Using Console.log in Node.js
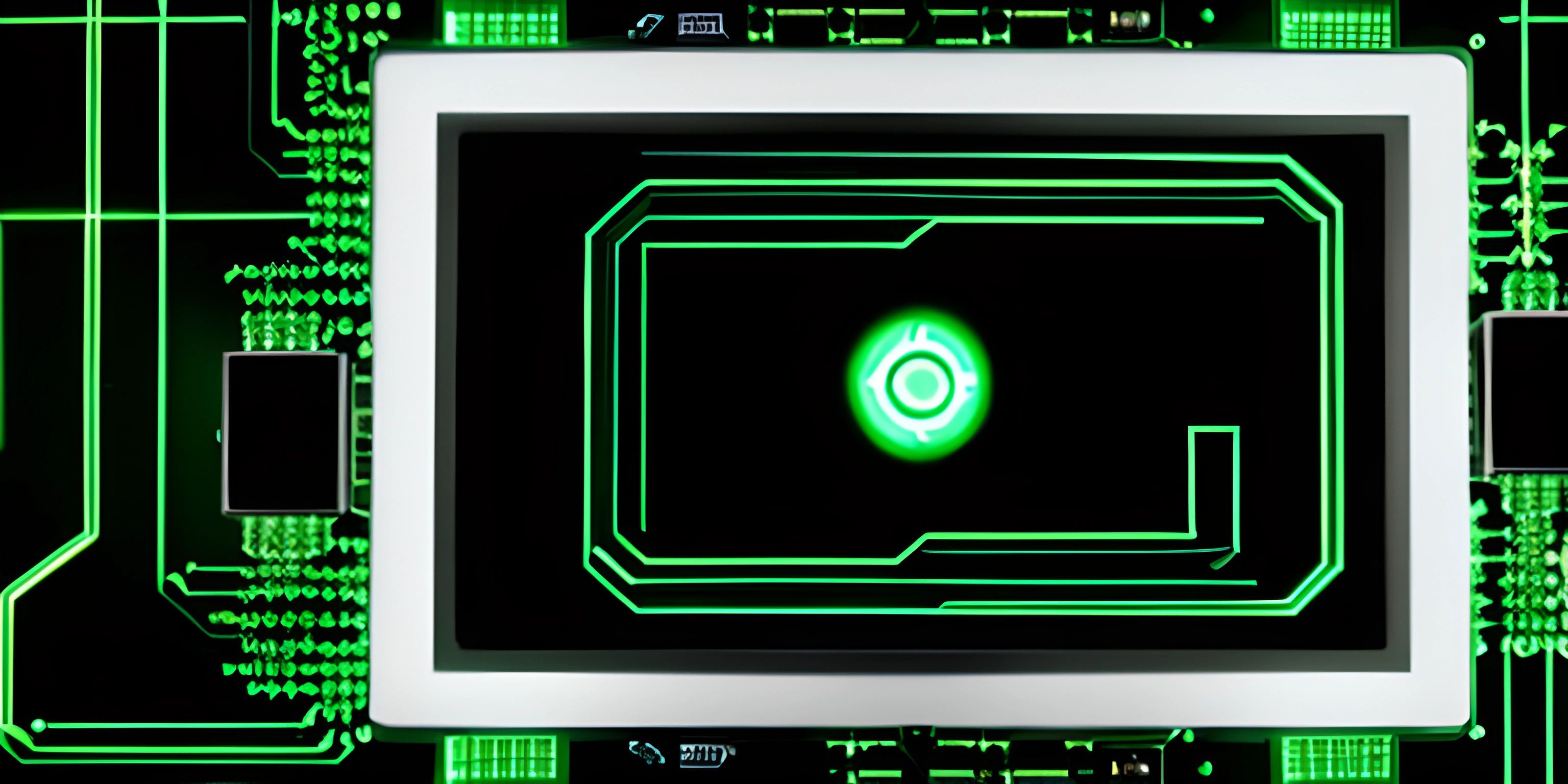
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In Node.js, the console.log()
method is commonly used to print values to the console for debugging purposes. This method can take multiple arguments and print them to the console as a space-separated string.
Syntax
The basic syntax for using console.log()
is as follows:
console.log(value1 [, value2, ..., valueN]);
Here, value1, value2, ..., valueN
are the values that you want to print to the console.
Example
Let's see an example of using console.log()
to print different types of values:
// Print a simple string console.log("Hello, Cratecode!"); // Print a number console.log(42); // Print an array console.log([1, 2, 3, 4, 5]); // Print an object console.log({name: "John", age: 30}); // Print multiple values console.log("Name:", "John", "Age:", 30);
Output
When you run the above code, you will see the following output in the console:
Hello, Cratecode! 42 [ 1, 2, 3, 4, 5 ] { name: 'John', age: 30 } Name: John Age: 30
Now you know how to use console.log()
to print values to the console in Node.js. This method can be very helpful for debugging your code and understanding the flow of your program.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is console.log() in Node.js?
console.log()
is a built-in method in Node.js that allows you to print values to the console. It is widely used for debugging purposes, as it helps you to visualize the data and track the flow of your application.
How do I use console.log() in my Node.js application?
To use console.log()
in your Node.js application, simply call the method and pass the value you want to print as an argument. For example:
console.log("Hello, World!"); console.log(42); console.log({"name": "Alice", "age": 30});
Can I print multiple values in one console.log() call?
Yes, you can print multiple values in one console.log()
call by passing multiple arguments separated by commas. For example:
console.log("Name:", "Alice", "Age:", 30);
This will print "Name: Alice Age: 30" in the console.
How to format console.log() output using template literals?
You can use template literals (backticks) and placeholders (${expression}
) to format the output of console.log()
. For example:
const name = "Alice"; const age = 30; console.log(`Name: ${name}, Age: ${age}`);
This will print "Name: Alice, Age: 30" in the console.