Python Turtle Projects
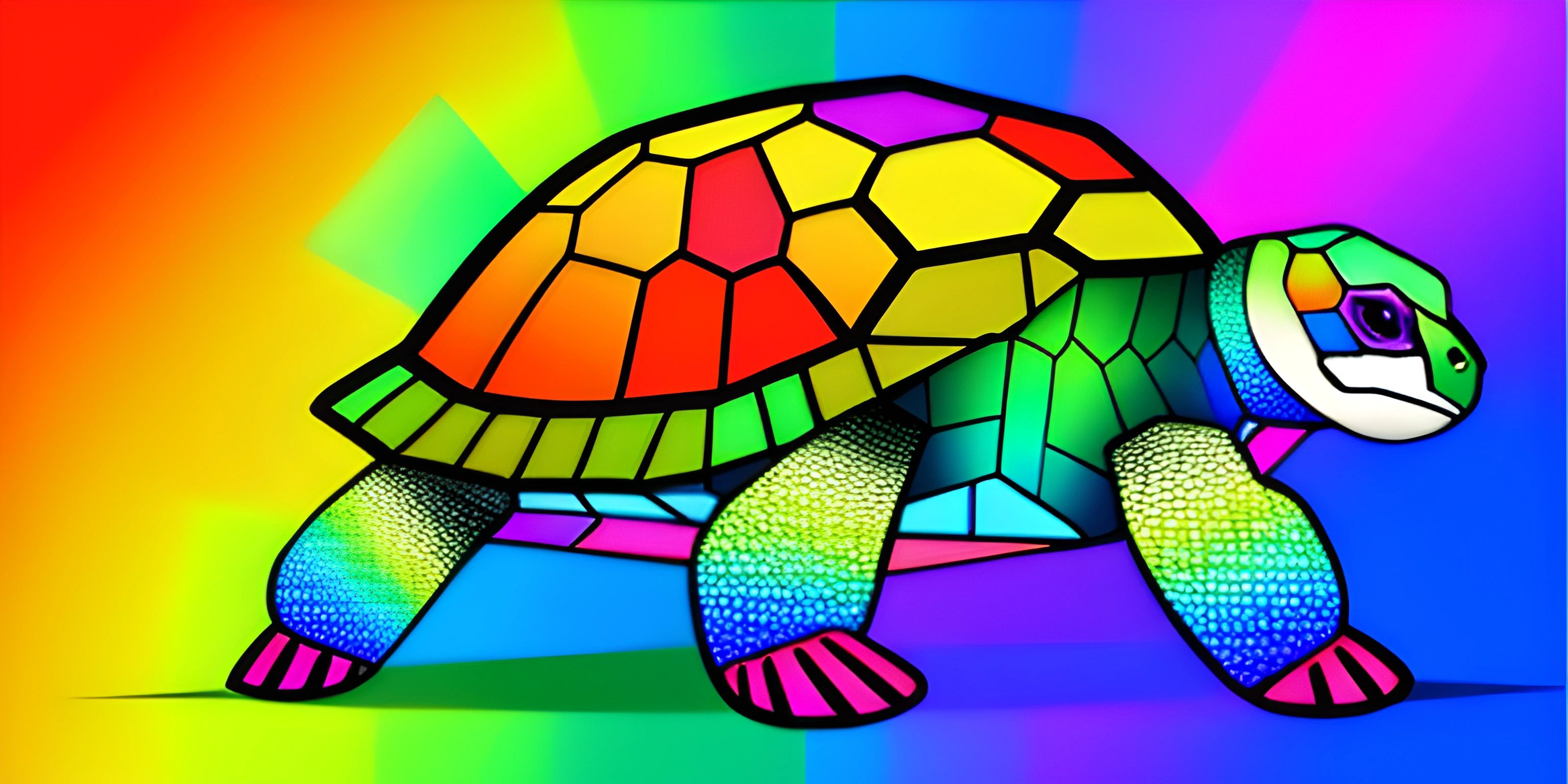
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python Turtle is a fantastic library that allows you to create beautiful graphics effortlessly. It's an excellent way to explore programming concepts while flexing your artistic muscles. In this article, we'll explore a variety of creative projects you can try using Python Turtle. So get ready to unleash your inner artist and have some fun with coding!
Basic Shapes
Let's start with a simple project that introduces you to Python Turtle's drawing capabilities. In this project, we'll create basic shapes such as squares, triangles, and circles.
First, make sure you have the Python Turtle library installed. Then try the following code to draw a square:
import turtle t = turtle.Turtle() for i in range(4): t.forward(100) t.right(90) turtle.mainloop()
You can now experiment with different shapes by modifying the range()
value and the angle in t.right()
. For example, to draw a triangle, change the range()
value to 3 and the angle to 120.
Spiral Art
Ready to get a little more creative? In this project, we'll make colorful spiral patterns using Python Turtle.
import turtle import random t = turtle.Turtle() turtle.bgcolor("black") t.speed(0) colors = ["red", "blue", "green", "yellow", "purple", "orange"] for i in range(100): t.color(random.choice(colors)) t.forward(i * 4) t.right(120) turtle.mainloop()
Feel free to play around with the colors, angles, and range values to create your own unique spiral designs.
Fractal Trees
Fractal trees are a mesmerizing way to explore recursion with Python Turtle. Here's the code to create a simple fractal tree:
import turtle def draw_tree(branch_length, t): if branch_length > 5: t.forward(branch_length) t.right(20) draw_tree(branch_length - 15, t) t.left(40) draw_tree(branch_length - 15, t) t.right(20) t.backward(branch_length) t = turtle.Turtle() turtle.bgcolor("white") t.left(90) t.up() t.backward(100) t.down() t.color("green") draw_tree(100, t) turtle.mainloop()
Experiment with different values for branch length, angles, and conditions to create a variety of fractal tree patterns.
Maze Generator
Want to challenge your Python Turtle skills further? Try building a maze generator using the Depth-First Search algorithm. This project will help you understand algorithms and data structures while creating fascinating mazes.
You can start by defining a grid, implementing the Depth-First Search algorithm, and using Python Turtle to draw the generated maze.
Conclusion
Python Turtle offers endless possibilities for creative projects. We've explored basic shapes, spiral art, fractal trees, and maze generation, but don't stop there! You can create animations, interactive games, or even simulate natural phenomena like Conway's Game of Life. The sky's the limit when it comes to Python Turtle projects, so go ahead and unleash your creativity!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).