What is p5.js?
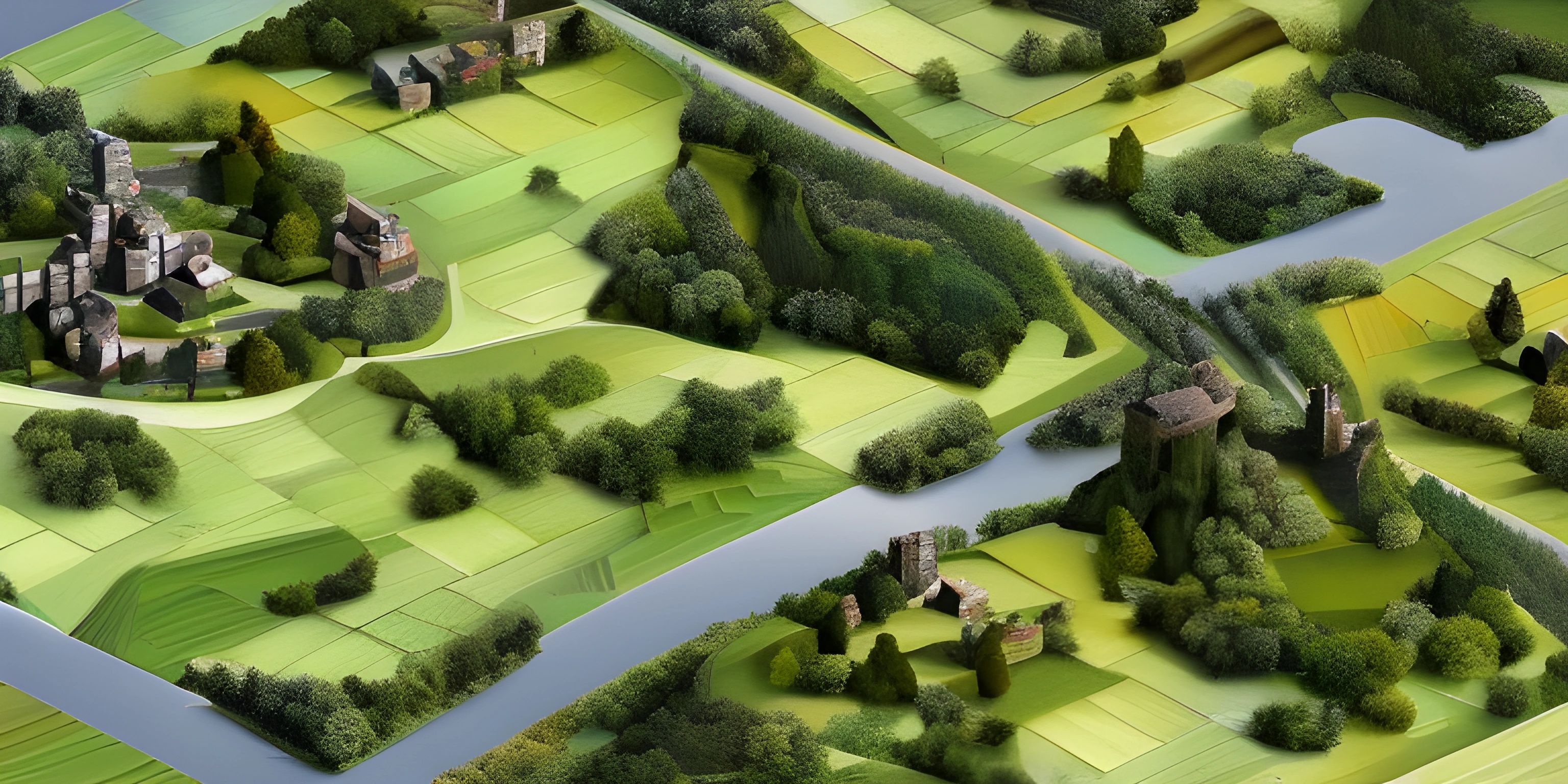
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever felt like your code could use a splash of color or a dash of visual pizzazz? Enter p5.js, a JavaScript library that turns your browser into a canvas ready for your artistic expression. Whether you're a beginner or an experienced coder looking to explore new creative frontiers, p5.js offers a delightful way to blend coding with art.
What is p5.js?
p5.js is an open-source JavaScript library that makes it easy to create interactive graphics and animations right in your web browser. Think of it as a friendly companion to the HTML5 canvas element, providing an intuitive API to draw shapes, render text, and create dynamic visuals. Inspired by the Processing language, p5.js aims to make coding accessible and enjoyable, especially for artists, designers, and educators.
Setting Up p5.js
Before you can paint your digital masterpiece, you need to set up p5.js. Luckily, it's as simple as making toast. First, include the p5.js library in your HTML file.
Here's a basic HTML template to get you started:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>My p5.js Sketch</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> </head> <body> <script> // Your p5.js code will go here </script> </body> </html>
With this setup, you can start coding your first p5.js sketch. Ready, set, draw!
Hello World in p5.js
Let's kick things off with the classic "Hello World" example, but this time, let's make it visual. In p5.js, you'll typically use two main functions: setup
and draw
.
setup
: Runs once when the program starts. It's used to set initial properties.draw
: Continuously executes the lines of code within it, allowing for animation.
Here's a simple sketch that draws a friendly "Hello World!" on the screen:
function setup() { createCanvas(400, 400); // Creates a 400x400 pixel canvas background(220); // Sets the background color to a light gray } function draw() { textSize(32); // Sets the text size to 32 pixels fill(50); // Sets the text fill color text("Hello World!", 100, 200); // Draws the text at coordinates (100, 200) }
Drawing Shapes
In the world of p5.js, shapes are your best friends. From basic rectangles and ellipses to complex polygons, you can draw a variety of shapes with ease. Here's how you can draw some basic shapes:
function setup() { createCanvas(400, 400); background(220); } function draw() { fill(255, 0, 0); // Red fill color rect(50, 50, 100, 100); // Draws a rectangle at (50, 50) with width and height of 100 fill(0, 255, 0); // Green fill color ellipse(200, 200, 100, 100); // Draws an ellipse at (200, 200) with width and height of 100 fill(0, 0, 255); // Blue fill color triangle(300, 300, 350, 250, 350, 350); // Draws a triangle with specified vertices }
Animation and Interaction
One of the most exciting aspects of p5.js is its ability to create dynamic, interactive sketches. By updating variables within the draw
function, you can create animations. Additionally, p5.js provides functions to handle user input, such as mouse clicks and key presses.
Simple Animation
Here's a simple example where a circle moves across the canvas:
let x = 0; function setup() { createCanvas(400, 400); } function draw() { background(220); fill(0); ellipse(x, height / 2, 50, 50); // Draws a circle at (x, height/2) x += 2; // Increases the x position by 2 each frame }
Interactive Sketch
Now, let's make our sketch interactive by changing the circle's color when the mouse is pressed:
let x = 0; let circleColor = 0; function setup() { createCanvas(400, 400); } function draw() { background(220); fill(circleColor); ellipse(x, height / 2, 50, 50); x += 2; if (x > width) { x = 0; } } function mousePressed() { circleColor = color(random(255), random(255), random(255)); // Changes color to a random color }
Advanced Features
As you grow more comfortable with p5.js, you can explore its advanced features like 3D graphics, sound, and integrating other libraries. Whether you're creating generative art, interactive installations, or educational tools, p5.js has something to offer.
Conclusion
p5.js opens up a world of possibilities for creative coding. From simple drawings to complex animations and interactive sketches, it provides a fun and accessible way to bring your ideas to life. So, grab your digital paintbrush and start creating!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
Can I use p5.js with other JavaScript libraries?
Yes, p5.js can be used alongside other JavaScript libraries like jQuery, Three.js, and D3.js. Just make sure to include them properly in your HTML file.
Is p5.js suitable for beginners?
Absolutely! p5.js is designed to be beginner-friendly, with a straightforward API and plenty of resources to help you get started. It's a great way to learn programming through creative projects.
Can I create 3D graphics with p5.js?
Yes, p5.js supports 3D graphics. You can use functions like createCanvas
with the WEBGL
parameter to create a 3D canvas and start drawing 3D shapes.
How can I debug my p5.js sketches?
You can use the browser's developer tools to debug your p5.js sketches. Console logging with console.log
is also very useful for checking variable values and program flow.
Where can I find more resources to learn p5.js?
The p5.js website has extensive documentation, tutorials, and examples. Additionally, there are numerous online courses, forums, and communities dedicated to p5.js that you can explore.