Exploring the p5.js Draw Function
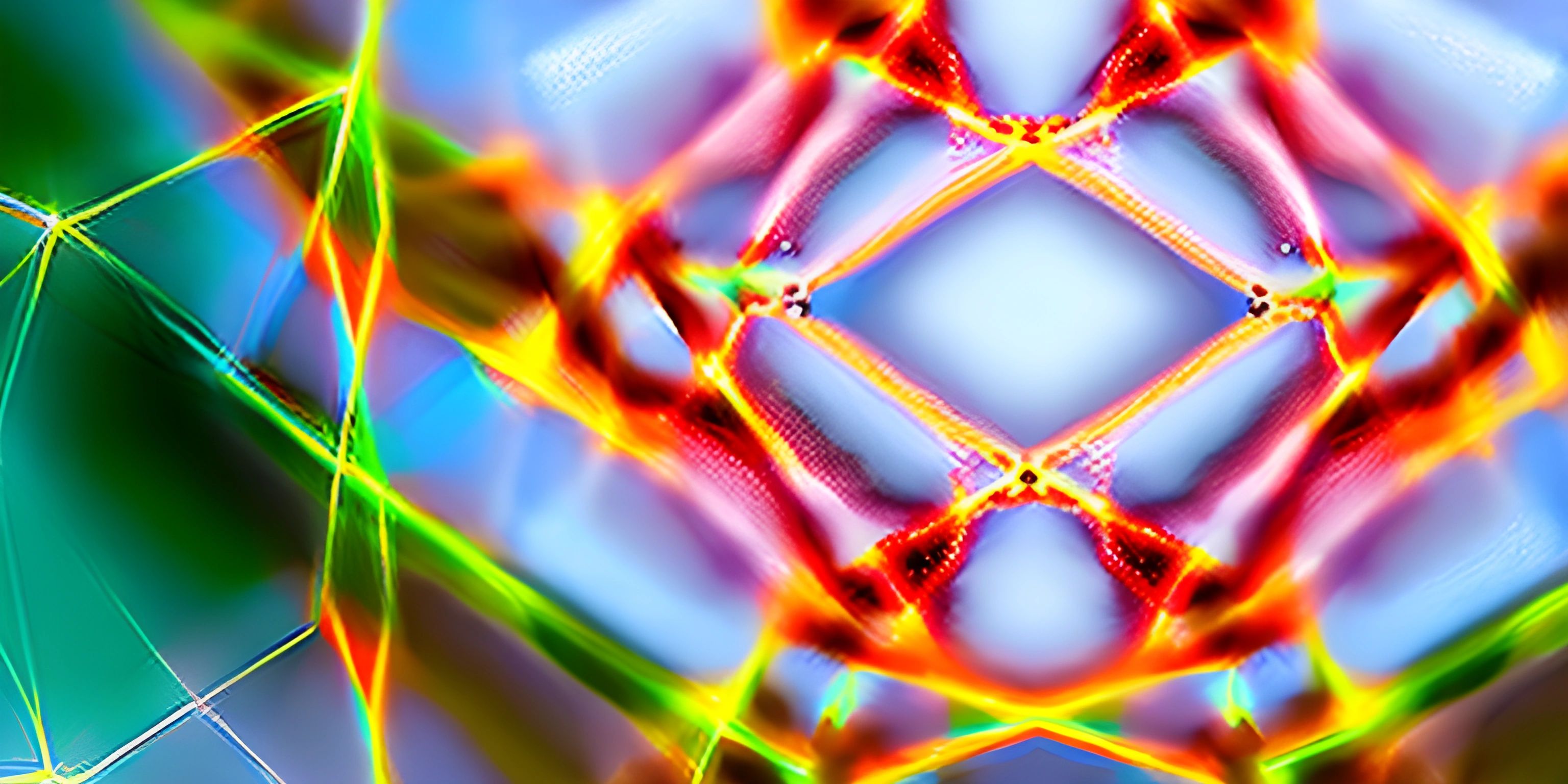
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Let's go on an artistic journey with p5.js! Imagine you're a painter, but instead of a brush, you have code, and instead of a canvas, you have your browser window. The p5.js library is like your trusty paint set, and the draw
function is your secret technique to turn static doodles into dynamic masterpieces.
Getting Started with p5.js
Before diving into the draw
function, let's take a quick look at p5.js itself. It’s a JavaScript library that makes coding accessible for artists and designers. You can easily include p5.js in your HTML by adding the following script tag:
<script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script>
For more details on setting up your first p5.js sketch, check out our p5.js setup guide.
The Setup and Draw Functions
In p5.js, there are two main functions you need to get familiar with: setup
and draw
. Think of setup
as the place where you prepare your workspace, and draw
as where the magic happens repeatedly.
The Setup Function
The setup
function runs once, when the program starts. It’s used to set the initial state of your sketch, like defining the canvas size:
function setup() { createCanvas(400, 400); // Create a 400x400 pixel canvas background(255); // Set background color to white }
The Draw Function
The draw
function is where you continuously draw on the canvas. It runs in a loop, refreshing the canvas at 60 frames per second by default. This is perfect for creating animations!
Here’s a simple example that draws a moving circle:
let x = 0; function setup() { createCanvas(400, 400); background(255); } function draw() { background(255); // Clear the canvas ellipse(x, height / 2, 50, 50); // Draw a circle x += 2; // Move the circle 2 pixels to the right }
In this example, the circle starts at the left edge and moves right. Each frame, background(255)
clears the canvas, and ellipse
draws the circle at its new position.
Animation Using the Draw Function
Now, let’s get a bit fancier. We can add variables and incorporate more shapes and colors to create a more dynamic animation.
Example: Bouncing Ball
Here’s an example of a bouncing ball that changes color when it hits the edges:
let x = 200; let y = 200; let xSpeed = 3; let ySpeed = 3; let r = 25; let col; function setup() { createCanvas(400, 400); col = color(random(255), random(255), random(255)); } function draw() { background(255); // Move the ball x += xSpeed; y += ySpeed; // Bounce off edges if (x > width - r || x < r) { xSpeed *= -1; col = color(random(255), random(255), random(255)); // Change color } if (y > height - r || y < r) { ySpeed *= -1; col = color(random(255), random(255), random(255)); // Change color } fill(col); noStroke(); ellipse(x, y, r * 2, r * 2); }
In this code:
- Variables
x
,y
keep track of the ball’s position. - Variables
xSpeed
,ySpeed
control its speed. - When the ball hits the canvas edges, it bounces by reversing its speed and changes color.
Advanced Techniques
What if you want to create more complex animations, like moving multiple objects or controlling animation speed? Let’s explore some advanced p5.js functionalities.
Example: Multiple Moving Balls
You can use arrays to handle multiple objects. Here’s an example with multiple balls bouncing around:
let balls = []; function setup() { createCanvas(400, 400); for (let i = 0; i < 10; i++) { balls.push(new Ball(random(width), random(height), random(10, 30))); } } function draw() { background(255); for (let ball of balls) { ball.move(); ball.display(); ball.checkEdges(); } } class Ball { constructor(x, y, r) { this.x = x; this.y = y; this.r = r; this.xSpeed = random(2, 5); this.ySpeed = random(2, 5); this.col = color(random(255), random(255), random(255)); } move() { this.x += this.xSpeed; this.y += this.ySpeed; } display() { fill(this.col); noStroke(); ellipse(this.x, this.y, this.r * 2, this.r * 2); } checkEdges() { if (this.x > width - this.r || this.x < this.r) { this.xSpeed *= -1; this.col = color(random(255), random(255), random(255)); } if (this.y > height - this.r || this.y < this.r) { this.ySpeed *= -1; this.col = color(random(255), random(255), random(255)); } } }
In this example, we create a Ball
class to encapsulate properties and behaviors of each ball. This helps organize the code and makes it easier to manage multiple balls.
Optimizing Your Animations
Animations can be resource-intensive, so it’s important to optimize your code. Here are a few tips:
- Minimize Background Redraws: Only redraw the background if necessary. For instance, if only a small part of the canvas changes, consider clearing only that part.
- Use
frameRate()
: Control the frame rate usingframeRate()
to balance performance and smoothness. - Efficient Object Management: Use arrays or other data structures to manage and update multiple objects efficiently.
For more tips on optimizing performance, check out our optimization guide.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
What is the `draw` function in p5.js?
The draw
function in p5.js runs continuously and is used to create animations. It refreshes the canvas at 60 frames per second by default, allowing you to make dynamic and interactive sketches.
How can I make an object bounce off the edges of the canvas?
To make an object bounce, reverse its speed when it hits the canvas edges. Check the object's position and, if it exceeds the canvas boundaries, multiply its speed by -1 to change its direction.
Can I animate multiple objects in p5.js?
Yes, you can animate multiple objects by using arrays to store and manage them. Define a class for the objects and use loops to update and display each object in the draw
function.
How do I control the speed of my animation in p5.js?
Control the speed of your animation by adjusting the variables that determine object movement. Additionally, use the frameRate()
function to set the number of frames displayed per second.
What are some best practices for optimizing animations in p5.js?
Optimize animations by minimizing background redraws, using frameRate()
to control the frame rate, and efficiently managing objects with arrays or other data structures. This helps improve performance and maintain smooth animations.