Mastering Conditional Statements in Java
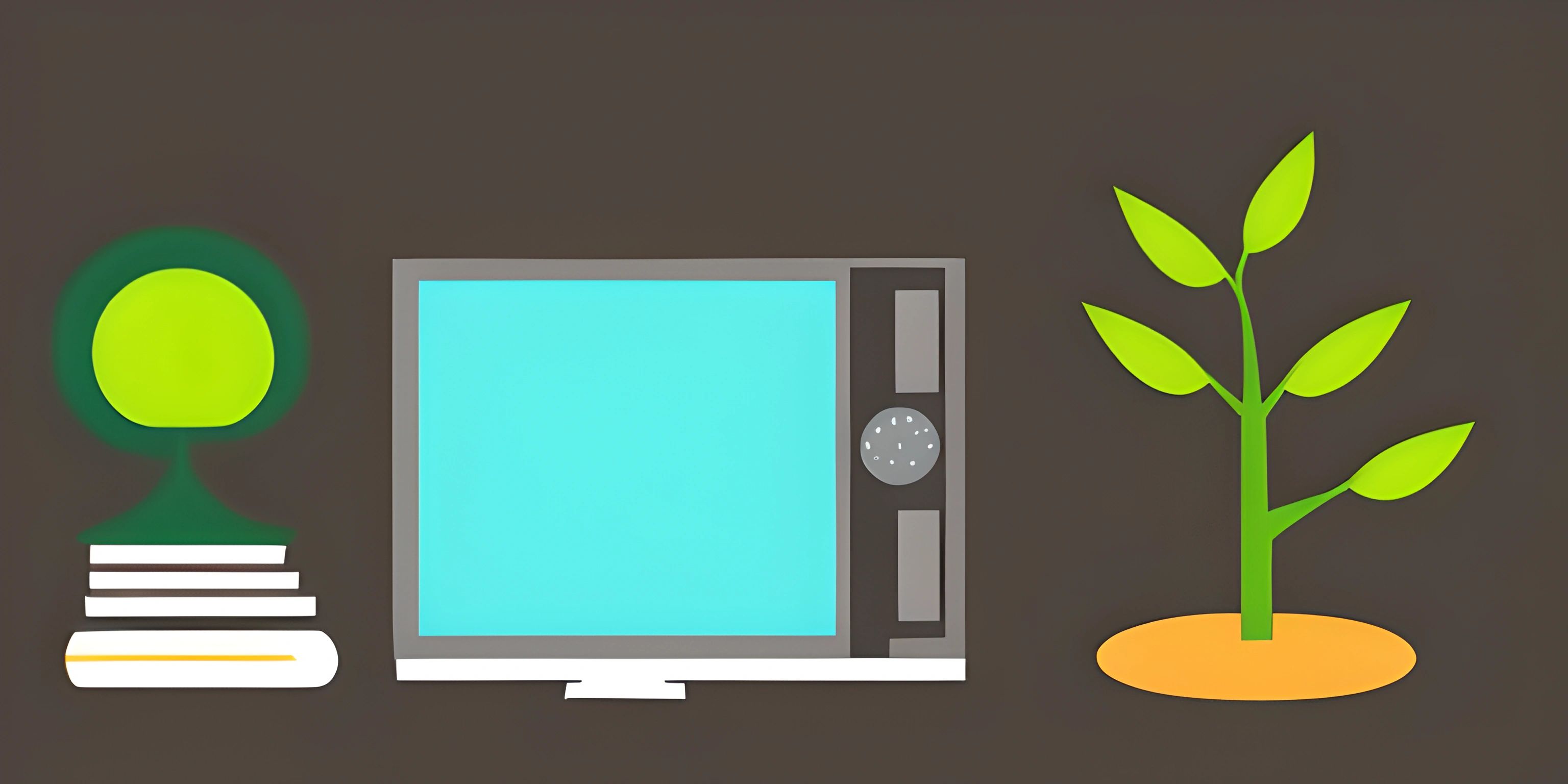
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Controlling program flow is crucial in any programming language, and Java is no exception. In this article, we'll dive deep into conditional statements in Java, such as if, else, and switch, to help you direct your program's flow with ease.
If Statements
In Java, an if
statement is used to conditionally execute code based on a given condition. When the condition is true, the block of code within the if
statement is executed. If the condition is false, the code is skipped. Let's take a look at a simple example:
int age = 18; if (age >= 18) { System.out.println("You are eligible to vote!"); }
In the example above, we have an integer variable age
with a value of 18. The if
statement checks if the age is greater than or equal to 18. Since the condition is true, the code within the if
block is executed, and "You are eligible to vote!" is printed.
Else Statements
Sometimes, you may want to execute some code when the if
condition is false. That's when else
comes into play. The else
statement follows an if
statement and is executed when the if
condition is false. Let's take a look at the voting example again, but this time with an else
statement:
int age = 16; if (age >= 18) { System.out.println("You are eligible to vote!"); } else { System.out.println("Sorry, you are not eligible to vote yet."); }
In this example, the age is set to 16, which makes the condition in the if
statement false. As a result, the code within the else
block is executed, and "Sorry, you are not eligible to vote yet." is printed.
Else If Statements
What if you have multiple conditions to check? That's where the else if
statement comes in handy. An else if
statement follows an if
statement and allows you to check for additional conditions. If the previous conditions are false, the else if
condition is checked. You can chain multiple else if
statements together to check for various conditions. Let's look at an example that demonstrates this:
int marks = 85; if (marks >= 90) { System.out.println("Grade: A"); } else if (marks >= 80) { System.out.println("Grade: B"); } else if (marks >= 70) { System.out.println("Grade: C"); } else { System.out.println("Grade: D"); }
In this example, we check the student's grade based on their marks. The program first checks if the marks are greater than or equal to 90. If this is false, it moves on to the next else if
statement to check if the marks are greater than or equal to 80, and so on. The output for the given marks
value (85) would be "Grade: B".
Switch Statements
A switch
statement is another way of controlling program flow in Java. It allows you to execute different code blocks based on the value of a variable or expression. The switch
statement is particularly useful when you have a limited set of values to compare, as it can make your code more readable and concise. Here's an example that demonstrates the use of a switch
statement:
int dayOfWeek = 3; switch (dayOfWeek) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; case 3: System.out.println("Wednesday"); break; case 4: System.out.println("Thursday"); break; case 5: System.out.println("Friday"); break; case 6: System.out.println("Saturday"); break; case 7: System.out.println("Sunday"); break; default: System.out.println("Invalid day of the week."); }
This example prints the day of the week based on the integer value of dayOfWeek
. The switch
statement compares the value of dayOfWeek
to each case
. When a match is found, the code within that case
is executed, followed by a break
statement to exit the switch
. If no match is found, the code within the default
block is executed.
Now that you have a solid understanding of conditional statements in Java, you can confidently control your program's flow and create more dynamic and responsive applications. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).