Node.js Readline Introduction
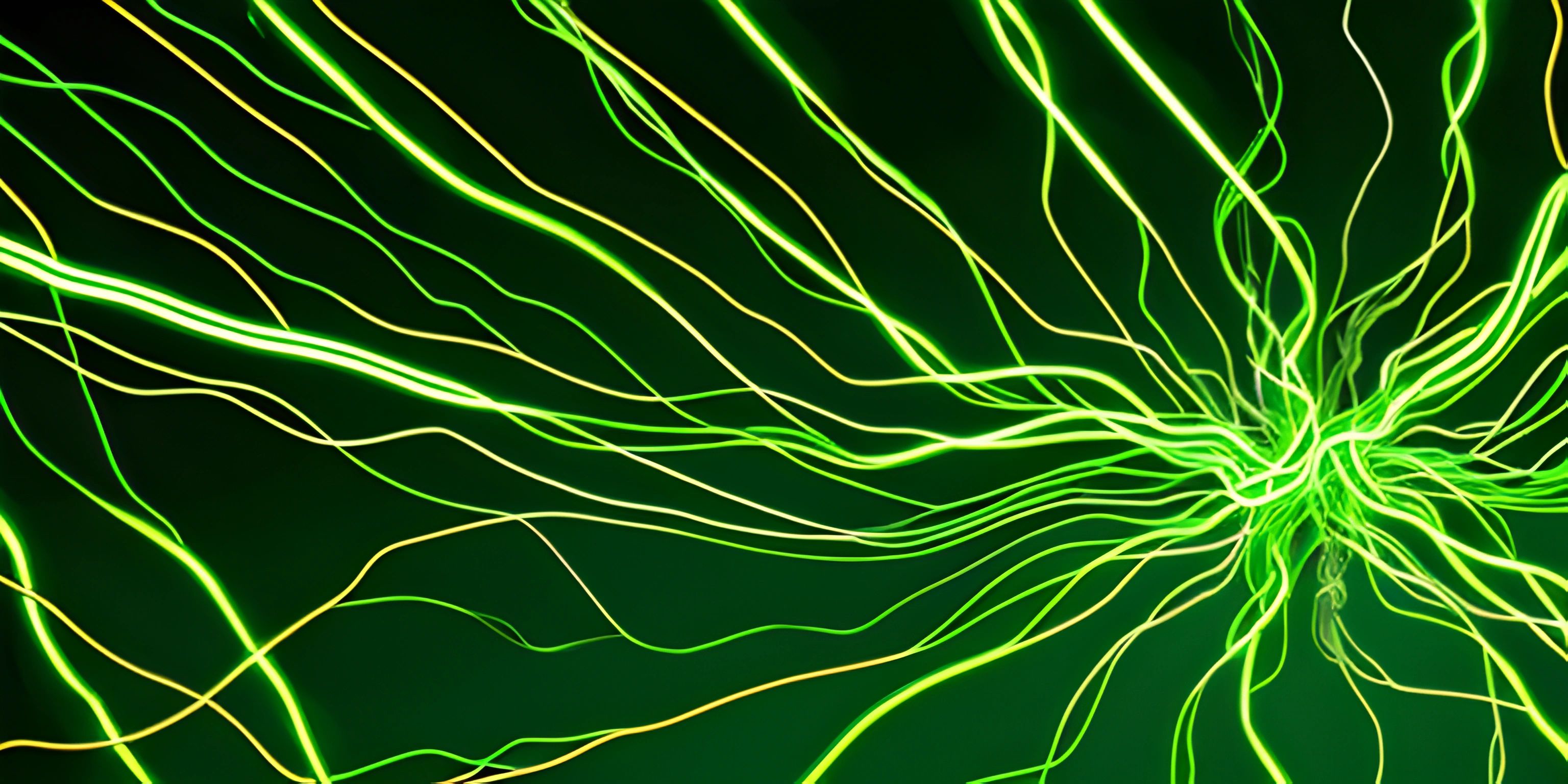
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Every now and then, we need our applications to have a little chit-chat with users. In Node.js, the readline module is the perfect companion for creating interactive command-line interfaces. It allows us to read input from users and display output, making it an essential tool for building communication bridges between our programs and the human world.
Readline Module Overview
The readline module is built into Node.js, which means we don't have to install any additional packages (phew!). To start using it, all we need to do is require the module like this:
const readline = require("readline");
Now that we've got readline in our toolbox, let's explore how to create a simple interface.
Creating a Readline Interface
To create a readline interface, we need to call the createInterface
method and pass in an object with the input and output streams. The most common configuration is to use the process.stdin
(standard input) and process.stdout
(standard output) streams, like so:
const rl = readline.createInterface({ input: process.stdin, output: process.stdout });
With our shiny new readline interface (rl
), we can now easily ask questions and receive answers from the user.
Asking Questions and Reading User Input
To ask a question, we use the question
method on our readline interface. This method takes two arguments: the question text and a callback function that will be executed when the user provides an answer. The user's answer will be passed to the callback as its first argument. Here's an example:
rl.question("What's your favorite programming language? ", (answer) => { console.log(`Ah, so you like ${answer}!`); rl.close(); });
When the user types their answer and hits Enter, the callback function will be called, and we'll display the response using console.log
. Don't forget to close the interface with rl.close()
when you're done!
Wrapping It Up
Now you know how to create a readline interface in Node.js and communicate with users through questions and answers. With this newfound knowledge, you're ready to create engaging command-line applications that interact with users in a smooth, natural way. So go on, start a conversation with your code and see where it takes you!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: File I/O (Reading) (psst, it's free!).
FAQ
What is the readline module in Node.js?
The readline module in Node.js is a built-in module that provides an interface for reading input and displaying output, line by line, from a readable stream (like the command line). It's particularly useful for creating interactive command-line applications or reading data from a file line by line.
How do I use the readline module in my Node.js application?
To use the readline module in your Node.js application, you need to require the module and create an interface with it. Here's a simple example to get you started:
const readline = require("readline"); const rl = readline.createInterface({ input: process.stdin, output: process.stdout });
Now, you can use rl.question()
to ask questions and collect user input, or rl.on('line', callback)
to read input line by line.
How do I process user input with the readline module?
You can process user input with the readline module by using the rl.question()
method. This method takes two arguments: the question to be displayed and a callback function to handle the user's response. Here's an example:
rl.question("What's your name? ", (answer) => { console.log(`Hello, ${answer}!`); rl.close(); });
How can I read data from a file line by line using the readline module?
To read data from a file line by line using the readline module, you'll need to use the fs.createReadStream()
method from the fs
module to create a readable stream. Then, pass this stream as the input for the readline interface. Here's an example:
const fs = require("fs"); const readline = require("readline"); const fileStream = fs.createReadStream("example.txt"); const rl = readline.createInterface({ input: fileStream, output: process.stdout }); rl.on("line", (line) => { console.log(`Line from file: ${line}`); });
How do I close the readline interface once I'm done with it?
To close the readline interface after you've finished using it, simply call the rl.close()
method. This will also remove any input and output event listeners, ensuring a clean exit from your application.