Conditionals in Programming
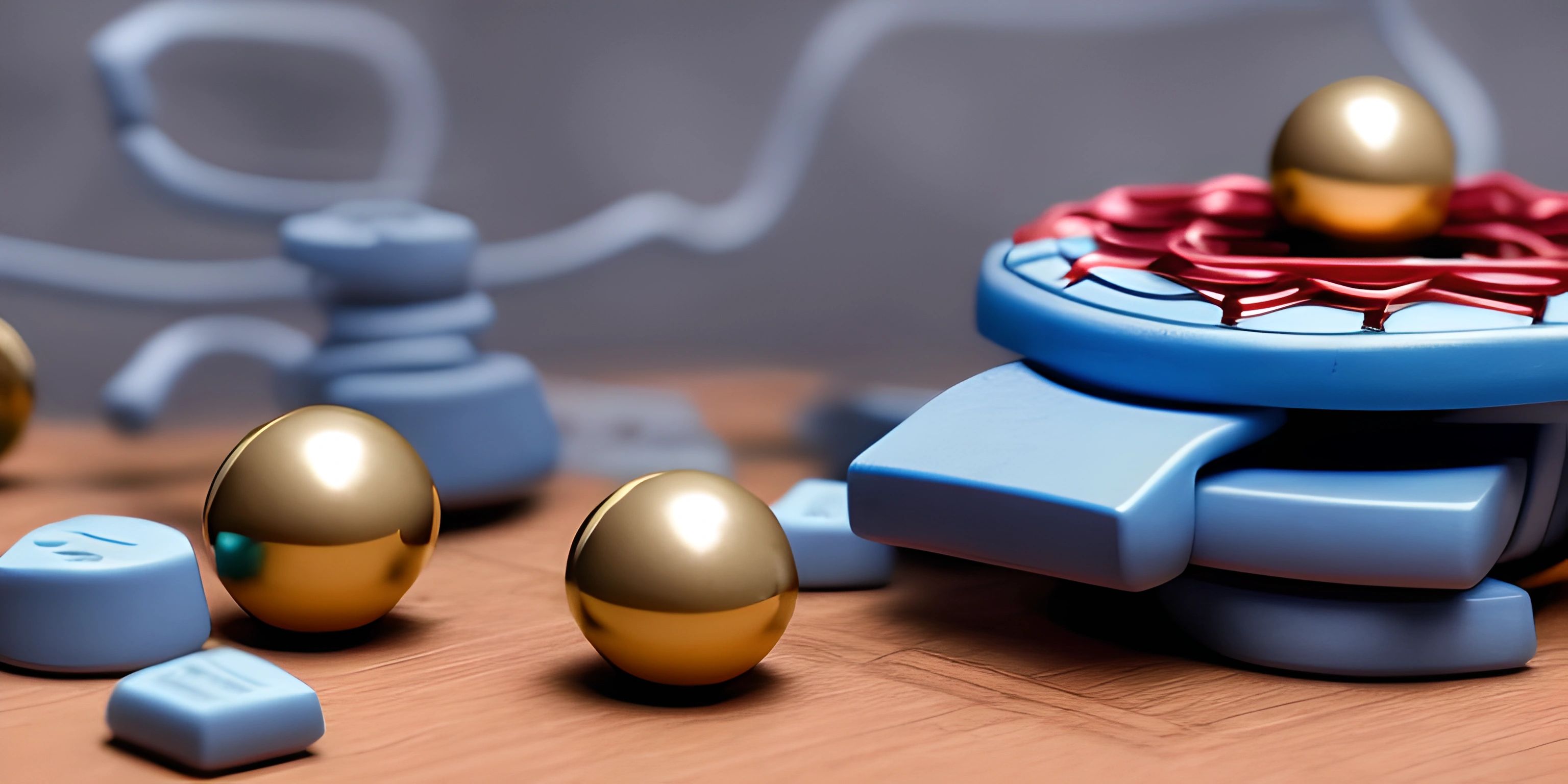
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Conditionals are the bread and butter of decision-making in programming. They allow your program to make choices based on certain conditions, ultimately leading to more complex and dynamic behavior. Let's dive into the world of conditionals and see what they're all about.
The If Statement
The most basic conditional is the if
statement. It checks if a condition is true and then executes the code inside the block. Consider this simple example:
temperature = 75 if temperature > 70: print("It's warm outside!")
In this example, the program checks if the temperature
variable is greater than 70. If it is, it prints "It's warm outside!". Since the temperature is 75, the condition is true, and the message will be printed.
The Else Statement
Now, what if we want to print a different message when it's not warm outside? That's where the else
statement comes in. It's like the other side of the coin – it executes the code inside the block when the if
condition is false.
Here's an example:
temperature = 65 if temperature > 70: print("It's warm outside!") else: print("It's not warm outside.")
In this case, the temperature is 65, so the if
condition is false. The program skips the first block and executes the else
block, printing "It's not warm outside."
The Elif Statement
Sometimes we have more than two choices to make. That's when we can use the elif
(short for "else if") statement. It checks for additional conditions when the previous if
or elif
conditions are false.
Let's extend our temperature example:
temperature = 45 if temperature > 70: print("It's warm outside!") elif temperature > 50: print("It's cool outside.") else: print("It's cold outside.")
Now, with the temperature at 45, the program checks the if
statement (false), moves to the elif
statement (also false), and finally reaches the else
statement. It prints "It's cold outside."
Switch Cases
Some programming languages, like JavaScript, offer a switch
statement as an alternative to multiple if
, elif
, and else
statements. A switch
statement compares a value against various cases and executes the code inside the matching case block.
Here's a switch
example in JavaScript:
let day = 3; switch (day) { case 1: console.log("Monday"); break; case 2: console.log("Tuesday"); break; case 3: console.log("Wednesday"); break; // ...other cases for the rest of the week default: console.log("Invalid day."); }
In this example, the program checks the day
variable against each case. When it finds a matching case (in this case, day
is 3, so it matches the third case), it executes the code inside and then breaks out of the switch
statement.
Conclusion
Conditionals are indispensable tools in programming for making decisions based on specific conditions. Mastering if
, else
, elif
, and switch
statements will enable you to create more dynamic and versatile programs. Now, go ahead and explore the power of conditionals in your code!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using Constants (psst, it's free!).
FAQ
What are conditionals in programming and why are they important?
Conditionals are statements in programming that allow the flow of a program to be controlled based on certain conditions. They are crucial because they enable a program to make decisions, execute different pieces of code, and respond to various inputs or scenarios. Without conditionals, programs would be limited to executing the same series of actions every time, regardless of the input or circumstances.
How do I use basic conditional statements in programming?
Basic conditional statements can be written using the if
, else
, and elif
(else if) keywords. Here's an example in Python:
temperature = 35 if temperature > 30: print("It's hot outside!") elif temperature > 20: print("It's comfortably warm.") else: print("It's cold outside!")
In this example, the program decides which message to print based on the value of the temperature
variable.
What are some common comparison operators used in conditionals?
Common comparison operators used in conditionals include:
==
: Equal to!=
: Not equal to<
: Less than>
: Greater than<=
: Less than or equal to>=
: Greater than or equal to These operators allow you to compare values and create expressions that evaluate to a boolean value (True or False) to be used in conditional statements.
Can I combine multiple conditions in a single conditional statement?
Yes, you can combine multiple conditions using logical operators like and
, or
, and not
. Here's an example in Python that demonstrates the use of multiple conditions:
age = 25 has_license = True if age >= 18 and has_license: print("You are allowed to drive.") else: print("You are not allowed to drive.")
In this example, the program checks if the age
is greater than or equal to 18 and if the person has a license before allowing them to drive.
What is a ternary conditional expression?
A ternary conditional expression, also known as a conditional operator or inline if, is a shorthand way of writing a simple if-else
statement in a single line. It allows you to assign a value to a variable based on a condition. Here's an example in Python:
score = 85 result = "Passed" if score >= 60 else "Failed"
In this example, the value of result
will be "Passed" if the score
is greater than or equal to 60, otherwise, it will be "Failed".