Node.js Console Log Examples
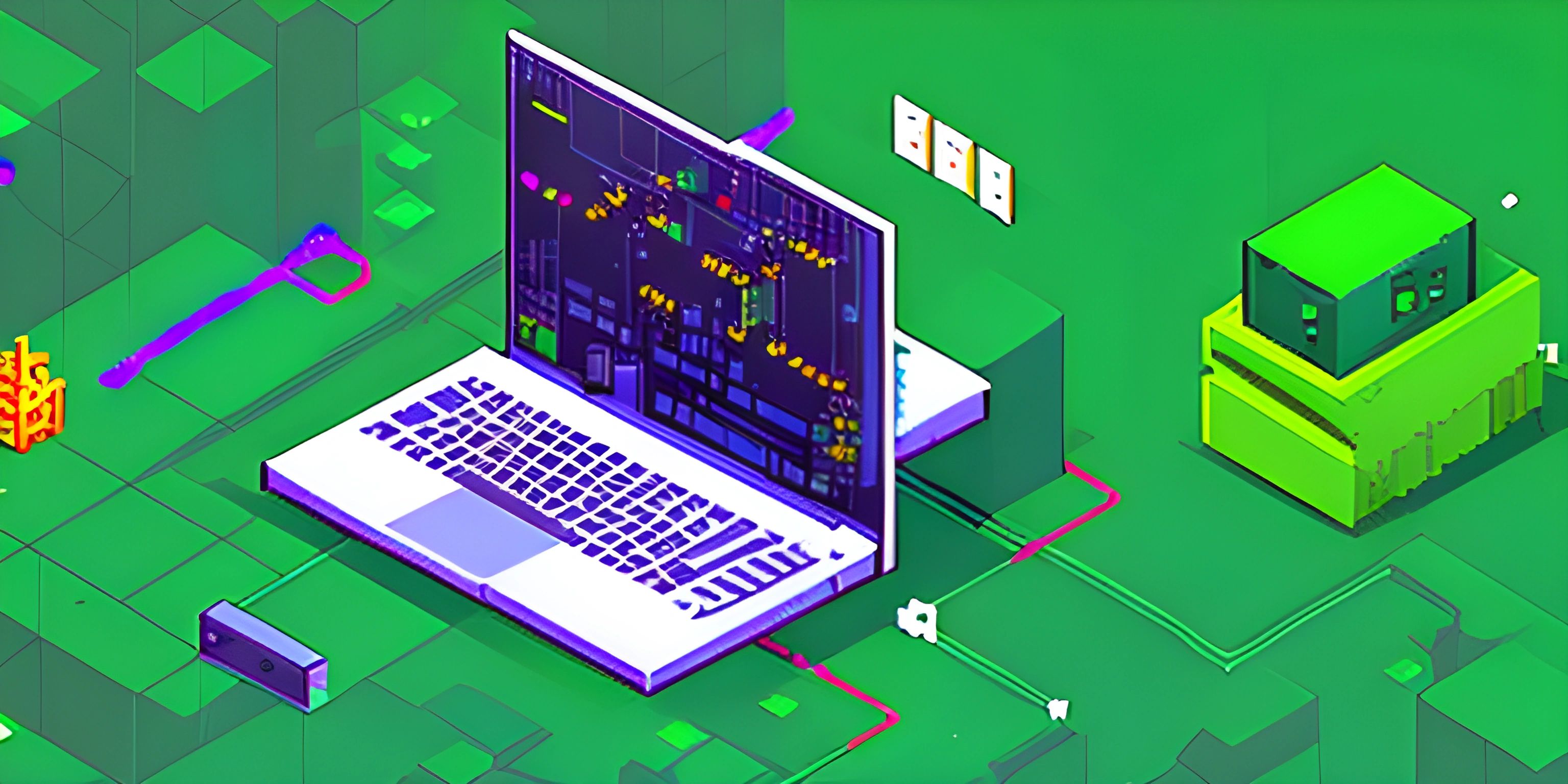
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ah, the humble console.log()
. It's the first thing most of us encounter when we dive into the world of JavaScript. Whether you're trying to debug an issue, display some information, or just want to check if your code is even running, console.log()
has got your back. In this article, we’ll explore various ways to use console.log()
in a Node.js environment and make your debugging life a whole lot easier.
Basic Usage of console.log()
Starting off with the basics, the simplest form of console.log()
is to directly print strings or numbers to the console.
console.log("Hello, Node.js!"); console.log(42);
Running this code in a Node.js environment will produce the following output:
Hello, Node.js! 42
Concatenating Strings and Variables
Just like in the browser, you can concatenate strings and variables. This is useful when you want to display dynamic content.
let userName = "Alice"; console.log("Welcome, " + userName + "!");
Output:
Welcome, Alice!
Notice how we used the +
operator to combine the string "Welcome, " with the value of userName
and the string "!".
Using Template Literals
If you want a cleaner way to include variables in your strings, JavaScript's template literals are your friend. This makes the code more readable and avoids the messy concatenation.
let userName = "Bob"; console.log(`Welcome, ${userName}!`);
Output:
Welcome, Bob!
Logging Objects and Arrays
In Node.js, you can also log objects and arrays.
let user = { name: "Charlie", age: 30 }; let hobbies = ["reading", "gaming", "coding"]; console.log(user); console.log(hobbies);
Output:
{ name: 'Charlie', age: 30 } [ 'reading', 'gaming', 'coding' ]
Formatting Output
For more advanced logging, console.log()
supports formatting specifiers like %s
for strings, %d
for numbers, and %j
for JSON.
let userName = "David"; let age = 25; console.log("User: %s, Age: %d", userName, age);
Output:
User: David, Age: 25
Using console.dir()
While console.log()
is great for most cases, sometimes you need to inspect objects more thoroughly. This is where console.dir()
comes in handy.
let user = { name: "Eve", age: 28, hobbies: ["painting", "hiking"] }; console.dir(user, { depth: null, colors: true });
Output (with colors if your terminal supports it):
{ name: 'Eve', age: 28, hobbies: [ 'painting', 'hiking' ] }
Logging with Different Levels
Node.js also supports different logging levels such as console.info()
, console.warn()
, and console.error()
to categorize your log messages.
console.info("This is an informational message."); console.warn("This is a warning message."); console.error("This is an error message.");
Output:
This is an informational message. This is a warning message. This is an error message.
Timing with console.time()
and console.timeEnd()
If you need to measure how long a piece of code takes to execute, you can use console.time()
and console.timeEnd()
.
console.time("loop"); for (let i = 0; i < 1000000; i++) { // Some intensive task } console.timeEnd("loop");
Output:
loop: 5.123ms
Counting with console.count()
To count the number of times a particular line of code is executed, use console.count()
.
for (let i = 0; i < 5; i++) { console.count("Loop executed"); }
Output:
Loop executed: 1 Loop executed: 2 Loop executed: 3 Loop executed: 4 Loop executed: 5
Grouping Logs
You can group multiple logs together using console.group()
and console.groupEnd()
.
console.group("User Details"); console.log("Name: Frank"); console.log("Age: 40"); console.log("Occupation: Engineer"); console.groupEnd();
Output:
User Details Name: Frank Age: 40 Occupation: Engineer
Customizing Console Output with chalk
For even more control over your console output, you can use the chalk
library to add colors and styles.
First, install the chalk
package:
npm install chalk
Then, use it in your code:
const chalk = require("chalk"); console.log(chalk.blue("Hello, world!")); console.log(chalk.red.bold("Error: Something went wrong!")); console.log(chalk.green("Success: Everything is working!"));
Output:
Hello, world! (in blue) Error: Something went wrong! (in bold red) Success: Everything is working! (in green)
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: File I/O (Reading) (psst, it's free!).
FAQ
How can I log multiple variables in a single `console.log()`?
You can log multiple variables by separating them with commas inside the console.log()
function. For example: console.log(var1, var2, var3)
.
Is there a way to format the output of `console.log()`?
Yes, you can use formatting specifiers like %s
, %d
, and %j
to format strings, numbers, and JSON respectively. For example: console.log("User: %s, Age: %d", userName, age)
.
How can I print colored text to the console in Node.js?
You can use the chalk
library to add colors and styles to your console output. First, install the library using npm install chalk
, then use it in your code to customize your logs.
What is the difference between `console.log()` and `console.dir()`?
console.log()
is generally used for logging simple messages, while console.dir()
is useful for inspecting objects in more detail. console.dir()
allows you to specify options like depth and colors.
How can I measure the execution time of a piece of code?
You can use console.time()
to start a timer and console.timeEnd()
to end the timer and log the elapsed time. For example:
console.time("test"); // some code console.timeEnd("test");