x86 NASM Array Operations
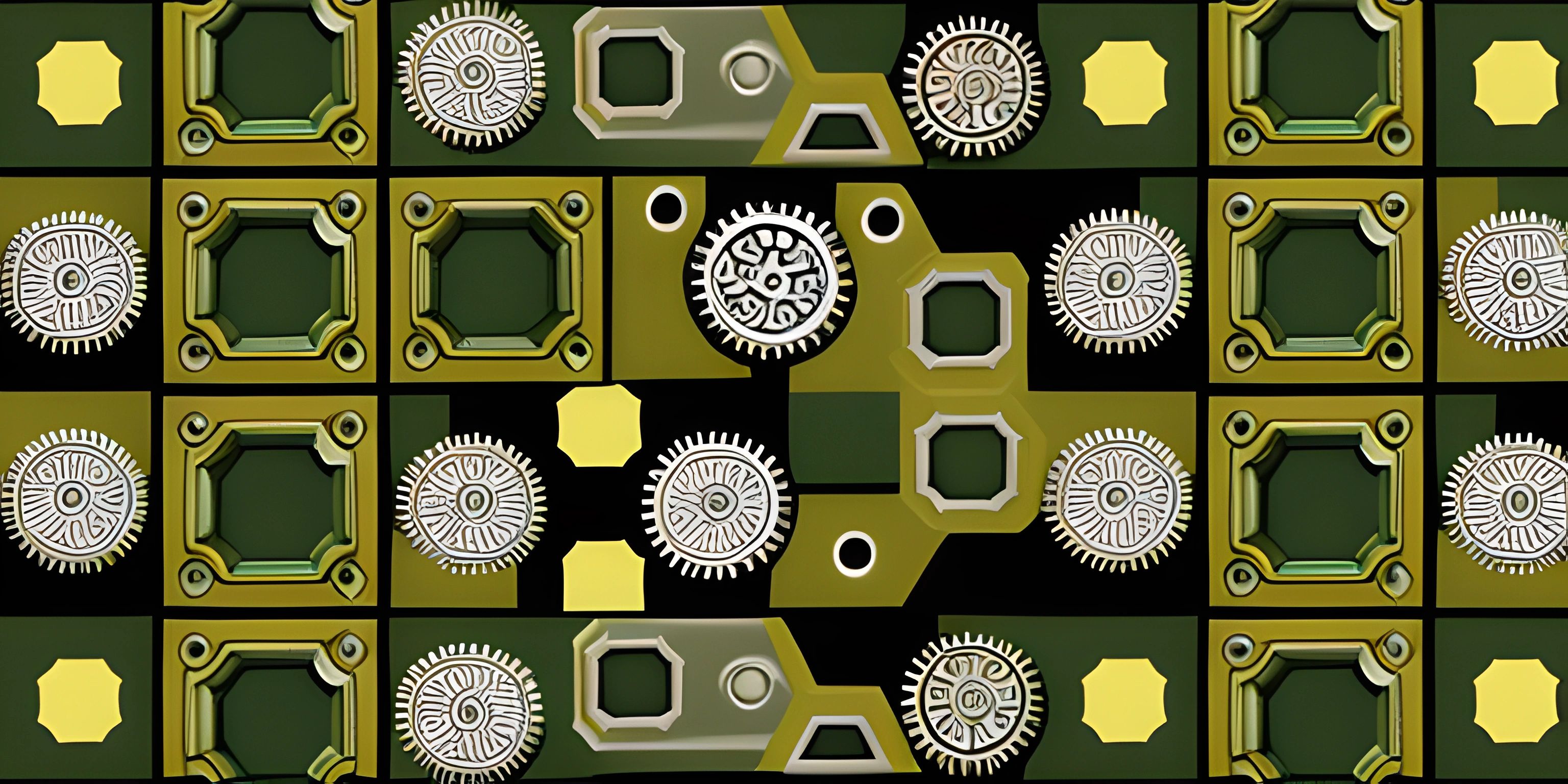
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When programming in x86 NASM assembly language, you'll often find yourself working with arrays. Arrays are vital to many algorithms and data structures, and mastering array operations is essential for efficient programming. In this article, we'll dive into the basics of arrays in x86 NASM and explore common array operations such as accessing, initializing, and modifying array elements.
Arrays in x86 NASM
An array is a contiguous block of memory that holds a fixed number of elements of the same data type. In x86 NASM, arrays can be declared using the times
directive followed by the data type and the initial values. Here's an example of declaring an array of 5 integers:
section .data array_example dd 1, 2, 3, 4, 5
This creates an array named array_example
, containing the integers 1, 2, 3, 4, and 5. The dd
directive is used to declare double-word (32-bit) integers.
Accessing Array Elements
To access an element in an array, you need to calculate its memory address. The address of the i-th element can be calculated using the formula: base_address + (index * element_size)
.
For example, to access the second element (index 1) of our array_example
array, we would calculate the address as follows:
mov eax, [array_example + 1 * 4]
In this case, we're using the mov
instruction to load the value of the second element into the eax
register. We multiply the index (1) by the size of each element (4 bytes), and add the result to the base address of the array.
Initializing Arrays
Sometimes, you may want to initialize an array with a specific value. The times
directive comes in handy for this purpose. Here's an example of initializing an array of 10 integers, all set to the value 42:
section .data array_init_example times 10 dd 42
Modifying Array Elements
To modify an array element, you need to write the new value to its memory address. Let's say we want to change the third element (index 2) of our array_example
array to the value 99:
mov dword [array_example + 2 * 4], 99
In this case, we're using the mov
instruction to store the value 99 at the memory address of the third element.
Looping Through an Array
One common operation is to iterate through an array, performing some action on each element. To do this, you can use a loop that increments an index variable and calculates the address of each element. Here's an example of a loop that adds 10 to each element in our array_example
array:
section .data array_example dd 1, 2, 3, 4, 5 array_length equ 5 section .text add_ten_to_array: mov ecx, 0 ; Initialize index variable (ecx) to 0 loop_start: cmp ecx, array_length jge loop_end ; If index >= array_length, jump to loop_end mov eax, [array_example + ecx * 4] ; Load array element into eax add eax, 10 ; Add 10 to the element mov [array_example + ecx * 4], eax ; Store the updated element back in the array inc ecx ; Increment index variable jmp loop_start ; Jump back to the beginning of the loop loop_end: ; ... continue with the rest of the program
In this code snippet, we use the ecx
register as our index variable and loop through the array using the cmp
, jge
, and jmp
instructions to control the flow.
Now you have a solid understanding of how to work with arrays in x86 NASM assembly language. With this knowledge, you can create more complex algorithms and data structures, unlocking the full potential of low-level programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Word Splitter (psst, it's free!).
FAQ
What are common array operations in x86 NASM assembly language?
Common array operations in x86 NASM assembly language include creating or initializing an array, accessing individual elements, modifying elements, searching for elements or values, and iterating through the array.
How do I create and initialize an array in x86 NASM assembly language?
To create and initialize an array in x86 NASM assembly language, you can use the db
, dw
, or dd
directive followed by the initial values of the array. Here is an example of creating an array of 4 bytes:
section .data myArray db 1, 2, 3, 4
How can I access an individual element in an x86 NASM array?
To access an individual element in an x86 NASM array, you can use the base address of the array and the appropriate index value. Here's an example of accessing the second element of a byte array:
section .data myArray db 1, 2, 3, 4 section .text global _start _start: ; Access the second element of the array mov eax, [myArray + 1]
How do I modify an element in an x86 NASM array?
To modify an element in an x86 NASM array, use the mov
instruction to update the value at the desired index. Here's an example of updating the third element of a byte array:
section .data myArray db 1, 2, 3, 4 section .text global _start _start: ; Update the third element of the array to 42 mov byte [myArray + 2], 42
How can I iterate through an x86 NASM array and perform operations on its elements?
To iterate through an x86 NASM array, you can use a loop with a counter variable, and increment the index or base address to access each element. Here's an example of iterating through a byte array and adding 10 to each element:
section .data myArray db 1, 2, 3, 4 arraySize equ 4 section .text global _start _start: ; Initialize loop counter mov ecx, 0 loop_start: ; Add 10 to the current element of the array add byte [myArray + ecx], 10 ; Increment loop counter inc ecx ; Check if we reached the end of the array cmp ecx, arraySize jl loop_start ; If not, continue looping