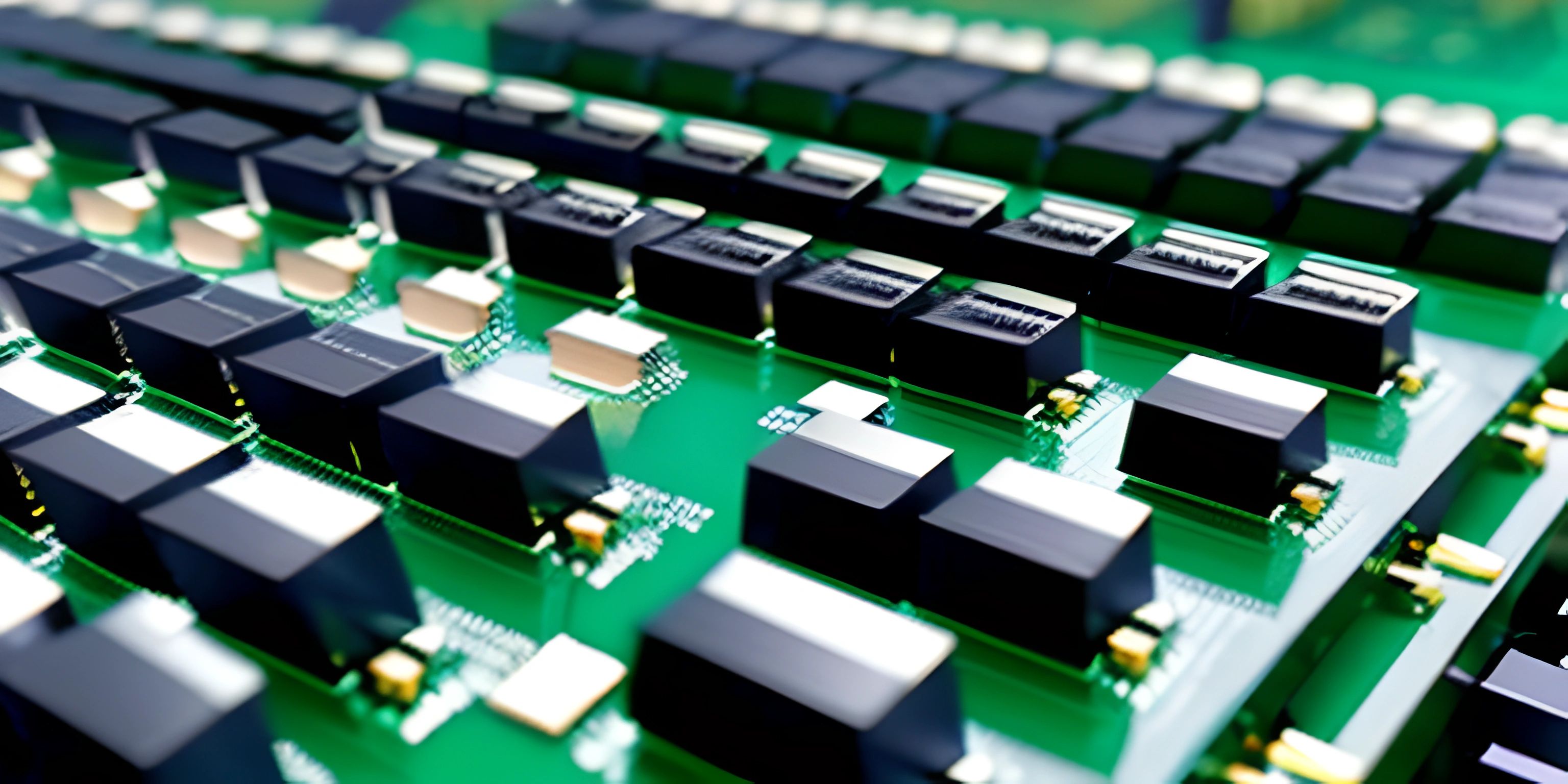
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Before diving into high-level programming languages like Python, JavaScript, or Ruby, it's important to understand the foundation they're built on. At the very core of computer programming lies assembly language, a low-level programming language that serves as a bridge between machine-level instructions and the more familiar syntax of high-level languages.
The Role of Assembly Language
Assembly language is a low-level programming language that provides a way for programmers to write code that is directly understood by the computer hardware. Unlike high-level languages, assembly language is platform-specific and closely tied to the underlying hardware. It allows programmers to manipulate the system's registers and memory, providing fine-grained control over how the computer operates.
From Assembly to Machine Code
When you write in assembly language, you're creating a set of instructions that are translated into machine code. Machine code is the lowest-level representation of code, consisting of binary instructions that the computer's hardware can directly execute. The process of converting assembly language into machine code is called assembling.
Here's a simple example of assembly code that adds two numbers and stores the result in a register:
MOV AX, 2 ADD AX, 3
These assembly instructions would be translated into machine code by an assembler, allowing the computer hardware to understand and execute them.
Why Assembly Language Matters
You might be wondering why we bother with assembly language in the first place, especially when we have more user-friendly, high-level languages available. Here are a few reasons why assembly language is still important:
Performance
Because assembly language is directly tied to the hardware it's running on, it can be optimized for performance. This means that assembly code can be written in a way that takes advantage of the specific features of the hardware, allowing for faster execution and better use of resources.
Understanding Hardware
Learning assembly language provides a deeper understanding of how computer hardware works, as it exposes you to the low-level details of how instructions are executed. This knowledge can be valuable when debugging, optimizing, or reverse-engineering code.
Embedded Systems
In embedded systems, where resources are limited, assembly language is often used to write code that is efficient, compact, and fast. High-level languages may be too resource-intensive for these situations.
Learning Assembly Language
If you're interested in learning assembly language, there are plenty of resources available, including tutorials, textbooks, and online courses. It's important to remember that assembly language is platform-specific, so choose a processor architecture (e.g., x86, ARM, MIPS) that aligns with your goals and interests.
While assembly language might seem daunting at first, remember that it's just another way to communicate with the computer. Once you start exploring and understanding the low-level world of assembly language, you'll gain valuable insights into the inner workings of computer hardware and appreciate the convenience and power of high-level languages even more.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is assembly language and why is it important?
Assembly language is a low-level programming language that is used to program a computer's central processing unit (CPU) directly. It is important because it provides a way for programmers to write instructions that can be executed directly by the hardware, allowing them to have a greater degree of control over the performance and behavior of a computer system. This level of control is especially useful in situations where high performance or precise timing is required.
How does assembly language differ from high-level programming languages?
Assembly language is a low-level programming language, which means it is much closer to the machine code that a computer's hardware can execute directly. High-level programming languages, on the other hand, are designed to be more human-readable and abstract away many of the complexities of working with hardware directly. This means that assembly language code is typically more difficult to read, write, and maintain than code written in high-level languages. However, assembly language allows for greater control over the hardware and can lead to more efficient and optimized code in certain situations.
Can I write programs entirely in assembly language?
Yes, you can write entire programs in assembly language, but it is not common practice for most modern software development. Writing programs entirely in assembly language can be time-consuming, error-prone, and difficult to maintain. However, there are still some cases where it might be beneficial to write portions of a program in assembly language, such as when optimizing critical sections of code for performance or working with hardware directly in embedded systems.
How does assembly language interact with hardware?
Assembly language is designed to work directly with a computer's hardware, specifically its CPU. Each assembly language instruction corresponds to one or more machine code instructions that the CPU can execute. When writing assembly language code, you are essentially writing a series of instructions that tell the CPU exactly what to do at the hardware level, such as moving data between memory locations, performing arithmetic operations, or controlling the flow of the program.
What tools do I need to get started with assembly language programming?
To get started with assembly language programming, you'll need a few essential tools:
- An assembler, which is a program that translates your assembly language code into machine code that the CPU can execute.
- A text editor for writing your assembly language code.
- A debugger, which will help you identify and fix errors in your code.
- Optionally, an integrated development environment (IDE) that combines these tools and provides a more user-friendly interface for writing, assembling, and debugging your assembly language code.