Random Walks in Generative Art
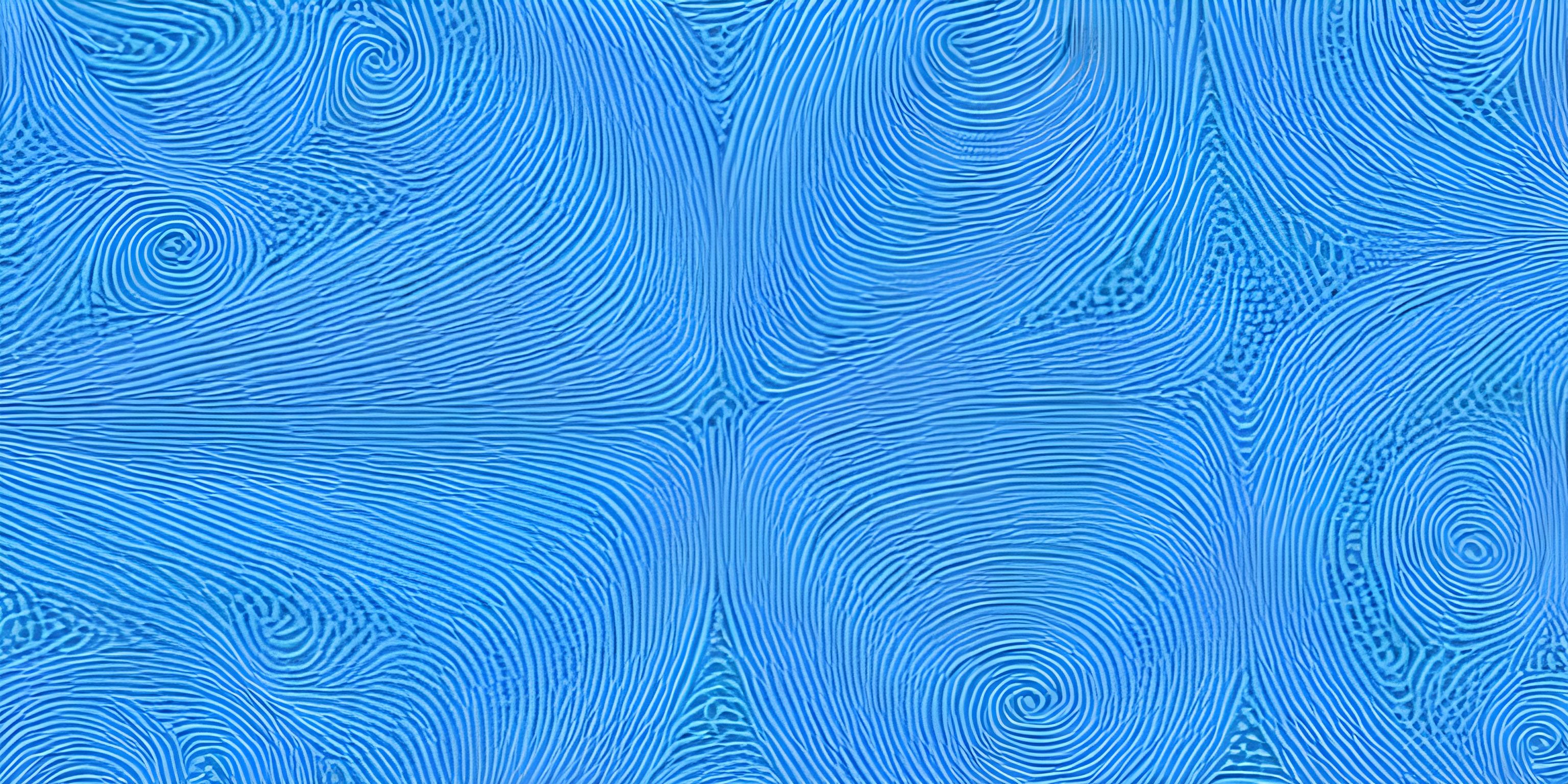
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Generative art combines the beauty of randomness with the rigor of algorithms to create unique, often mesmerizing pieces. One fascinating technique in this realm is the random walk. Imagine a drunken artist who takes steps in random directions to create a painting. This whimsical painter is our metaphor for a random walk!
What is a Random Walk?
A random walk is a mathematical process that describes a path consisting of a succession of random steps. In 2D space, this could mean moving up, down, left, or right with equal probability. It’s like flipping a coin to decide which way to go each time you take a step.
Applications in Generative Art
In generative art, random walks can be used to create intricate, organic-looking patterns. The randomness introduces a level of unpredictability, making each piece unique and often surprisingly complex. We'll explore how to implement this using Python and libraries such as turtle
and matplotlib
.
Implementing Random Walks in Python
Let's get our hands dirty with some code! We'll start simple and gradually add complexity. First, we need to set up our environment. Make sure you have Python installed, along with the turtle
and matplotlib
libraries.
pip install matplotlib
Basic Random Walk with Turtle
The turtle
module is a great way to visualize random walks. Think of it as a turtle crawling across your screen, leaving a trail behind.
import turtle import random # Screen setup screen = turtle.Screen() screen.title("Random Walk with Turtle") # Turtle setup walker = turtle.Turtle() walker.speed(0) # Fastest speed # Random walk function def random_walk(steps): for _ in range(steps): angle = random.choice([0, 90, 180, 270]) walker.setheading(angle) walker.forward(20) # Perform the random walk random_walk(200) # End the drawing screen.mainloop()
In this script, our turtle takes 200 steps in random directions. Each step is 20 units long, and the turtle turns by 0, 90, 180, or 270 degrees randomly.
Enhancing the Walk
To make things more interesting, let's add some color and vary the step lengths.
import turtle import random # Screen setup screen = turtle.Screen() screen.title("Enhanced Random Walk with Turtle") # Turtle setup walker = turtle.Turtle() walker.speed(0) # Fastest speed # Random walk function with color and varied step length def enhanced_random_walk(steps): colors = ["red", "green", "blue", "yellow", "purple", "orange"] for _ in range(steps): walker.color(random.choice(colors)) angle = random.choice([0, 90, 180, 270]) walker.setheading(angle) walker.forward(random.randint(10, 30)) # Perform the enhanced random walk enhanced_random_walk(200) # End the drawing screen.mainloop()
Now, each step not only has a random direction but also a random color and length, making the walk even more captivating.
Visualizing with Matplotlib
While turtle
is fun, matplotlib
allows us to create and save more complex visualizations. Let's craft a random walk and visualize it using matplotlib
.
import matplotlib.pyplot as plt import random # Random walk function def random_walk_2d(n_steps): x, y = [0], [0] for _ in range(n_steps): direction = random.choice(['N', 'S', 'E', 'W']) if direction == 'N': x.append(x[-1]) y.append(y[-1] + 1) elif direction == 'S': x.append(x[-1]) y.append(y[-1] - 1) elif direction == 'E': x.append(x[-1] + 1) y.append(y[-1]) elif direction == 'W': x.append(x[-1] - 1) y.append(y[-1]) return x, y # Generate random walk x, y = random_walk_2d(1000) # Plot the walk plt.figure(figsize=(10, 10)) plt.plot(x, y, color="blue", linestyle="-", linewidth=1) plt.title("2D Random Walk") plt.xlabel("X-axis") plt.ylabel("Y-axis") plt.grid(True) plt.show()
In this example, we simulate a 2D random walk with 1000 steps. The path is plotted on a grid, allowing us to see the entire journey.
Beyond the Basics
Random walks can be extended and modified in countless ways. You can introduce constraints, like boundaries or obstacles, or even fuse random walks with other generative techniques, such as Perlin noise or fractals.
Random Walk with Boundaries
Let's consider a random walk confined within a square boundary.
import matplotlib.pyplot as plt import random # Random walk function with boundaries def bounded_random_walk(n_steps, boundary): x, y = [0], [0] for _ in range(n_steps): direction = random.choice(['N', 'S', 'E', 'W']) new_x, new_y = x[-1], y[-1] if direction == 'N': new_y += 1 elif direction == 'S': new_y -= 1 elif direction == 'E': new_x += 1 elif direction == 'W': new_x -= 1 # Check boundaries if abs(new_x) <= boundary and abs(new_y) <= boundary: x.append(new_x) y.append(new_y) return x, y # Generate bounded random walk x, y = bounded_random_walk(1000, 50) # Plot the walk plt.figure(figsize=(10, 10)) plt.plot(x, y, color="red", linestyle="-", linewidth=1) plt.title("Bounded 2D Random Walk") plt.xlabel("X-axis") plt.ylabel("Y-axis") plt.grid(True) plt.xlim(-50, 50) plt.ylim(-50, 50) plt.show()
This script confines the walk within a boundary of ±50 units on both the x and y axes. The walker can move freely within this space but is stopped at the boundaries.
Conclusion
Random walks offer a fascinating way to infuse unpredictability into generative art. By leveraging Python libraries like turtle
and matplotlib
, you can create captivating visualizations and explore the endless possibilities of algorithmic creativity.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is a random walk?
A random walk is a mathematical process involving a series of steps in random directions. In 2D space, this means moving up, down, left, or right with equal probability.
How can I visualize a random walk in Python?
You can use the turtle
module for simple visualizations and matplotlib
for more complex plots. Both libraries are excellent for creating and displaying random walks.
Can I add boundaries to a random walk?
Yes, you can introduce boundaries by checking the walker's position at each step and ensuring it stays within the defined limits. If the walker reaches a boundary, you can prevent it from moving further in that direction.
What are some variations of random walks?
Variations include changing step lengths, introducing different probabilities for directions, adding boundaries, or combining random walks with other generative techniques like Perlin noise or fractals.
Why use random walks in generative art?
Random walks introduce unpredictability and complexity, making each piece unique and often unexpectedly intricate. They can create organic, natural-looking patterns that are difficult to achieve with deterministic algorithms.