Introduction to Jest Testing Framework
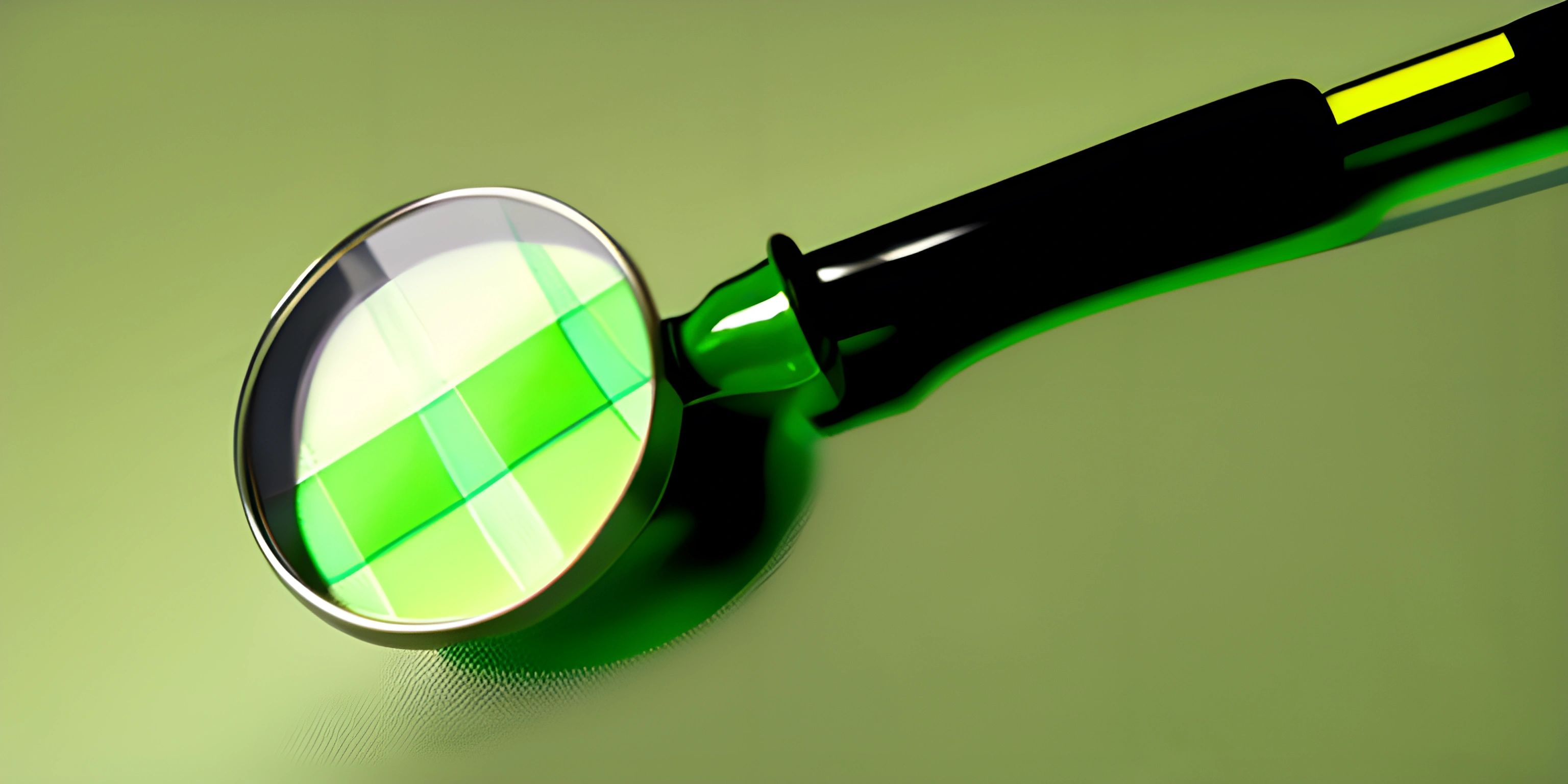
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Jest is a popular, open-source testing framework for JavaScript created by Facebook. With its zero configuration, ease of use, and extensive features, Jest has become the go-to testing solution for many developers. This article gives you an overview of Jest and shows you how to set it up and write your first tests.
Why Use Jest?
Jest offers several benefits, including:
- Fast and efficient: Jest runs tests in parallel, optimizing for performance.
- Snapshot testing: Easily capture and compare snapshots of your application's output.
- Mocking: Jest provides built-in mocking capabilities, making it simple to test complex logic.
- Code coverage: Track how much of your code is covered by tests, and easily identify areas that need more testing.
- Watch mode: Run your tests as you code, automatically running relevant tests as you make changes.
Setting Up Jest
To start using Jest, you need to have Node.js installed on your system. Once Node.js is set up, follow these steps to install Jest:
- Create a new project: Create a new directory for your project and navigate to it in the terminal.
mkdir jest-introduction cd jest-introduction
- Initialize npm: Run
npm init
to create apackage.json
file. You can use the-y
flag to accept the default settings.
npm init -y
- Install Jest: Install Jest as a development dependency by running the following command:
npm install --save-dev jest
Writing Your First Test
Now that Jest is installed, let's write a simple test. First, create a file named sum.js
in your project directory with the following content:
function sum(a, b) { return a + b; } module.exports = sum;
Next, create a file named sum.test.js
in the same directory with the following code:
const sum = require("./sum"); test("sums two numbers", () => { expect(sum(1, 2)).toBe(3); });
In your package.json
, update the test
script to run Jest:
"scripts": { "test": "jest" }
Now, run your test using the following command:
npm test
You should see output indicating that your test has passed.
Additional Resources
As you dive deeper into Jest, you may find the following resources helpful:
- Official Jest Documentation
- Testing React with Jest and Enzyme
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Jest?
Jest is a popular, open-source testing framework for JavaScript developed by Facebook. It offers features such as snapshot testing, mocking, code coverage, and watch mode, making it a go-to solution for many developers.
How do I set up Jest?
To set up Jest, first make sure you have Node.js installed. Then, create a new project, initialize npm, and install Jest as a development dependency using the command npm install --save-dev jest
.
How do I write a test using Jest?
To write a test using Jest, create a test file with a .test.js
extension in your project directory. Import the code you want to test, and use the test
function provided by Jest to define your tests. Inside the test function, use expect
and Jest's built-in matchers to assert the expected behavior of your code.