Understanding NASM Assembly: Basic Syntax and Commands
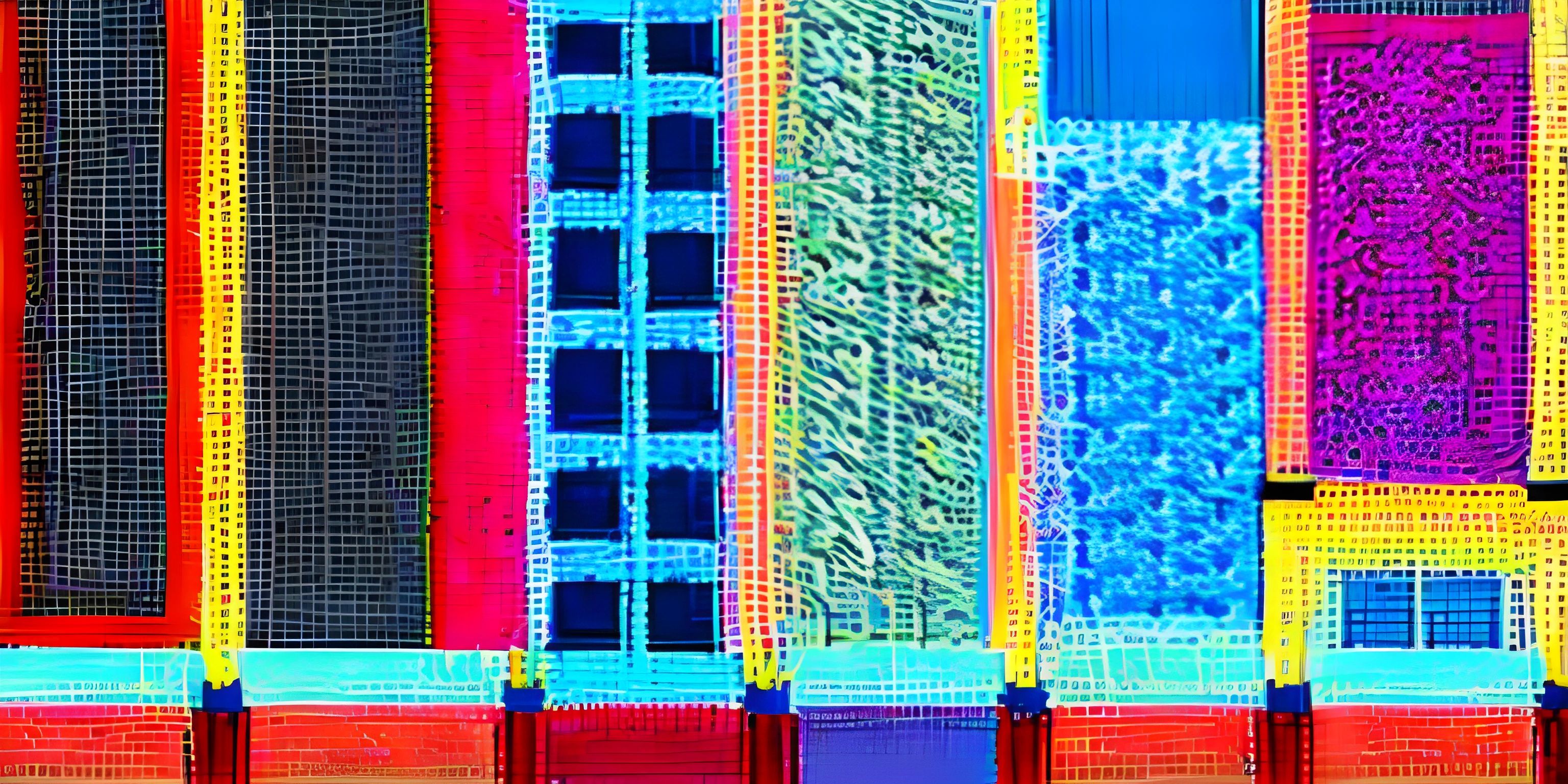
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome aboard the assembly express! Our destination today: NASM Assembly, specifically its basic syntax and commands. Buckle up and keep your hands inside the vehicle!
Syntax Overview
NASM, Netwide Assembler, is an assembler commonly used for x86 architecture. It reads source code written by programmers and converts it into machine language. Now, let's dive into the syntax of NASM Assembly!
Comments
Every journey starts with a single step, or in this case, a single line of code. Let's kick off our venture with comments. In NASM, comments are marked with a semicolon (;). Anything after a semicolon on a line is ignored, allowing us to jot down notes or explanations.
mov eax, 1 ; This is a comment. It does not affect the program.
Instructions
Instructions are the meat of any assembly program. They're the actions that you want the computer to carry out. An instruction in NASM typically has two parts: the opcode and the operands. The opcode is the command the computer will execute, and the operands are the data that the command will act on. Here's an example:
mov eax, 1 ; 'mov' is the opcode, 'eax' and '1' are the operands.
Registers
Registers in NASM are like little storage units. These are the places in computer memory where data is stored for immediate use. For example, eax
is a register, and mov eax, 1
puts the value 1 into that register.
mov eax, 1 ; This line moves 1 into the eax register.
Basic Commands
Now that we've mastered the basic syntax, it's time to introduce some basic commands. In NASM, there are numerous commands to perform different operations.
mov
The mov
command is used to move data from one place to another. For instance, moving a value to a register.
mov eax, 1 ; This moves 1 into the eax register.
add
The add
command is used to add two values together.
add eax, 2 ; This adds 2 to whatever value is currently in the eax register.
sub
Sub
, as you might expect, subtracts one value from another.
sub eax, 1 ; This subtracts 1 from whatever value is currently in the eax register.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is NASM Assembly?
NASM, or Netwide Assembler, is an assembler used for x86 architecture. It reads source code written by programmers and converts it into machine language, which can be executed by a computer.
How can I write a comment in NASM Assembly?
Comments in NASM Assembly are written by placing a semicolon (;) at the start of the comment. Anything after the semicolon on the same line is treated as a comment and ignored by the compiler.
What are some basic commands in NASM Assembly?
Some basic commands in NASM Assembly include mov
to move data, add
to add values, and sub
to subtract values.
What are registers in NASM Assembly?
Registers in NASM Assembly are like storage units in the computer's memory where data is stored for immediate use. For instance, eax
is a register, and the command mov eax, 1
would move the value 1 into the eax
register.
What is the structure of an instruction in NASM Assembly?
An instruction in NASM Assembly typically has two parts: the opcode and the operands. The opcode is the command for the computer to execute, and the operands are the data or values that the command will act on.