MIPS Assembly Introduction
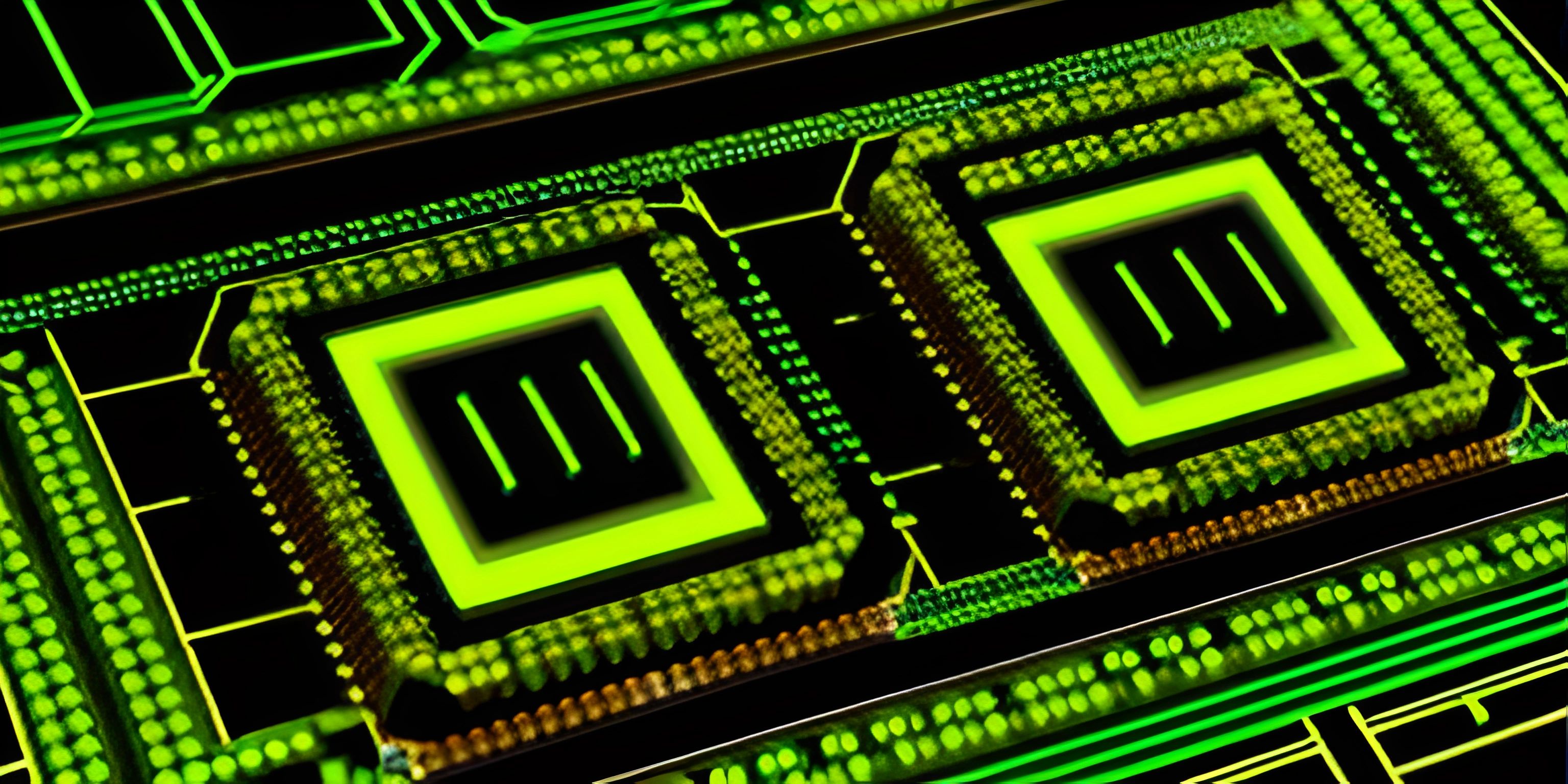
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of MIPS Assembly language! It's like learning a secret code that only the cool kids know. Don't worry, we'll make sure you fit right in. In this journey, we'll cover the basic concepts and fundamentals of MIPS Assembly language to help you get started.
What is MIPS Assembly?
MIPS Assembly is a low-level programming language used for programming MIPS (Microprocessor without Interlocked Pipeline Stages) processors. MIPS Assembly is human-readable, but it's much closer to the language that computers understand (machine code) than high-level languages like Python or Java.
Why learn MIPS Assembly?
You might be wondering, "Why should I learn MIPS Assembly when there are so many high-level languages to choose from?" It's a valid question! But, here's the thing: learning MIPS Assembly gives you a deeper understanding of how computers work at their core. It's like learning the secrets of the universe, but for computers.
Registers
In MIPS Assembly, we use registers to store and manipulate data. Registers are like tiny storage units within the processor, each capable of holding a specific type of information. In MIPS, there are 32 general-purpose registers, numbered from $0
to $31
.
For example, here's a simple MIPS Assembly code snippet to add two numbers:
addi $8, $0, 5 # $8 = 5 addi $9, $0, 7 # $9 = 7 add $10, $8, $9 # $10 = $8 + $9
This code adds 5
and 7
and stores the result in register $10
. Don't worry if you don't understand everything just yet; we're just starting to scratch the surface.
Instructions
MIPS Assembly language is made up of instructions. Instructions are simple, human-readable commands that tell the processor what to do. The code snippet above contains three instructions: addi
and add
.
addi
(short for "add immediate") adds an immediate value (a constant) to a register and stores the result in another register.add
adds the values stored in two registers and stores the result in a third register.
We'll dive deeper into different types of instructions as we progress.
Assembler Directives
Assembler directives are special commands that give the assembler (the program that translates MIPS Assembly code into machine code) additional information about how to process the code. They usually start with a period (.
) and are followed by a keyword.
For example, the .data
and .text
directives are commonly used to separate the data and code sections of a MIPS Assembly program:
.data myVar: .word 42 .text .globl main main: lw $8, myVar
This code snippet defines a variable myVar
with the value 42
in the data section and loads its value into register $8
in the code section.
Conclusion
We've just dipped our toes into the MIPS Assembly ocean, but don't worry, there's much more to explore and discover. As you dive deeper, you'll learn about various instructions, addressing modes, and even how to write your own MIPS Assembly programs. Soon enough, you'll be part of the cool kids club, speaking the secret language of MIPS Assembly. So buckle up and enjoy the ride!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is MIPS Assembly language?
MIPS Assembly language is a low-level programming language used for MIPS (Microprocessor without Interlocked Pipeline Stages) processors. It is a human-readable representation of the machine code that the processor executes. MIPS Assembly provides a more intuitive way of programming compared to writing binary code, and it is typically used for low-level tasks, such as operating system development, firmware, and device drivers.
What are some basic concepts in MIPS Assembly?
Some basic concepts in MIPS Assembly include:
- Registers: Small, fast storage locations within the CPU that hold data for operations.
- Instructions: The fundamental operations that the CPU can perform, such as addition, multiplication, and branching.
- Addressing modes: Different ways of specifying the location of data in memory.
- Assembly directives: Special instructions that tell the assembler how to interpret the code and generate machine code.
How do I write a simple MIPS Assembly program?
To write a simple MIPS Assembly program, follow these steps:
- Open a text editor and type in your assembly instructions.
- Save the file with a ".asm" extension.
- Use an assembler tool, such as the MIPS Assembler and Runtime Simulator (MARS), to convert your assembly code into machine code.
- Run the machine code on a MIPS processor or simulator. Here's an example of a simple program that adds two numbers:
.data # Data segment num1: .word 5 # Define a 32-bit integer named num1 with a value of 5 num2: .word 7 # Define a 32-bit integer named num2 with a value of 7 result: .space 4 # Allocate space for the result (4 bytes) .text # Code segment main: # Main function label lw $t0, num1 # Load the value of num1 into register $t0 lw $t1, num2 # Load the value of num2 into register $t1 add $t2, $t0, $t1 # Add the values in registers $t0 and $t1 and store the result in register $t2 sw $t2, result # Store the result from register $t2 in memory at the address labeled "result"
What are some common MIPS Assembly instructions?
Some common MIPS Assembly instructions include:
- 'add': Adds two registers and stores the result in a third register.
- 'sub': Subtracts one register from another and stores the result in a third register.
- 'lw': Loads a word (4 bytes) from memory into a register.
- 'sw': Stores a word (4 bytes) from a register to memory.
- 'beq': Branches to a specified address if two registers are equal.
- 'j': Unconditional jump to a specified address.
How can I learn more about MIPS Assembly?
To learn more about MIPS Assembly, consider the following resources:
- MIPS Assembly tutorials and reference guides available online.
- Books on MIPS Assembly programming.
- Online forums and communities related to MIPS Assembly programming.
- Courses and workshops on computer architecture and assembly programming.