Using CSS Modules for Styling Next.js Components
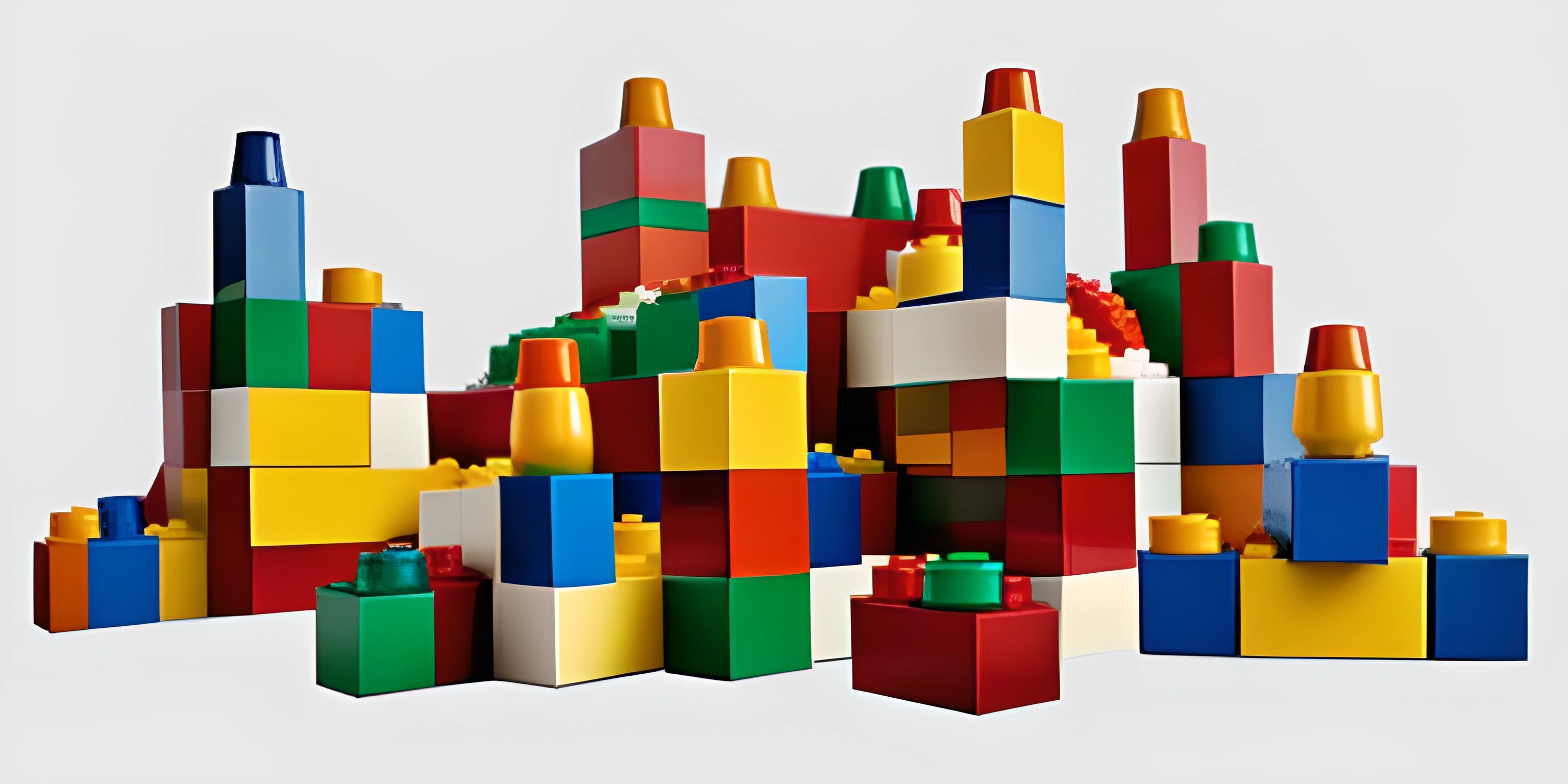
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Next.js is a popular framework for building React applications, and one of its powerful features is the built-in support for CSS Modules. So, what are CSS Modules? CSS Modules are a technique for making the CSS styles scoped to a specific component, preventing unwanted side effects and clashes. In this guide, we'll learn how to work with CSS Modules and style components in a Next.js application.
Setting up Next.js
Before diving into CSS Modules, you'll need a Next.js project. If you don't have one already, create a new one using create-next-app with the following command:
npx create-next-app your-project-name
Creating a CSS Module
To create a CSS Module, simply create a new .module.css
file in your project. The naming convention is important, as it tells Next.js that this file is a CSS Module. For example, let's create a Button.module.css
file:
/* Button.module.css */ .button { background-color: blue; color: white; padding: 8px 16px; border: none; border-radius: 4px; cursor: pointer; }
Using a CSS Module in a Component
Now that we have a CSS Module, let's use it in a Next.js component. Create a new file called Button.js
:
// Button.js import React from "react"; import styles from "./Button.module.css"; const Button = ({ children, onClick }) => { return ( <button className={styles.button} onClick={onClick}> {children} </button> ); }; export default Button;
Here, we import the CSS Module using the styles
variable. The styles are automatically scoped to the component, so there's no need to worry about naming clashes or unintended side effects.
Global Styles
CSS Modules are great for component-specific styling, but sometimes you need global styles that apply to your entire application. To achieve this, create a file called _app.js
in the pages
folder:
// pages/_app.js import "../styles/global.css"; import { AppProps } from "next/app"; function MyApp({ Component, pageProps }: AppProps) { return <Component {...pageProps} />; } export default MyApp;
Now, any styles defined in the global.css
file will be applied globally. Note that this file should not be a CSS Module.
That's it! You're now ready to use CSS Modules in your Next.js application. This technique will help you to write clean, maintainable, and conflict-free styles. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What are CSS Modules and why should I use them in Next.js?
CSS Modules are a popular styling approach that allows you to define CSS styles in a modular way. They help you avoid global style conflicts by generating unique class names for each style, automatically scoping them to their respective components. In Next.js, using CSS Modules is a great way to ensure consistent styling and avoid unintended side-effects from cascading styles.
How do I create a CSS Module in a Next.js project?
To create a CSS Module in your Next.js project, follow these steps:
- Create a new CSS file with the extension
.module.css
in the same folder as your component (e.g.,MyComponent.module.css
). - Write your CSS styles within the file as you normally would.
- In your React component, import the CSS Module by adding the following line:
import styles from "./MyComponent.module.css";
- Apply the imported styles to your JSX elements using the
className
attribute, like so:<div className={styles.myStyle}>Hello, world!</div>
.
Can I use CSS Modules alongside other styling methods in Next.js?
Yes, you can use CSS Modules alongside other styling methods in your Next.js project. For instance, you can combine them with global stylesheets, styled-jsx, or other CSS-in-JS libraries. However, it's essential to maintain a consistent and organized styling approach throughout your project to ensure maintainability and ease of collaboration.
How can I customize the generated class names in CSS Modules?
By default, Next.js generates unique class names for your CSS Modules. However, you can customize the format of these class names by modifying the next.config.js
configuration file in your project:
module.exports = { webpack: (config) => { config.module.rules.push({ test: /\.module\.css$/, use: [ { loader: "css-loader", options: { modules: { localIdentName: "[local]__[hash:base64:5]", }, }, }, ], }); return config; }, };
In the example above, the localIdentName
option is set to [local]__[hash:base64:5]
, which will generate class names in the format myStyle__abcde
, where myStyle
is the original class name and abcde
is a unique hash.
Is it possible to use CSS Modules with SASS or other CSS preprocessors in Next.js?
Yes, you can use CSS Modules with SASS or other CSS preprocessors in Next.js by installing the relevant dependencies and configuring your project accordingly. For instance, to use SASS with CSS Modules, you need to install sass
and follow these steps:
- Create a new SASS file with the extension
.module.scss
or.module.sass
(e.g.,MyComponent.module.scss
). - Write your SASS code within the file.
- In your React component, import the SASS Module like this:
import styles from "./MyComponent.module.scss";
- Apply the imported styles to your JSX elements using the
className
attribute, just like you would with a regular CSS Module.