Python Control Flow
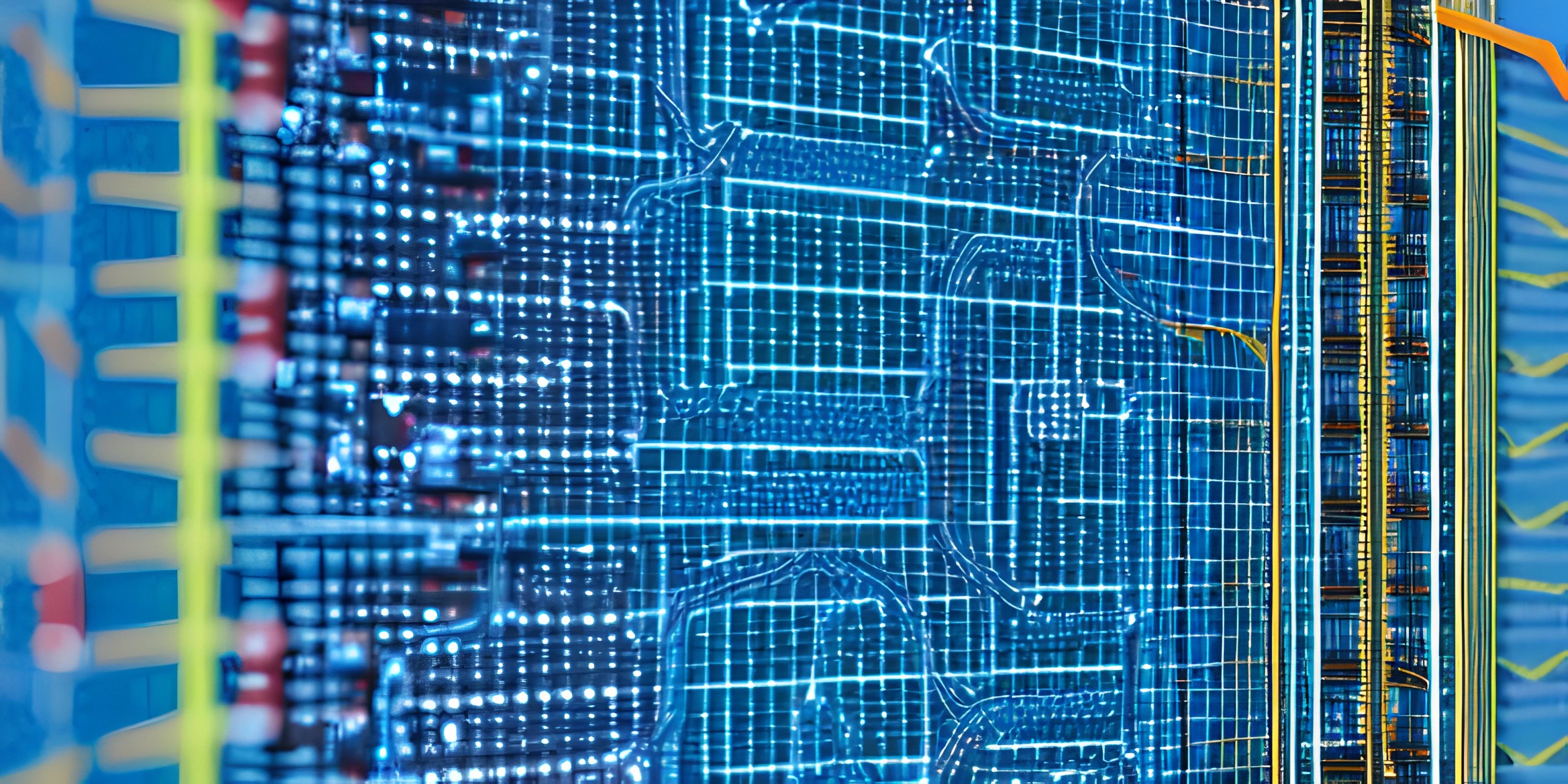
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Control flow is what makes a program dynamic and capable of handling different situations. In Python, the primary way to create control flow is through the use of if
, elif
, and else
statements. These statements allow you to execute specific blocks of code based on conditions. Let's dive into each of them and see how they work together.
If Statements
An if
statement is used to test a condition, and if that condition is True
, the block of code indented under the if
statement is executed. Here's a basic example:
age = 18 if age >= 18: print("You are an adult!")
In this example, the condition age >= 18
is True
, so the print
statement inside the if
block is executed, and "You are an adult!" is printed.
Else Statements
An else
statement is used to catch any other cases not handled by the if
statement. It is optional and should be placed after the if
statement. Here's an example of using else
:
age = 16 if age >= 18: print("You are an adult!") else: print("You are not an adult yet.")
In this example, the condition age >= 18
is False
, so the code inside the else
block is executed, printing "You are not an adult yet."
Elif Statements
An elif
(short for "else if") statement is used to test multiple conditions in a sequence. If the if
condition is False
, the program checks the next elif
condition, and so on. If none of the conditions are True
, the code in the else
block (if present) is executed. Here's an example using if
, elif
, and else
:
age = 12 if age >= 18: print("You are an adult!") elif age >= 13: print("You are a teenager!") else: print("You are a child!")
In this example, since age = 12
, the if
condition is False
, so the program moves on to check the elif
condition, which is also False
. Finally, it moves to the else
block, printing "You are a child!"
Combining Conditions
You can use logical operators like and
, or
, and not
to combine conditions in if
, elif
, and else
statements. For example:
age = 17 has_driver_license = True if age >= 18 and has_driver_license: print("You can drive legally!") else: print("You cannot drive legally yet.")
In this example, the condition age >= 18 and has_driver_license
is False
, so the else
block is executed, printing "You cannot drive legally yet."
Remember that proper indentation is crucial in Python control flow statements, as it determines the scope of the code blocks.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the basic control flow statements in Python?
The basic control flow statements in Python are if
, elif
, and else
. if
is used to test a condition, elif
is used for additional conditions, and else
is used to catch any remaining cases when all previous conditions are False
.
How do you combine conditions in control flow statements?
You can combine conditions in control flow statements using logical operators like and
, or
, and not
. For example, if age >= 18 and has_driver_license:
would check if both conditions are True
.
Why is indentation important in Python control flow statements?
Indentation is important in Python control flow statements because it determines the scope of the code blocks. Proper indentation ensures that the code inside the if
, elif
, or else
blocks is executed only when the corresponding conditions are met.