Exploring p5.js Features
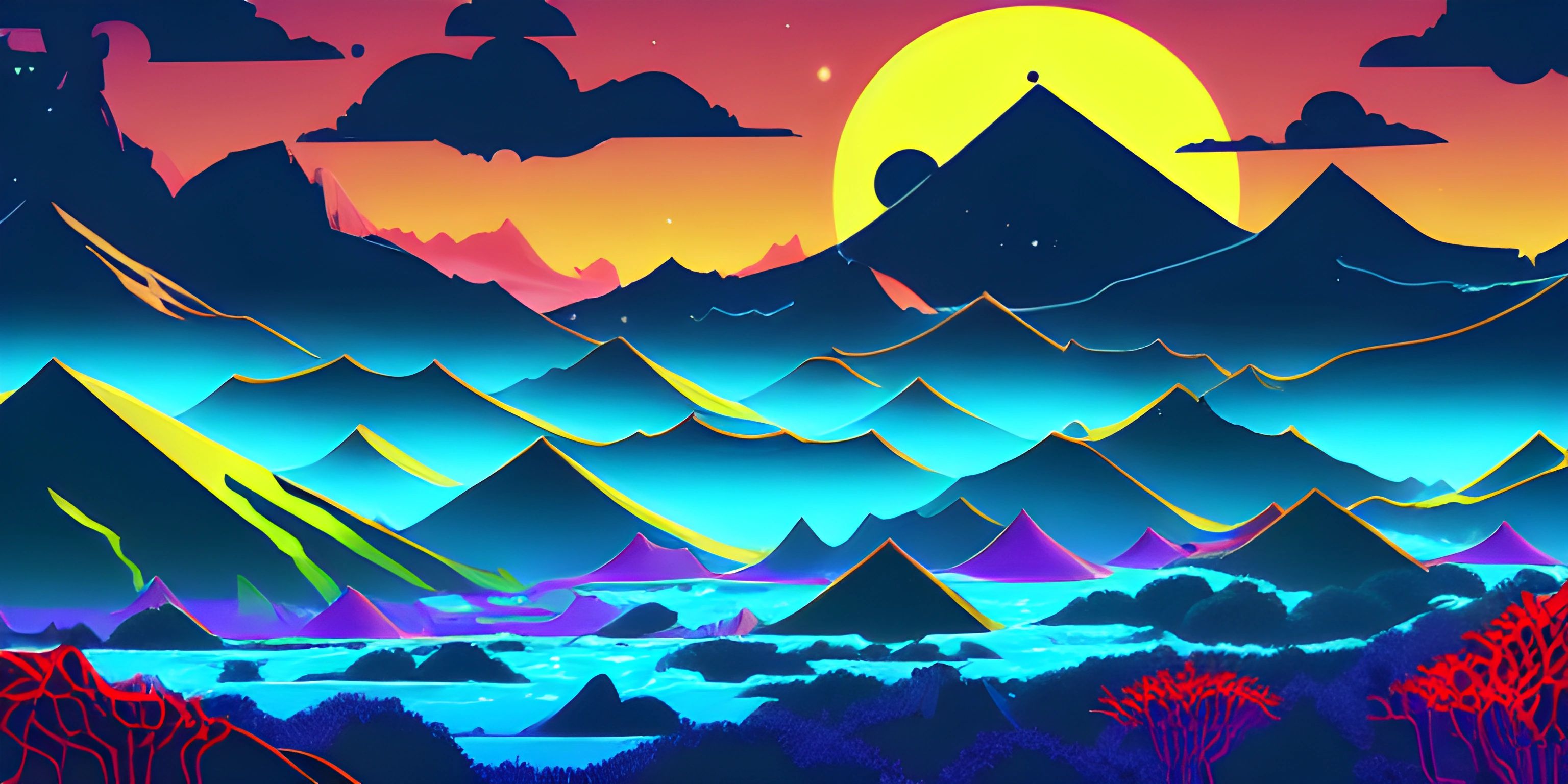
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're an artist, but instead of a brush and canvas, your tools are a keyboard and a screen. Welcome to the world of p5.js, a JavaScript library that's like a playground for creative coding! Whether you're interested in making animations, visualizations, or interactive art, p5.js has got your back. Let's dive into some of the coolest features it offers and how you can use them to unleash your inner digital artist.
Getting Started with p5.js
Before we jump into the features, let's get p5.js set up. You'll need a basic HTML file to see your creations come to life. Here's a starter template:
<!DOCTYPE html> <html> <head> <title>p5.js Sketch</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> </head> <body> <script> function setup() { createCanvas(400, 400); // Create a 400x400 pixel canvas } function draw() { background(220); // Set background color to light grey ellipse(200, 200, 50, 50); // Draw a circle at (200, 200) with a diameter of 50 } </script> </body> </html>
This template sets up a basic p5.js sketch with a canvas and a circle. Easy peasy, right?
Drawing Shapes
One of the most fundamental features of p5.js is its ability to draw shapes. From simple circles to complex polygons, you can create a wide array of shapes with just a few lines of code.
Basic Shapes
Let's start by drawing basic shapes like rectangles and ellipses:
function setup() { createCanvas(400, 400); } function draw() { background(220); rect(50, 50, 100, 150); // Draw a rectangle at (50, 50) with width 100 and height 150 ellipse(300, 200, 80, 80); // Draw a circle at (300, 200) with a diameter of 80 }
In this example, rect
and ellipse
are used to draw a rectangle and a circle, respectively. But p5.js doesn't stop there. You can also draw triangles, lines, and even custom shapes.
Custom Shapes
To draw custom shapes, use the beginShape
and endShape
functions:
function setup() { createCanvas(400, 400); } function draw() { background(220); beginShape(); vertex(100, 100); // First point vertex(150, 50); // Second point vertex(200, 100); // Third point vertex(150, 150); // Fourth point endShape(CLOSE); // Close the shape }
This code creates a diamond shape by connecting vertices.
Colors and Styling
What's art without color? p5.js makes it super easy to add colors and styles to your shapes.
Colors
You can set the color of your shapes using the fill
and stroke
functions:
function setup() { createCanvas(400, 400); } function draw() { background(220); fill(255, 0, 0); // Set fill color to red stroke(0, 0, 255); // Set stroke color to blue rect(50, 50, 100, 150); }
In this example, the rectangle will have a red fill and a blue outline.
Gradients
For more advanced coloring, you can create gradients by interpolating colors:
function setup() { createCanvas(400, 400); } function draw() { for (let i = 0; i < height; i++) { let inter = map(i, 0, height, 0, 1); let c = lerpColor(color(255, 0, 0), color(0, 0, 255), inter); stroke(c); line(0, i, width, i); } }
This creates a gradient from red to blue from the top to the bottom of the canvas.
Animations
One of the most captivating features of p5.js is its ability to create animations. Since draw
runs in a loop, you can update your shapes' positions to animate them.
Bouncing Ball
Let's create a simple bouncing ball animation:
let x = 200; let y = 200; let xspeed = 2; let yspeed = 3; function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(x, y, 50, 50); x += xspeed; y += yspeed; if (x > width - 25 || x < 25) { xspeed *= -1; // Reverse direction } if (y > height - 25 || y < 25) { yspeed *= -1; // Reverse direction } }
This code makes a ball bounce around the canvas, reversing direction when it hits the edges.
Interactivity
p5.js also excels in making your sketches interactive. You can use mouse and keyboard inputs to control your drawings.
Mouse Interaction
Let's make a circle follow the mouse:
function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(mouseX, mouseY, 50, 50); // Draw circle at mouse position }
Keyboard Interaction
You can also use keyboard inputs to move shapes around:
let x = 200; let y = 200; function setup() { createCanvas(400, 400); } function draw() { background(220); ellipse(x, y, 50, 50); } function keyPressed() { if (keyCode === LEFT_ARROW) { x -= 10; } else if (keyCode === RIGHT_ARROW) { x += 10; } else if (keyCode === UP_ARROW) { y -= 10; } else if (keyCode === DOWN_ARROW) { y += 10; } }
In this sketch, the circle moves based on arrow key presses.
Text and Fonts
Adding text to your sketches can be a game-changer. p5.js supports various fonts and text styles.
Basic Text
Here's how to add basic text to your canvas:
function setup() { createCanvas(400, 400); } function draw() { background(220); textSize(32); fill(0); text("Hello, p5.js!", 50, 200); }
Custom Fonts
To use custom fonts, you need to load them first:
<!DOCTYPE html> <html> <head> <title>p5.js Custom Font</title> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> </head> <body> <script> let font; function preload() { font = loadFont('your-font-file.ttf'); // Make sure to replace with your font file } function setup() { createCanvas(400, 400); textFont(font); } function draw() { background(220); textSize(32); fill(0); text("Custom Font!", 50, 200); } </script> </body> </html>
Conclusion
p5.js is a powerful tool for creative coding that makes it easy to draw shapes, add colors, create animations, and interact with your sketches. Whether you're a seasoned coder or a beginner, p5.js opens up a world of artistic possibilities.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
How do I set up p5.js?
You can set up p5.js by including the p5.js library in a basic HTML file. Use the <script>
tag to link to the p5.js CDN and then write your JavaScript code within another <script>
tag.
Can I use p5.js with other JavaScript libraries?
Yes, p5.js can be used alongside other JavaScript libraries. Just make sure to manage your script loading order to avoid conflicts.
How do I draw custom shapes in p5.js?
You can draw custom shapes in p5.js using the beginShape
and endShape
functions, and add points with the vertex
function.
What are some common uses for p5.js?
p5.js is commonly used for creating visual art, animations, educational tools, and interactive installations. It's a go-to library for creative coding.
Can I create 3D graphics with p5.js?
Yes, p5.js supports 3D graphics. You can use the WEBGL
renderer to create and manipulate 3D shapes and scenes.