Building Interactive Sketches with py5
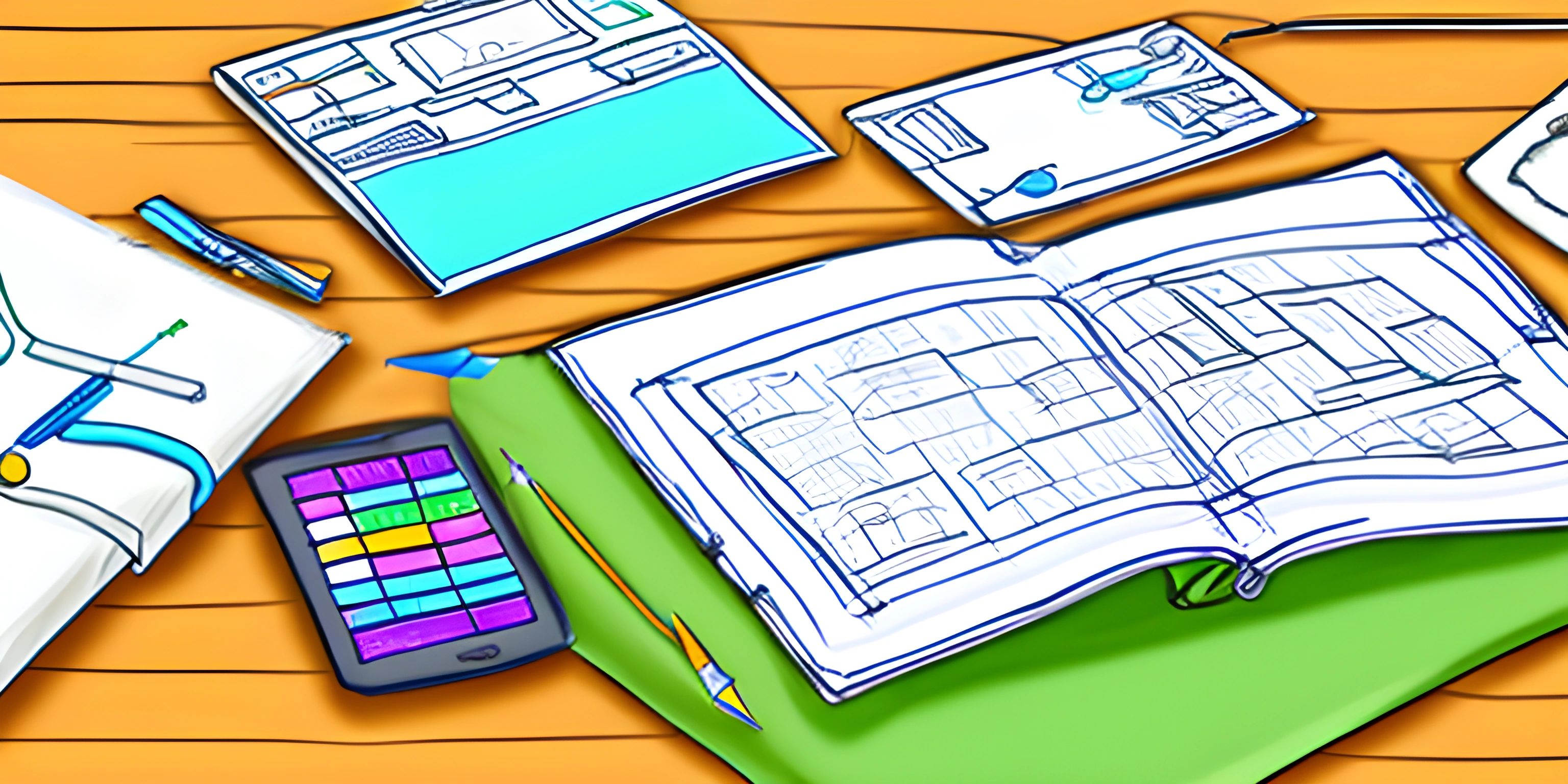
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Let's dive into the wonderful world of creating interactive sketches with py5. Imagine your screen as a canvas, and you as the artist, wielding the mighty brush of code. With py5, you can create dynamic and interactive sketches right in Python!
Getting Started
First things first, install py5. Open your terminal and run:
pip install py5
Once it's installed, you're ready to start sketching!
Setting Up the Sketch
A py5 sketch needs a setup
and a draw
function. Think of setup
as your sketch's preparation phase and draw
as its action phase. In setup
, you define your canvas size and other initial settings. In draw
, you bring your canvas to life, and it runs continuously in a loop.
import py5 def setup(): py5.size(800, 600) # Set canvas size to 800x600 py5.background(255) # Fill the background with white def draw(): py5.ellipse(py5.mouse_x, py5.mouse_y, 50, 50) # Draw an ellipse following the mouse py5.run_sketch()
In this example, we set up an 800x600 canvas and draw an ellipse that follows the mouse cursor.
Adding Interactivity
To make your sketches interactive, you can use mouse and keyboard events. Let's make some magic happen when the mouse is pressed and when keys are pressed!
Mouse Interaction
You can create functions like mouse_pressed
, mouse_released
, and mouse_dragged
to handle mouse events.
def mouse_pressed(): py5.fill(0, 0, 255) # Change fill color to blue when the mouse is pressed def mouse_released(): py5.fill(255, 0, 0) # Change fill color to red when the mouse is released def draw(): py5.ellipse(py5.mouse_x, py5.mouse_y, 50, 50) # Draw ellipse py5.run_sketch()
In this example, the ellipse changes color based on mouse actions. When the mouse is pressed, it turns blue, and when released, it turns red.
Keyboard Interaction
Similarly, you can handle keyboard events with functions like key_pressed
, key_released
, and key_typed
.
def key_pressed(): if py5.key == 'r': py5.background(255, 0, 0) # Red background for 'r' key if py5.key == 'g': py5.background(0, 255, 0) # Green background for 'g' key if py5.key == 'b': py5.background(0, 0, 255) # Blue background for 'b' key py5.run_sketch()
In this example, the background color changes based on the key pressed ('r' for red, 'g' for green, 'b' for blue).
Combining Mouse and Keyboard Interactions
Now, let's combine both mouse and keyboard interactions to create a more complex interactive sketch.
def setup(): py5.size(800, 600) py5.background(255) def draw(): py5.ellipse(py5.mouse_x, py5.mouse_y, 50, 50) def mouse_pressed(): py5.fill(0, 0, 255) # Blue fill on mouse press def mouse_released(): py5.fill(255, 0, 0) # Red fill on mouse release def key_pressed(): if py5.key == 'r': py5.background(255, 0, 0) # Red background for 'r' key if py5.key == 'g': py5.background(0, 255, 0) # Green background for 'g' key if py5.key == 'b': py5.background(0, 0, 255) # Blue background for 'b' key py5.run_sketch()
Here, we maintain the ellipse color-changing behavior with mouse actions and change the background color with keyboard actions.
Advanced Interaction: Creating a Simple Game
Let's create a simple game where the player controls a circle with the mouse and collects falling squares. We'll use both mouse and keyboard interactions to make it engaging.
import random # Global variables player_x = 400 player_y = 300 player_radius = 25 squares = [] score = 0 def setup(): py5.size(800, 600) py5.background(255) py5.no_stroke() py5.fill(0, 0, 0) # Black fill for squares def draw(): global score py5.background(255) # Draw and move player (circle) player_x, player_y = py5.mouse_x, py5.mouse_y py5.fill(0, 0, 255) py5.ellipse(player_x, player_y, player_radius * 2, player_radius * 2) # Create and move squares if py5.frame_count % 60 == 0: # Create a square every second squares.append([random.randint(0, py5.width), 0, 20, 20]) for square in squares: square[1] += 5 # Move square down py5.rect(square[0], square[1], square[2], square[3]) # Collision detection if (player_x - player_radius < square[0] < player_x + player_radius and player_y - player_radius < square[1] < player_y + player_radius): squares.remove(square) score += 1 # Display score py5.fill(0) py5.text_size(32) py5.text(f"Score: {score}", 10, 30) def key_pressed(): # Reset game global squares, score if py5.key == 'r': squares = [] score = 0 py5.run_sketch()
In this game, the player moves a circle with the mouse to collect falling squares, earning points for each collection. Press 'r' to reset the game.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is py5?
py5 is a Python library that provides an interface to the Processing visualization framework, allowing users to create visual and interactive sketches using Python.
How do I install py5?
You can install py5 using pip. Open your terminal and run the command pip install py5
.
Can I handle both mouse and keyboard interactions in a py5 sketch?
Yes, py5 supports mouse and keyboard interactions through functions like mouse_pressed
, mouse_released
, key_pressed
, and key_released
.
How do I reset the sketch or game in py5?
You can reset the sketch or game by defining a key_pressed
function that clears the necessary variables or resets the state. For example, pressing 'r' can reset the game state.
What are some use cases for py5?
py5 can be used for creating interactive visualizations, educational tools, simple games, data art, and more. Its versatility makes it suitable for a wide range of creative coding projects.