Creating Smooth and Dynamic Animations with p5.js
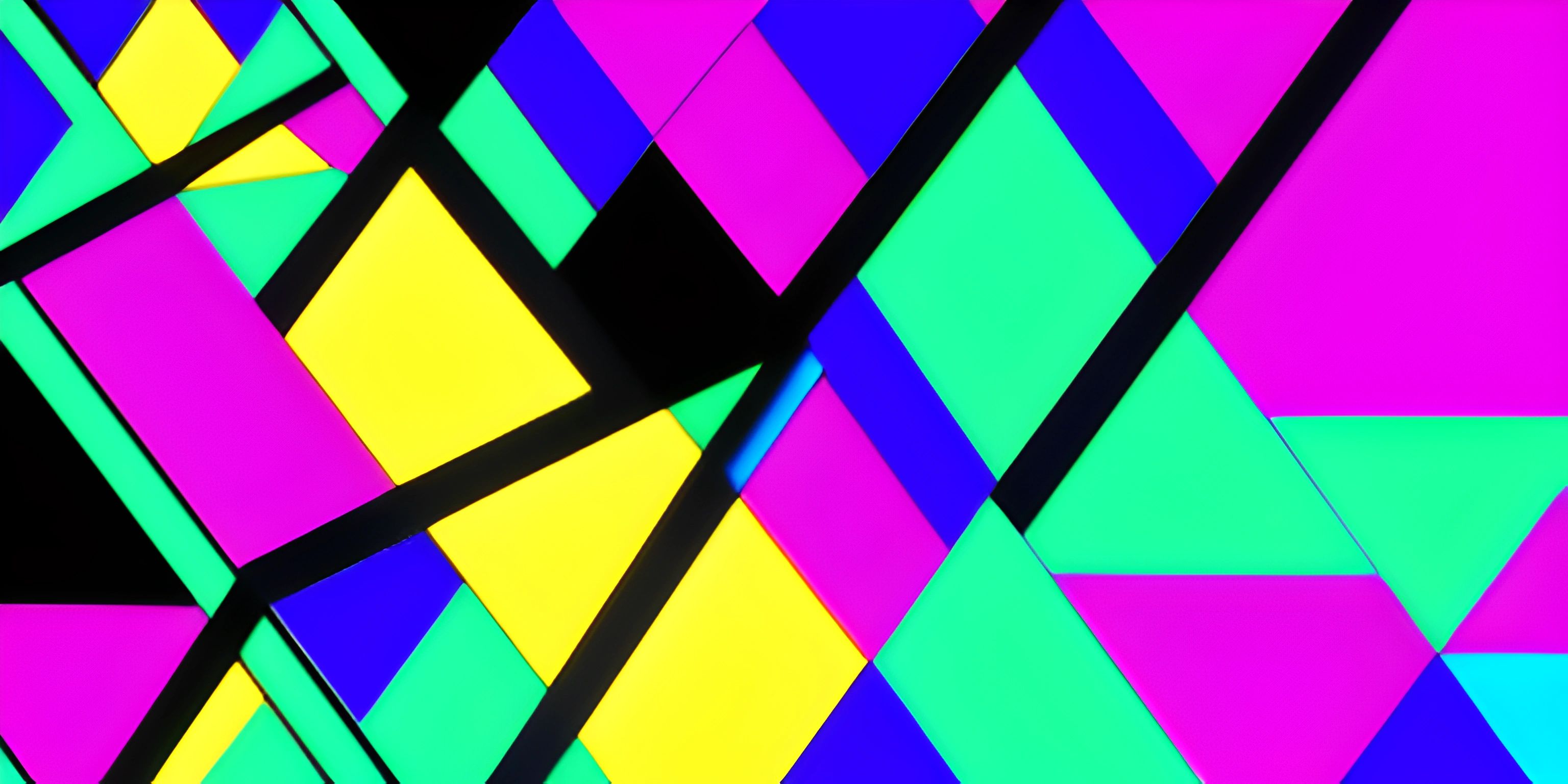
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Animating with p5.js is like having your very own digital sketchbook, giving you the power to bring your creations to life! In this guide, we'll explore how to make smooth and dynamic animations that interact with user input and respond to changes in real-time.
Getting Started with p5.js
Before we dive into the world of animation, let's make sure we have everything set up. If you're new to p5.js, be sure to set up the p5.js environment and familiarize yourself with its basic drawing functions.
The Animation Loop
The beating heart of a p5.js animation is the draw()
function. This function runs continuously in a loop, allowing you to update the visuals on the canvas. To create a smooth animation, the draw()
function should be designed to execute multiple times per second. Here's an example of a simple animation loop:
function setup() { createCanvas(600, 400); } function draw() { background(255); ellipse(mouseX, mouseY, 50, 50); }
In this example, we draw an ellipse that follows the mouse cursor. The background(255)
call clears the canvas each frame, giving the illusion of motion as the ellipse moves.
Controlling Animation Speed
By default, p5.js runs the draw()
function at 60 frames per second. However, we can control the frame rate using the frameRate()
function, which accepts a number as its argument. For example, frameRate(30)
sets the frame rate to 30 frames per second.
function setup() { createCanvas(600, 400); frameRate(30); } function draw() { background(255); ellipse(mouseX, mouseY, 50, 50); }
Keep in mind that slower frame rates may result in less smooth animations, but they can also reduce processing demands.
Creating Dynamic Animations
Our current animation is quite basic, so let's step it up a notch by making it more dynamic. One way to create dynamic animations is by using variables that change over time. For instance, let's make the size of our ellipse grow and shrink in a continuous loop:
let size = 50; let growing = true; function setup() { createCanvas(600, 400); } function draw() { background(255); if (growing) { size += 1; } else { size -= 1; } if (size > 100 || size < 50) { growing = !growing; } ellipse(mouseX, mouseY, size, size); }
Now, the ellipse changes size as it follows the mouse, creating a more dynamic and engaging animation.
Interacting with User Input
To make our animations truly interactive, we can respond to user input. For instance, let's change the color of our ellipse based on the mouse button being pressed:
let size = 50; let growing = true; let fillColor = 0; function setup() { createCanvas(600, 400); } function draw() { background(255); if (growing) { size += 1; } else { size -= 1; } if (size > 100 || size < 50) { growing = !growing; } if (mouseIsPressed) { fillColor = 255; } else { fillColor = 0; } fill(fillColor); ellipse(mouseX, mouseY, size, size); }
Now, when the mouse button is pressed, the ellipse turns white, and when it's released, it turns black.
Embark on your animation journey with p5.js and create incredible interactive experiences. The possibilities are limited only by your imagination! Don't forget to explore p5.js documentation and experiment with different functions to bring your ideas to life. Happy animating!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Taking User Input (psst, it's free!).