Pytest Introduction
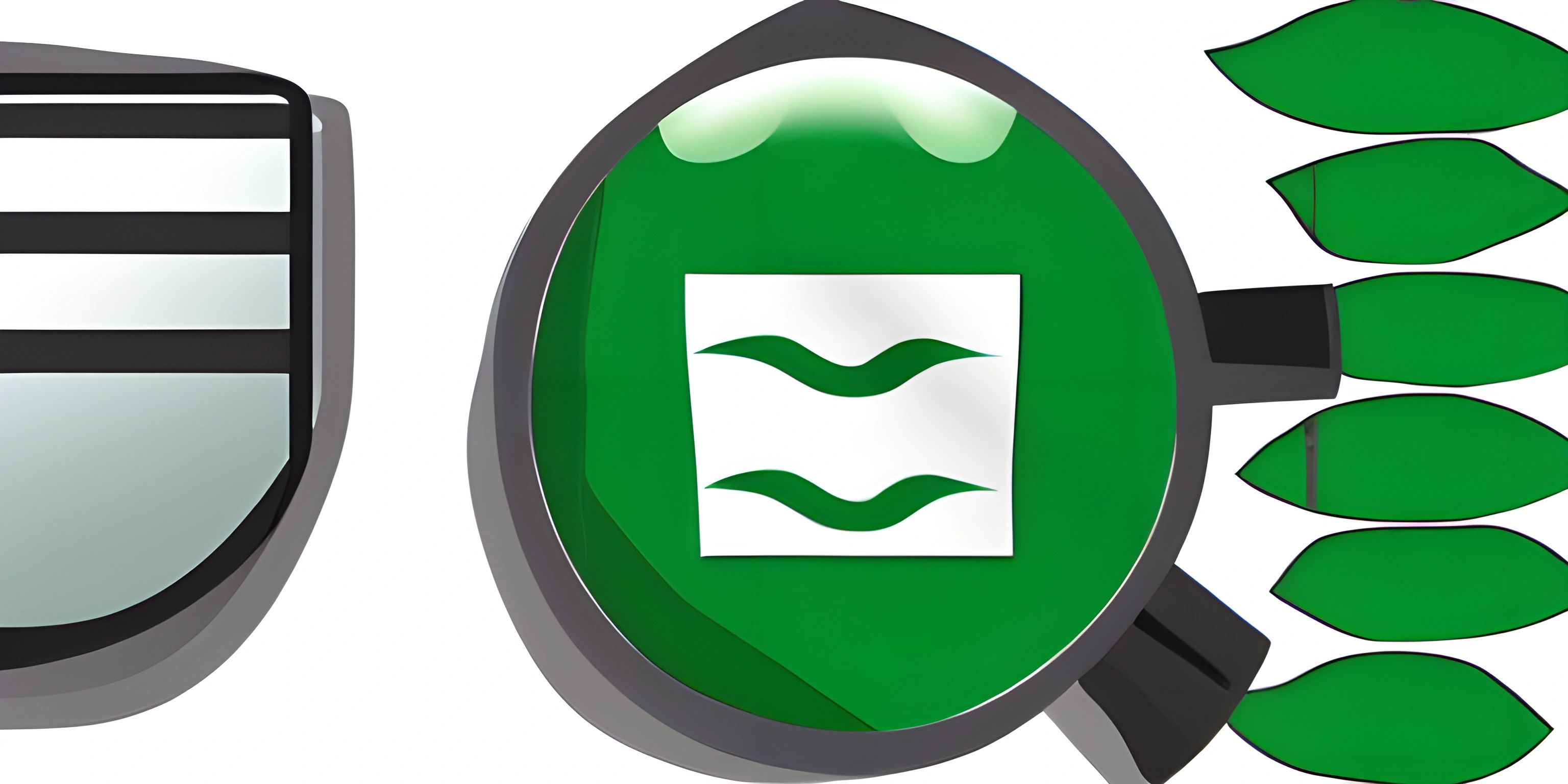
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to writing code, ensuring its reliability and robustness is crucial. One way to achieve this is by using testing frameworks. In the world of Python, pytest is a popular and powerful testing framework that makes writing and organizing tests a breeze.
What is pytest?
Pytest is an open-source testing framework for Python that allows developers to write simple, scalable, and maintainable test cases. It boasts a rich ecosystem of plugins and integrations, making it an excellent choice for projects of various sizes and complexities.
The pytest framework promotes a clean and modular approach to writing tests, which makes it easy to understand and debug your code when necessary. Whether you're working on a small script or a large-scale application, pytest can handle it all.
Installing pytest
Before diving into pytest, you need to install it. The easiest way to do this is by using pip
, the Python package installer. Simply run the following command in your terminal:
pip install pytest
Now that pytest is installed, we can start writing tests!
Writing your first pytest test
Let's create a simple function that we want to test. Create a file named greetings.py
and add the following code:
def greet(name): return f"Hello, {name}!"
Now let's write a test for this function. Create a new file named test_greetings.py
in the same directory as greetings.py
. In this file, write the following code:
import pytest from greetings import greet def test_greet(): assert greet("John") == "Hello, John!"
The test_greet
function is our test case. It's a simple function that checks whether the output of greet("John")
is equal to the expected string.
To run this test, open up a terminal, navigate to the directory containing both files, and type:
pytest
Pytest will automatically discover and run tests in your project. You should see output indicating that the test passed.
Key pytest features
One of pytest's strengths is its concise and expressive syntax. It allows you to write powerful assertions using the assert
keyword, making your tests easy to read and understand.
Another advantage of pytest is its ability to generate detailed reports on test failures, helping you investigate and resolve issues in your code.
Finally, pytest is extremely extensible through its vast ecosystem of plugins. You can find plugins for almost any requirement, from parallel test execution to integrating with various web development frameworks.
Conclusion
Pytest is a versatile and powerful testing framework for Python, making it an excellent choice for projects of all sizes. Its simple syntax, detailed reports, and rich plugin ecosystem make it a favorite among Python developers. Start using pytest in your projects today, and ensure your code stays reliable and robust.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: AI Test Playground (psst, it's free!).
FAQ
What is pytest?
Pytest is an open-source testing framework for Python that allows developers to write simple, scalable, and maintainable test cases. It has a clean and modular approach to writing tests, making it easy to understand and debug your code when necessary.
How do I install pytest?
You can install pytest using pip, the Python package installer, by running the following command in your terminal: pip install pytest
.
How do I write a simple test case using pytest?
To write a simple pytest test case, start by creating a new Python file with a name starting with test_
, e.g., test_greetings.py
. Inside this file, write a function that starts with test_
, import the function or module you want to test, and use the assert
keyword to check if the expected output matches the actual output.
How do I run pytest tests?
To run pytest tests, open a terminal, navigate to the directory containing your test files, and type pytest
. Pytest will automatically discover and run tests in your project.