p5.js Overview
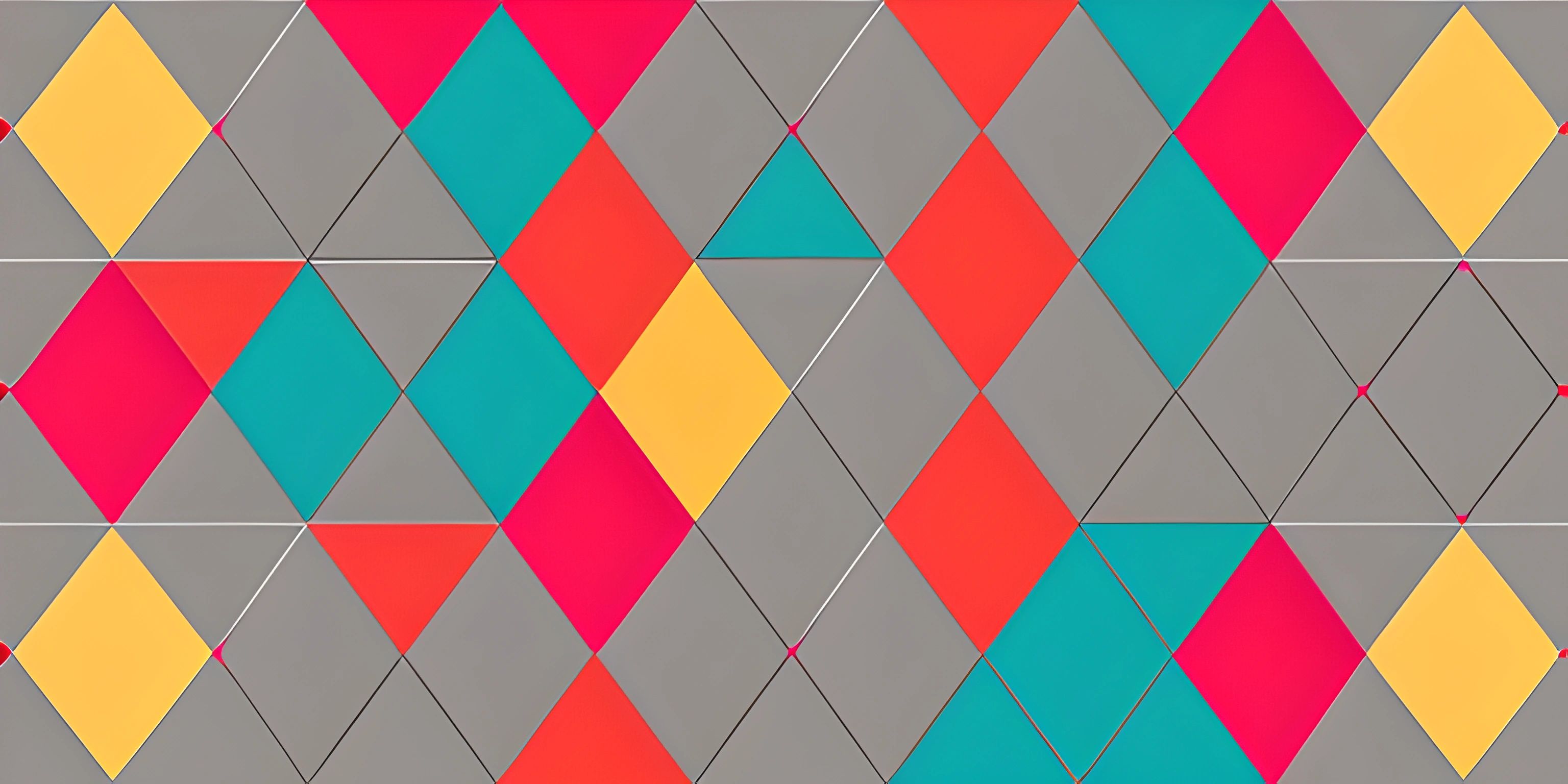
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine a tool that simplifies the process of creating visually stunning and interactive web applications. Well, p5.js is just that! This powerful JavaScript library enables creative coding by making graphics and interactivity as fluid and intuitive as possible.
What is p5.js?
p5.js is a JavaScript library, built on top of the HTML5 canvas API, that makes it easy to create graphics, animations, and interactive experiences on the web. Inspired by the Processing programming environment, it aims to bring the joy of creative coding to a wider audience by leveraging the power of JavaScript and the web.
Getting Started with p5.js
To begin using p5.js, all you need to do is include the p5.js library in your HTML file, like so:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>My p5.js Sketch</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> <script src="sketch.js"></script> </head> <body> </body> </html>
With the library included, create a new file called sketch.js
. This is where you'll write your p5.js code. The p5.js library provides two essential functions: setup()
and draw()
.
function setup() { createCanvas(800, 400); } function draw() { background(255); ellipse(400, 200, 50, 50); }
The setup()
function is executed once when the program starts and can be used for initializing variables, setting up the canvas, and other setup tasks. In this example, we create a canvas with a width of 800 pixels and a height of 400 pixels.
The draw()
function is called continuously, creating a loop. In this example, it sets the background color to white (255) and draws a circle (ellipse) at the center of the canvas with a width and height of 50 pixels.
Creative Coding with p5.js
p5.js offers a wide range of functions for drawing shapes, manipulating colors, working with typography, and handling user input. Here's a simple example of an interactive sketch that changes color based on mouse position:
function setup() { createCanvas(800, 400); } function draw() { let r = map(mouseX, 0, width, 0, 255); let g = map(mouseY, 0, height, 0, 255); let b = map(mouseX + mouseY, 0, width + height, 0, 255); background(r, g, b); fill(255); ellipse(mouseX, mouseY, 50, 50); }
In this example, we use the map()
function to convert the mouse's x and y positions to RGB color values. The background color is then set to these values, creating an interactive, color-changing canvas.
Beyond the Basics
p5.js is a versatile library with a vast ecosystem of add-ons and community-contributed libraries. You can explore further possibilities like 3D graphics, audio synthesis, and even computer vision!
Dive into the world of p5.js, and let your imagination run wild as you create mesmerizing visuals and engaging interactive experiences. The only limit is your creativity!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Story Builder (psst, it's free!).