x86 Assembly NASM Stack
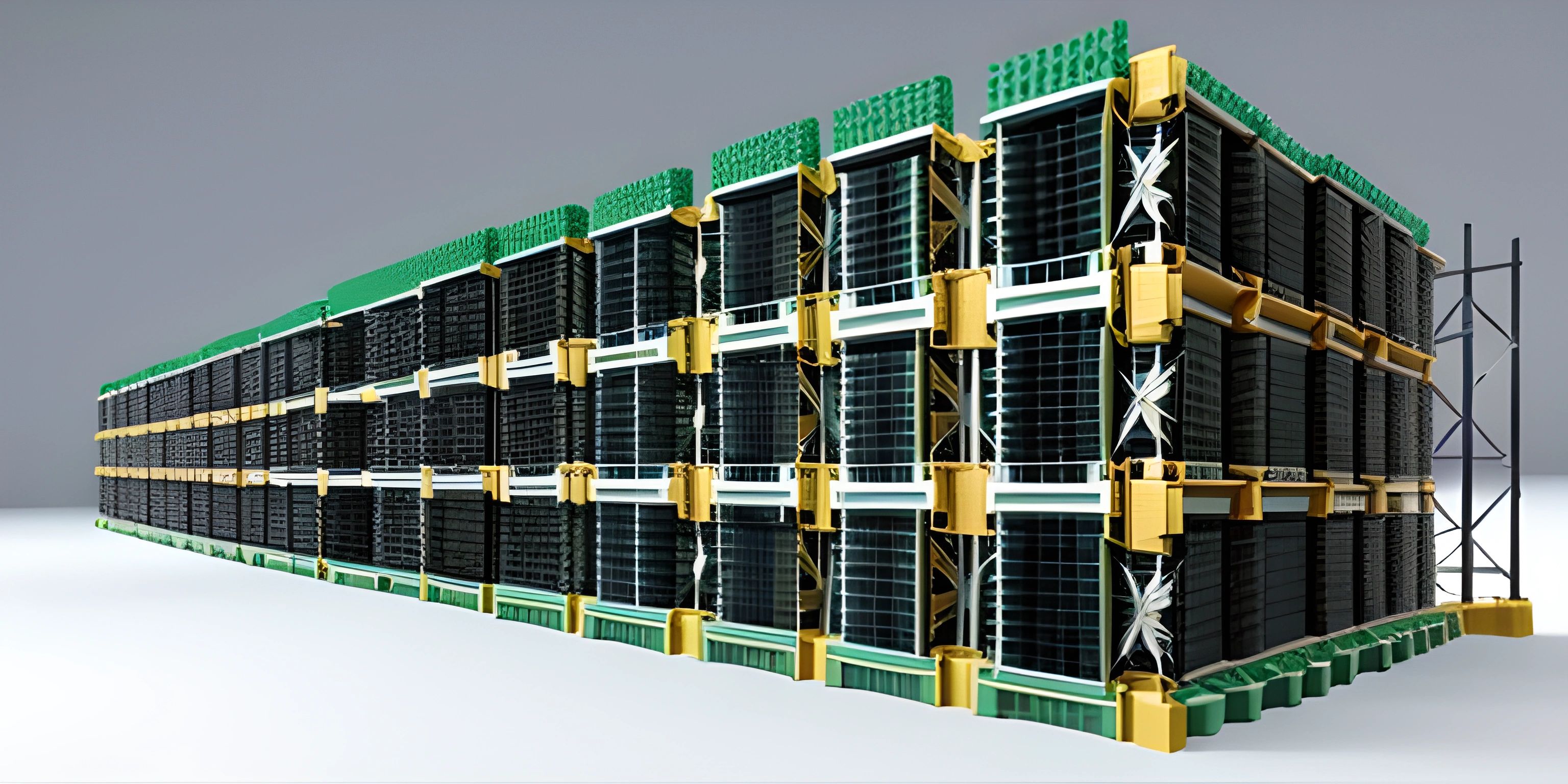
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Manipulating the stack in x86 Assembly language using NASM is a fundamental skill for any budding Assembly programmer. In this article, we'll explore how to use the stack for function parameters and local variables, making your Assembly programs more modular and easier to maintain.
The Stack
In x86 Assembly, the stack is a region of memory used to store temporary data, such as function parameters and local variables. The stack grows downwards in memory, meaning that as you push data onto the stack, the stack pointer (ESP) decreases.
Push and Pop
To manipulate the stack, you'll mainly use two key instructions: push
and pop
.
push
: Decreases the stack pointer by the size of the data being pushed (usually 4 bytes for a 32-bit value) and stores the data at the new location pointed by ESP.pop
: Retrieves the value at the current ESP location and stores it in the specified register, then increases the ESP by the size of the data being popped (usually 4 bytes for a 32-bit value).
Here's an example:
push eax ; Push the value of eax onto the stack pop ebx ; Pop the value from the stack into ebx
Function Parameters and the Stack
When calling a function, you can pass parameters to it by pushing them onto the stack. The called function will then use the stack to access these parameters.
Let's say we have a simple function sum
that takes two parameters, a
and b
, and returns their sum in the eax
register:
; Function: sum ; Parameters: a (4 bytes), b (4 bytes) ; Returns: eax = a + b sum: push ebp ; Save the old base pointer mov ebp, esp ; Set the new base pointer mov eax, [ebp+8] ; Load a from the stack add eax, [ebp+12] ; Add b to eax pop ebp ; Restore the old base pointer ret 8 ; Return and clean up 8 bytes of parameters from the stack
To call this function, you'd push the parameters onto the stack in reverse order:
push 3 ; Push b onto the stack push 2 ; Push a onto the stack call sum ; Call the sum function
After the call
instruction, the value 5 (2 + 3) will be in the eax
register.
Local Variables and the Stack
You can also use the stack to store local variables within a function. To do this, you'll need to adjust the stack pointer (ESP) to make room for your local variables. Here's an example:
; Function: local_example ; Parameters: None ; Returns: eax = 42 local_example: push ebp ; Save the old base pointer mov ebp, esp ; Set the new base pointer sub esp, 4 ; Make room for a 4-byte local variable mov [ebp-4], 42 ; Store 42 in the local variable mov eax, [ebp-4] ; Load the local variable into eax mov esp, ebp ; Clean up local variables pop ebp ; Restore the old base pointer ret ; Return
In this example, we create a local variable by subtracting 4 from ESP, store the value 42 in that local variable, and then load it into the eax
register before returning.
Now you're well-equipped to handle function parameters and local variables using the stack in x86 Assembly language with NASM. By mastering these concepts, you'll be able to write more modular and maintainable Assembly programs. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the stack in x86 Assembly language?
The stack is a region in memory used by x86 Assembly language programs for storing temporary data, function parameters, and local variables. It grows downward from higher addresses to lower addresses and operates on a Last-In, First-Out (LIFO) principle. This means that the most recently added item is the first one to be removed when needed.
How do I push and pop values to/from the stack in NASM?
In NASM, you can use the push
and pop
instructions to add and remove values from the stack, respectively. Here's a simple example:
push eax ; Push the value in the eax register onto the stack ; Perform some operations here pop eax ; Pop the topmost value from the stack back into the eax register
How do I pass function parameters using the stack?
To pass function parameters using the stack, you can push the values onto the stack before calling the function. The called function can then access these values using the base pointer (ebp) register. Here's an example:
; Caller function push param2 ; Push the second parameter push param1 ; Push the first parameter call some_function ; some_function some_function: push ebp ; Save the previous base pointer mov ebp, esp ; Set the current base pointer ; Now you can access the parameters using [ebp + offset] addressing ; [ebp + 8] refers to param1 and [ebp + 12] refers to param2
How to allocate and use local variables with the stack in NASM?
To allocate space for local variables in NASM, you can use the sub
instruction to adjust the stack pointer (esp) register. To access these variables, you can use the base pointer (ebp) register. Here's an example:
function_with_local_vars: push ebp ; Save the previous base pointer mov ebp, esp ; Set the current base pointer sub esp, 8 ; Allocate space for two 4-byte local variables ; ... ; Access local variables using [ebp - offset] addressing ; [ebp - 4] refers to the first local variable and [ebp - 8] refers to the second local variable
How can I clean up the stack after a function call in NASM?
After a function call, it's essential to clean up the stack by popping off any pushed parameters and restoring the previous stack pointer. Here's an example of how to do this:
; Caller function push param1 push param2 call some_function add esp, 8 ; Clean up the stack by restoring the esp register (assuming two 4-byte parameters) ; some_function some_function: push ebp mov ebp, esp ; Function body pop ebp ; Restore the previous base pointer ret ; Return to the caller function