P5.js Reference: Functions and Utilities
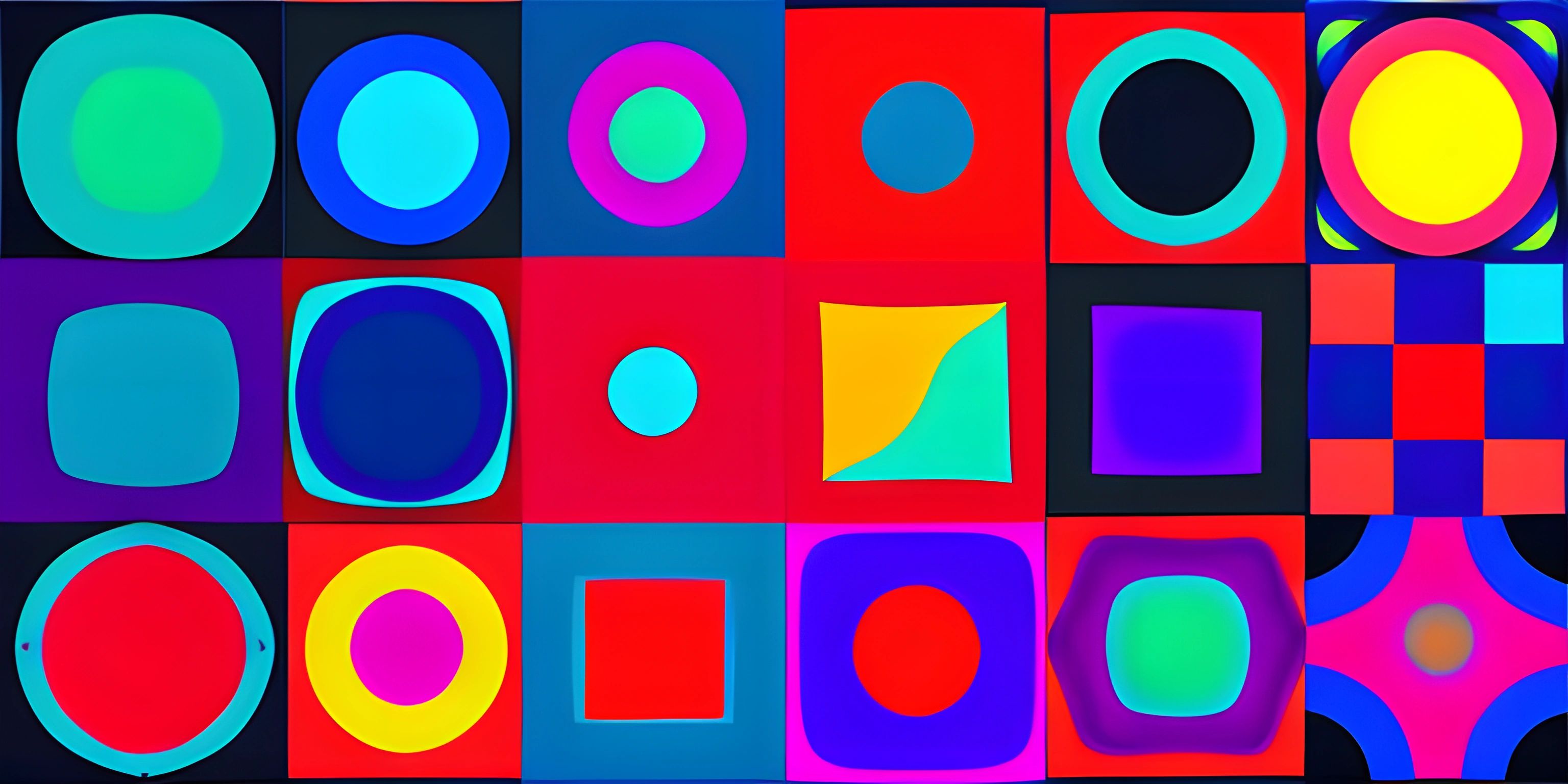
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
P5.js is a powerful JavaScript library that simplifies the creation of interactive graphics, animations, and multimedia applications in the browser. In this reference, we'll take a closer look at the key functions and utilities provided by p5.js to help you create stunning projects with ease.
Setup and Draw Functions
When working with p5.js, you'll often start by defining two essential functions: setup()
and draw()
.
setup()
The setup()
function is called once when the program begins, and it's where you can perform initial configurations, such as setting the canvas size or loading external resources.
function setup() { createCanvas(800, 600); }
draw()
The draw()
function is called repeatedly in a loop, allowing you to create animations by updating the screen contents. This loop is executed at a default frame rate of 60 FPS, but you can modify it using frameRate()
.
function draw() { background(0); ellipse(mouseX, mouseY, 50, 50); }
Graphics
P5.js provides a variety of functions for creating and manipulating graphics on the canvas.
Basic Shapes
You can draw basic shapes such as circles, rectangles, and lines using functions like ellipse()
, rect()
, and line()
.
ellipse(50, 50, 25, 25); // Draw a circle rect(100, 100, 50, 50); // Draw a square line(0, 0, 100, 100); // Draw a diagonal line
Colors and Styles
Customize the appearance of your graphics with functions like fill()
, stroke()
, and strokeWeight()
.
fill(255, 0, 0); // Red fill color stroke(0, 255, 0); // Green stroke color strokeWeight(5); // Stroke weight of 5 pixels
Transformations
Manipulate graphics on the canvas with translation, rotation, and scaling using translate()
, rotate()
, and scale()
functions.
translate(100, 100); // Move the origin to (100, 100) rotate(PI / 4); // Rotate the canvas by 45 degrees scale(2, 2); // Scale the canvas by a factor of 2
Interactivity
P5.js makes it simple to interact with users through keyboard, mouse, and touch events.
Mouse Events
Capture mouse events like clicks and movements using functions such as mousePressed()
, mouseReleased()
, and mouseMoved()
.
function mousePressed() { console.log("Mouse button pressed"); } function mouseReleased() { console.log("Mouse button released"); } function mouseMoved() { console.log("Mouse moved"); }
Keyboard Events
Detect keyboard input with functions like keyPressed()
, keyReleased()
, and keyTyped()
.
function keyPressed() { console.log("Key pressed:", keyCode); } function keyReleased() { console.log("Key released:", keyCode); } function keyTyped() { console.log("Key typed:", key); }
Touch Events
Respond to touch events on mobile devices using functions such as touchStarted()
, touchMoved()
, and touchEnded()
.
function touchStarted() { console.log("Touch started"); } function touchMoved() { console.log("Touch moved"); } function touchEnded() { console.log("Touch ended"); }
Utilities
P5.js provides handy utility functions for common tasks like generating random numbers or mapping values.
Random Values
Generate random numbers using the random()
function.
let randomValue = random(0, 100); // A random value between 0 and 100
Mapping Values
Map a value from one range to another using the map()
function.
let mappedValue = map(50, 0, 100, 0, 255); // Map 50 from range 0-100 to range 0-255
This reference only scratches the surface of the numerous functions and utilities available in p5.js. For a more comprehensive list, check out the official p5.js reference. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).