An Overview of p5.js Library
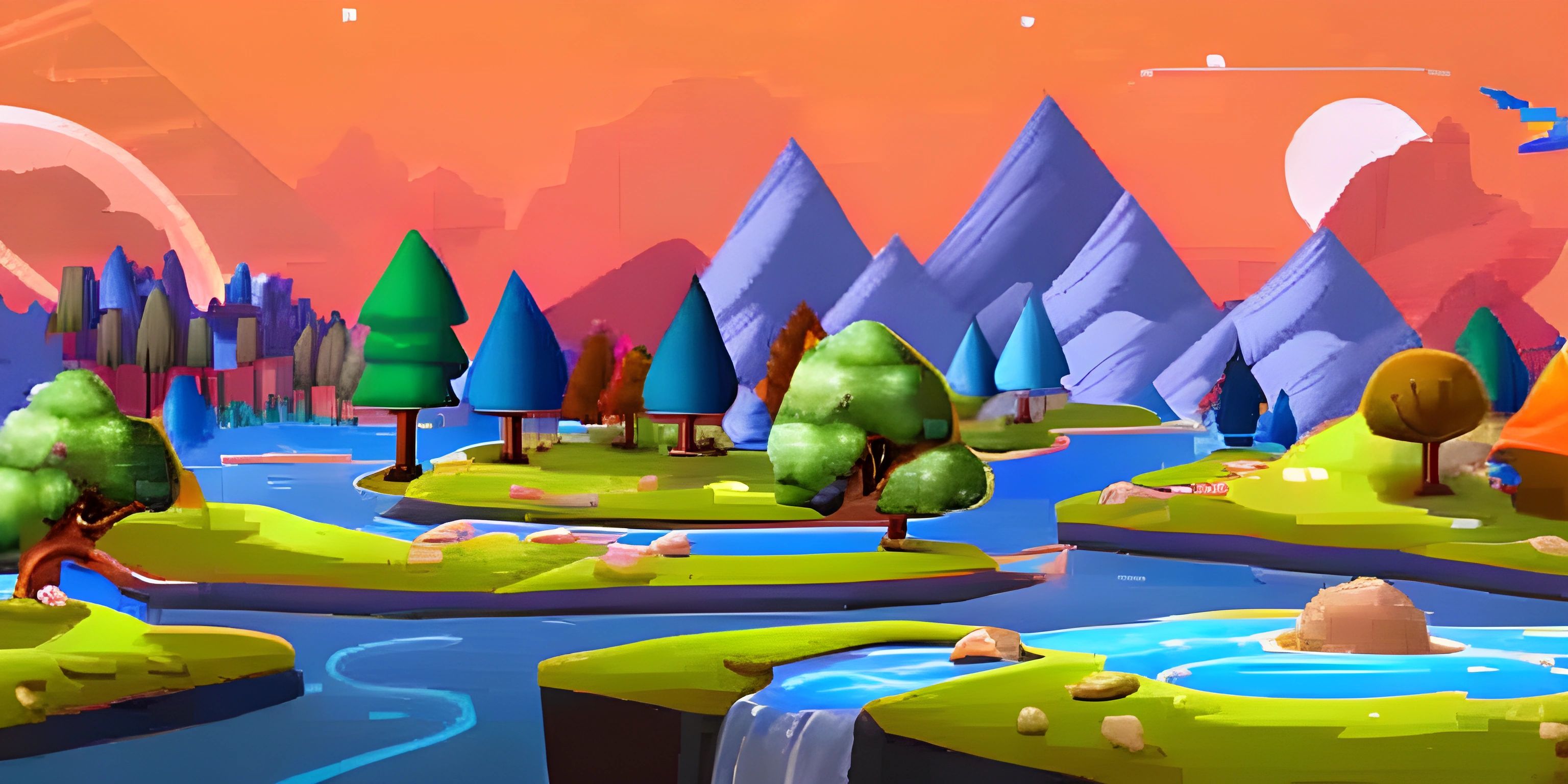
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the wonderful world of p5.js, a JavaScript library that makes creative coding a breeze! If you've ever wanted to draw, animate, or just have some fun with code, you're in the right place. p5.js is like the Swiss Army knife for artists and developers alike, combining the best of both worlds.
What is p5.js?
p5.js is a JavaScript library that focuses on making coding more accessible for artists, designers, educators, and anyone else who wants to create beautiful visuals. By building on the capabilities of the Processing language, p5.js brings the magic of creative coding to the web.
Why Use p5.js?
Imagine trying to draw a smiley face using plain JavaScript. It’s like painting the Mona Lisa with a toothbrush. p5.js, on the other hand, gives you a full palette and canvas, making it much simpler to create intricate designs and animations. Here’s why you might want to use p5.js:
- Simplicity: p5.js abstracts away many of the complexities of standard JavaScript, making it easier to focus on your creative vision.
- Flexibility: It works seamlessly with other web technologies like HTML, CSS, and other JavaScript libraries.
- Community: A thriving community provides numerous resources, tutorials, and examples to get you started.
Getting Started with p5.js
Setting up p5.js is as easy as pie. All you need is a web browser and a text editor.
Including p5.js in Your Project
You can include p5.js in your HTML file by adding the following script tag in the <head>
section:
<!DOCTYPE html> <html> <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> </head> <body> <script src="sketch.js"></script> </body> </html>
This script tag pulls in the p5.js library from a CDN, so you don’t need to download anything. Next, create a file named sketch.js
where all your p5.js code will live.
Basic Structure of a p5.js Sketch
Every p5.js sketch has two main functions: setup()
and draw()
.
- setup(): This function runs once at the beginning. It’s typically used for initializing variables, creating the canvas, and setting up the environment.
- draw(): This function loops continuously and is used to render your visuals.
Here’s a simple example to illustrate:
function setup() { createCanvas(400, 400); // Create a 400x400 pixel canvas } function draw() { background(220); // Set background color to light gray ellipse(200, 200, 100, 100); // Draw a circle at (200, 200) with a diameter of 100 }
This code creates a 400x400 pixel canvas and draws a circle in the center. Easy, right?
Drawing with p5.js
Basic Shapes
p5.js makes drawing shapes straightforward. Here are some basic shapes you can draw:
- Rectangle:
rect(x, y, width, height)
- Circle:
ellipse(x, y, width, height)
(orcircle(x, y, diameter)
) - Line:
line(x1, y1, x2, y2)
For example, to draw a rectangle and a line:
function setup() { createCanvas(400, 400); } function draw() { background(220); rect(50, 50, 150, 100); // Draw a rectangle line(0, 0, 400, 400); // Draw a diagonal line }
Colors and Styles
p5.js also provides functions to set colors and styles:
- fill(color): Sets the fill color for shapes.
- stroke(color): Sets the color for the shape’s outline.
- noFill(): Disables filling shapes.
- noStroke(): Disables drawing outlines.
Here’s how you can use these:
function setup() { createCanvas(400, 400); } function draw() { background(220); fill("blue"); // Fill shapes with blue color rect(50, 50, 150, 100); noFill(); // Disable filling shapes stroke("red"); // Outline shapes with red color ellipse(200, 200, 100, 100); }
Animations
Creating animations in p5.js is like flipping through a flipbook. The draw()
function runs 60 times per second by default, allowing you to update the visuals continuously.
Here’s an example of animating a moving circle:
let x = 0; function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(x, 200, 50, 50); x += 2; // Move the circle to the right }
In this example, the circle moves to the right by increasing the x
coordinate by 2 pixels in each frame.
Interactivity
p5.js isn’t just for animations; it’s also great for creating interactive applications. You can use mouse and keyboard events to make your sketches more dynamic.
Mouse Interaction
You can use built-in variables like mouseX
and mouseY
to get the current position of the mouse. Here’s an example:
function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(mouseX, mouseY, 50, 50); // Draw a circle at the mouse position }
Keyboard Interaction
You can also respond to keyboard events using functions like keyPressed()
and keyReleased()
. Here’s an example:
let x = 200; let y = 200; function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(x, y, 50, 50); } function keyPressed() { if (keyCode === UP_ARROW) { y -= 10; // Move up } else if (keyCode === DOWN_ARROW) { y += 10; // Move down } else if (keyCode === LEFT_ARROW) { x -= 10; // Move left } else if (keyCode === RIGHT_ARROW) { x += 10; // Move right } }
In this example, the circle moves in response to the arrow keys.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Advanced Data Types (psst, it's free!).
FAQ
Can I use p5.js with other JavaScript libraries?
Absolutely! p5.js is designed to work well with other JavaScript libraries. You can use it alongside frameworks like React, Vue, or even jQuery. Just make sure to manage your script tags and dependencies properly.
Is p5.js suitable for beginners?
Yes, p5.js is excellent for beginners. Its simplicity and ease of use make it a popular choice for those new to programming, especially in the context of creative coding and visual arts.
Do I need to install p5.js to use it?
No installation is needed if you use the CDN link in your HTML file. However, you can also download p5.js and host it locally if you prefer.
Can I create 3D graphics with p5.js?
Yes, p5.js supports 3D graphics through its WEBGL
renderer. You can create 3D shapes, animations, and even interactive 3D applications.
Where can I find more resources and tutorials for p5.js?
The official p5.js website has extensive documentation, examples, and tutorials. Additionally, there is a vibrant community of artists and developers who share their work and insights online.