Styling React Components
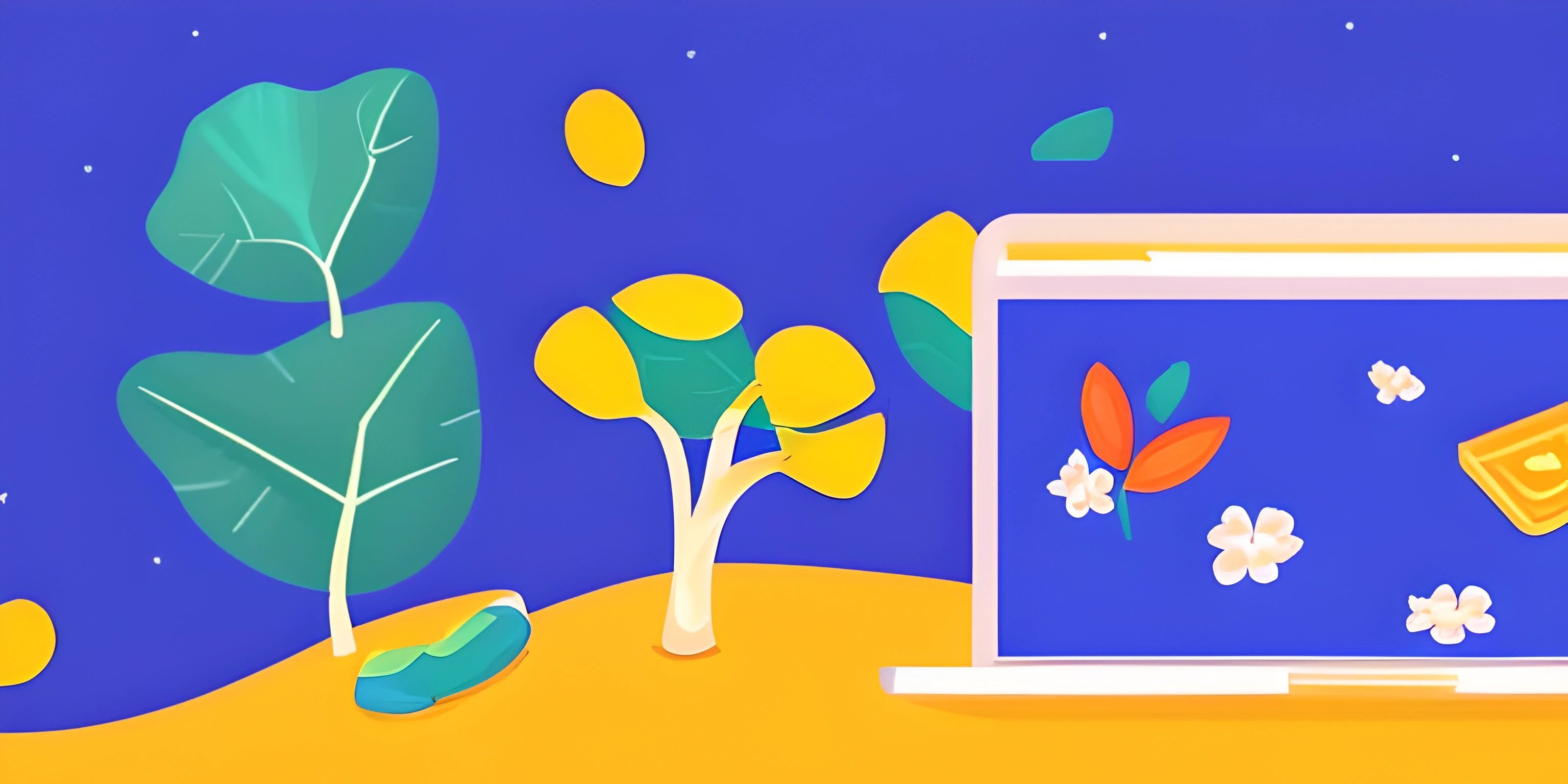
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React is fantastic for building reusable and efficient UI components, but you may wonder how to make these components look good. With numerous options at your disposal, it's essential to know the best practices for styling in React. Fear not, by the end of this article, you'll be a React styling wizard!
Inline Styling
A simple way to style your React components is using inline styling. Inline styling allows you to apply CSS directly to an element using the style
attribute. In React, you'll provide an object containing the CSS properties and their values. Let's start with a basic example:
function MyComponent() { const style = { color: "blue", fontSize: "18px", backgroundColor: "lightgray" }; return ( <div style={style}> This is a styled React component! </div> ); }
While inline styling is convenient for small projects, it's not the most scalable option. As your project grows, you might run into maintainability issues, making it harder to manage styles across your app.
CSS Modules
CSS Modules offer a more structured approach to styling. They enable you to create local, scoped CSS classes for individual components. Create a .module.css
file next to your React component, and then import the styles.
Suppose we have a Button.module.css
file:
.button { background-color: blue; color: white; padding: 10px 20px; border-radius: 5px; cursor: pointer; }
And a Button.jsx
file:
import React from 'react'; import styles from './Button.module.css'; function Button() { return ( <button className={styles.button}> Click me! </button> ); } export default Button;
This approach gives you the full power of CSS, while maintaining scoping and modularity.
Styled-components and Emotion
Now, let's dive into two popular CSS-in-JS libraries: styled-components and Emotion. Both libraries allow you to create styled components using JavaScript.
You'll need to install the libraries first:
npm install styled-components npm install @emotion/react @emotion/styled
Here's how to create a styled button using styled-components:
import React from 'react'; import styled from 'styled-components'; const StyledButton = styled.button` background-color: blue; color: white; padding: 10px 20px; border-radius: 5px; cursor: pointer; `; function Button() { return ( <StyledButton> Click me! </StyledButton> ); } export default Button;
And here's the Emotion equivalent:
import React from 'react'; import styled from '@emotion/styled'; const StyledButton = styled.button` background-color: blue; color: white; padding: 10px 20px; border-radius: 5px; cursor: pointer; `; function Button() { return ( <StyledButton> Click me! </StyledButton> ); } export default Button;
Both libraries allow you to write CSS within your JavaScript, making it easy to manage and maintain styles alongside your components.
Best Practices
To ensure a smooth styling experience in your React project, follow these best practices:
- Pick a consistent styling approach – Choose one method (inline, CSS Modules, styled-components, Emotion, etc.) and stick to it throughout your project.
- Use a naming convention – To maintain consistency, adopt a naming convention like BEM for your CSS classes.
- Keep component styles together – Keep the styles for each component within the same folder to improve maintainability and organization.
- Leverage reusable components – Create reusable styled components for common elements like buttons or form inputs.
Now you're equipped with the knowledge to style your React components like a pro! Choose the method that works best for you and your project, and remember to always follow best practices. Happy styling!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What are some common ways to style React components?
In React, there are several ways to style components:
- Inline styles
- CSS stylesheets
- CSS modules
- Styled-components
- Emotion Choose the method that best suits your project's requirements and your own personal preferences.
How do I use inline styles in React components?
To use inline styles in React components, define your styles as a JavaScript object and pass it to the style
attribute of the element. Make sure to use camelCase for property names:
const myStyles = { color: "red", fontSize: "24px" }; function MyComponent() { return <div style={myStyles}>Hello, World!</div>; }
How can I use CSS stylesheets with React components?
You can use traditional CSS stylesheets with React components by creating a separate .css
file and importing it into your component:
// App.css .container { background-color: blue; padding: 20px; } // App.js import React from 'react'; import './App.css'; function App() { return <div className="container">Welcome to my App!</div>; }
What are CSS modules and how do I use them in React?
CSS modules are a technique that allows you to scope CSS to a specific component, avoiding global scope and naming conflicts. To use CSS modules, create a .module.css
file and import it as an object in your component:
// Button.module.css .button { background-color: green; padding: 10px; } // Button.js import React from 'react'; import styles from './Button.module.css'; function Button() { return <button className={styles.button}>Click me!</button>; }
How do I use styled-components in my React project?
Styled-components is a popular CSS-in-JS library that allows you to write component-scoped CSS directly in your JavaScript code. First, install the library (npm install styled-components
), then import it and create styled components:
import React from 'react'; import styled from 'styled-components'; const StyledButton = styled.button` background-color: purple; padding: 10px; color: white; `; function App() { return <StyledButton>Click me!</StyledButton>; }