Problem Solving Strategies
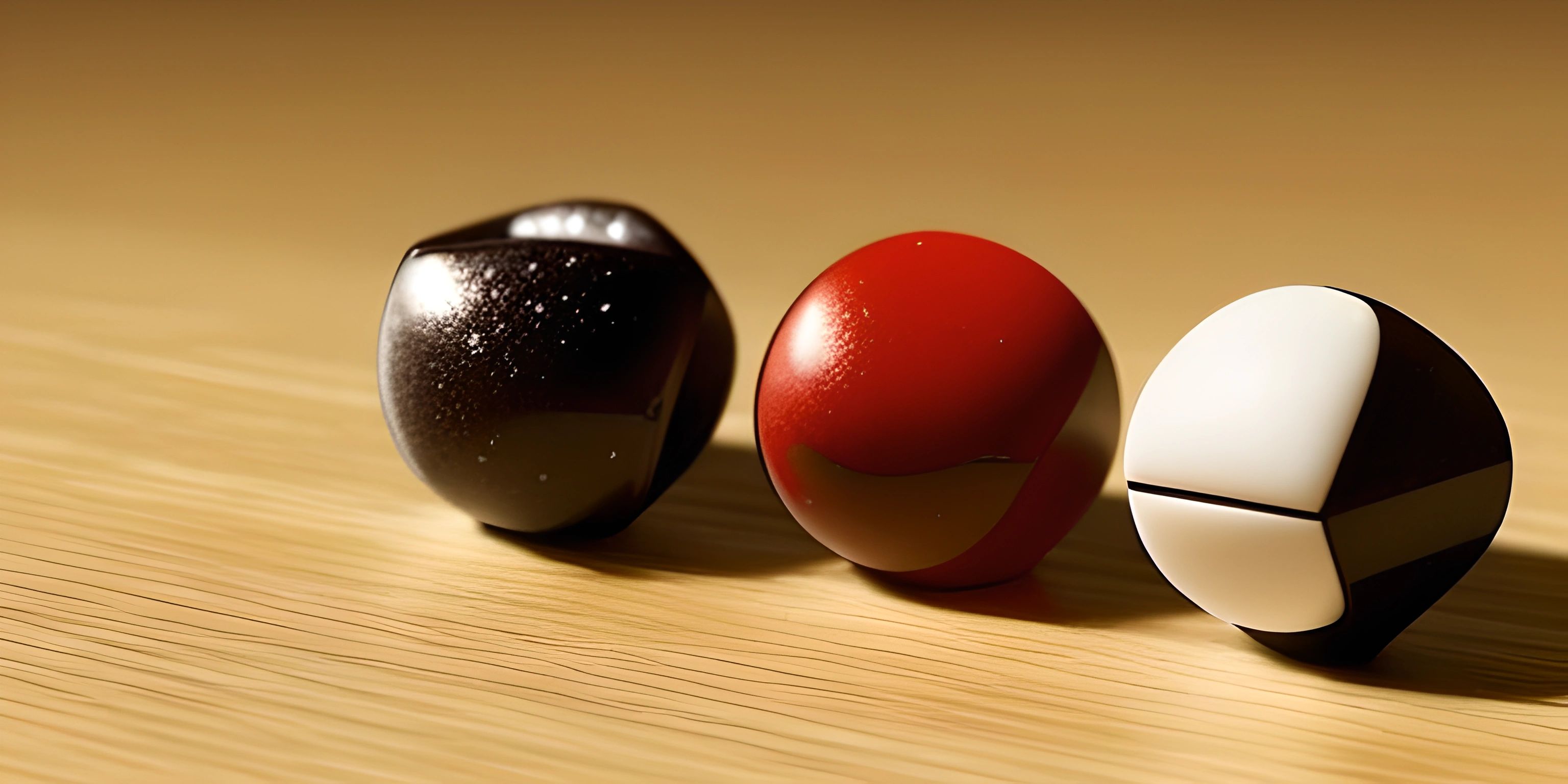
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Breaking down coding challenges and solving them step by step is an essential skill for any programmer. In this article, we will discuss some problem-solving strategies that can help you tackle coding problems more effectively.
Understand the Problem
Before diving into writing code, it's crucial to have a clear understanding of the problem. Read the problem statement carefully and try to identify the following:
- What are the inputs?
- What are the expected outputs?
- Are there any constraints or limitations?
Once you have a good understanding of the problem, it's helpful to write down a brief summary in your own words. This will help solidify your understanding and make it easier to explain the problem to others if you need assistance.
Break the Problem into Smaller Parts
Divide the problem into smaller, manageable parts. This will help you focus on individual aspects of the problem and make it easier to solve. For example, if you're building a web application, break the problem down into smaller tasks such as creating the user interface, handling user input, and processing data.
Write Pseudocode
Before writing actual code, it's helpful to write pseudocode, a high-level description of your solution using plain English. Pseudocode helps you focus on the logic of your solution without getting bogged down in syntax.
Here's an example of pseudocode for a function that calculates the factorial of a number:
function factorial(n) if n is 0 or 1 return 1 else return n * factorial(n - 1)
Write Code and Test
Now that you have a clear understanding of the problem and a plan for your solution, it's time to write the code. Start by writing code for each smaller part you identified earlier. Test each part individually to ensure it works as expected. This will help you catch errors early and make it easier to debug your code.
Review and Optimize
Once your code is working, take some time to review it for readability and efficiency. Are there any parts of your code that could be written more clearly or more efficiently? Are there any edge cases you haven't considered? Make any necessary changes to optimize your code and ensure it's easy to understand and maintain.
Practice Regularly
The more you practice problem-solving, the better you'll become at it. Regularly tackle coding challenges and problems to sharpen your skills and become a more effective problem solver.
By following these strategies, you'll be better equipped to break down coding challenges and solve them step by step. Remember to understand the problem, break it into smaller parts, write pseudocode, write and test your code, and review and optimize your solution. And most importantly, practice regularly to hone your problem-solving skills.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
What are some common problem-solving strategies in coding?
Some common problem-solving strategies in coding include:
- Understanding the problem: Read the problem statement carefully and identify the inputs, outputs, and constraints.
- Breaking the problem down: Divide the problem into smaller, manageable tasks or subproblems.
- Designing an algorithm: Create a step by step process to solve the problem, keeping in mind the language and data structures you'll be using.
- Implementing the solution: Write clean, efficient, and well-documented code to implement your algorithm.
- Testing and debugging: Test your code with various input cases, and debug any issues that arise.
How can I effectively break down a coding problem into smaller tasks?
To break down a coding problem into smaller tasks, first identify the main components of the problem, like input processing, calculations, and output formatting. Next, tackle each component one by one, focusing on the logic and data structures needed for each task. This approach not only helps you understand the problem better but also simplifies the implementation process.
What should I keep in mind while designing an algorithm for a coding problem?
When designing an algorithm for a coding problem, consider the following:
- Understand the problem requirements and constraints.
- Choose appropriate data structures and algorithms that fit the problem.
- Think about the time and space complexity of your solution.
- Ensure your algorithm covers edge cases and handles invalid inputs.
How can I improve my problem-solving skills in coding?
To improve your problem-solving skills in coding:
- Practice regularly by solving coding challenges on platforms like LeetCode, HackerRank, or Codecademy.
- Read and analyze other people's code to learn different approaches and techniques.
- Participate in coding competitions or hackathons to challenge yourself.
- Learn new programming languages, data structures, and algorithms to expand your knowledge base.
How do I know if my code is efficient and optimized?
To determine if your code is efficient and optimized, analyze the time and space complexity of your solution. An optimized solution should have a relatively low complexity, meaning it can handle large input sizes without significant performance degradation. Additionally, review your code for any repetitive or unnecessary calculations, and consider alternative data structures or algorithms that could improve the efficiency of your solution.