React Reconciliation Deep Dive
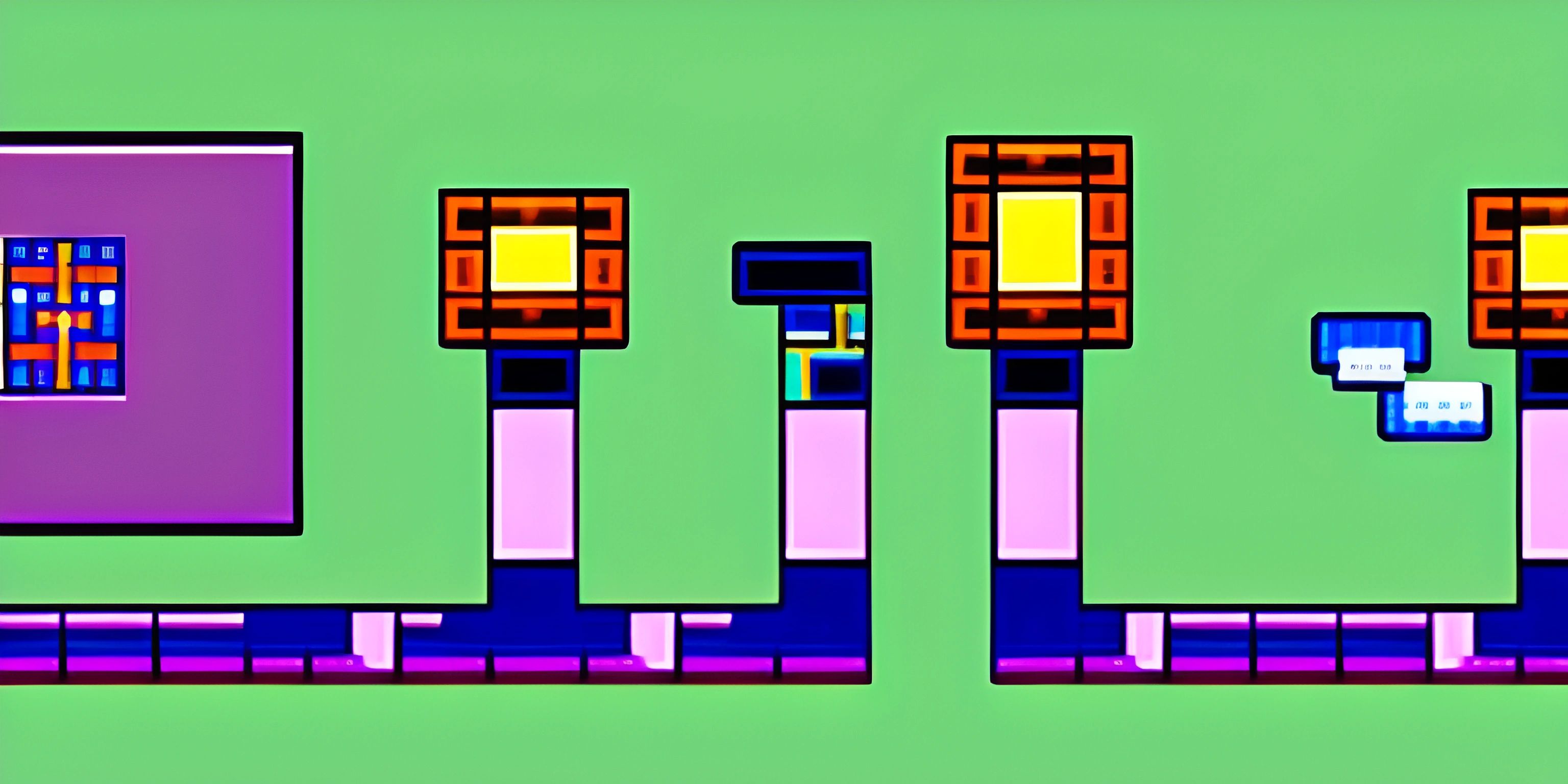
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React is known for its ability to build fast and efficient user interfaces. One of the key factors in achieving this performance is reconciliation. In this article, we'll dive into the reconciliation process, discover its inner workings, and learn how it helps improve the performance of React applications.
What is Reconciliation?
Reconciliation is the process React uses to determine which parts of the DOM need to be updated when the application's state changes. React achieves this through the use of a virtual DOM. The virtual DOM is an in-memory representation of the actual DOM, which React uses to calculate the minimal set of changes required to update the real DOM. This process is also called diffing.
The Virtual DOM
The virtual DOM is a lightweight representation of the actual DOM. It consists of React elements, which are JavaScript objects containing information about a DOM node, such as its type, properties, and children. When the application state changes, React creates a new virtual DOM tree that represents the updated UI.
The Diffing Algorithm
React's diffing algorithm is the heart of the reconciliation process. This algorithm compares the new virtual DOM tree with the old one to determine the minimal set of changes needed to update the real DOM. This process is also known as tree reconciliation.
The diffing algorithm works in two steps:
-
Element comparison: React compares elements at the same level in the tree, checking their types and properties. If the types are different, React assumes the whole subtree needs to be replaced.
-
Children reconciliation: If the element types are the same, React moves on to reconcile their children. It uses a key attribute to optimize this process, allowing it to identify which child elements have been added, removed, or reordered.
Why is Reconciliation Important?
Reconciliation is crucial for performance optimization in React applications. Updating the DOM is often the most time-consuming part of rendering a web application, and React's reconciliation process helps minimize the number of costly DOM updates.
By calculating the minimal set of changes required to update the real DOM, React can avoid updating parts of the UI that haven't changed, significantly reducing the time and resources needed for rendering updates.
Optimizing Reconciliation
While React's reconciliation process is efficient, there are ways to further optimize it for your application. Some best practices include:
-
Using keys: Assigning unique keys to elements in a collection can significantly speed up the reconciliation process, as it helps React quickly identify which elements have been added, removed, or reordered.
-
Component updates: Use the
shouldComponentUpdate
lifecycle method orReact.memo
to prevent unnecessary component updates when the component's state or props haven't changed. -
Pure components: Create components that only depend on their props and state and have no side effects. This makes it easier for React to determine if a component needs to be updated.
By following these best practices, you can ensure that your React applications run smoothly and efficiently.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is React's reconciliation process?
Reconciliation is the process React uses to determine which parts of the DOM need updating when the application's state changes. It does this by comparing a new virtual DOM tree with the old one and calculating the minimal set of updates required.
How does the virtual DOM help with reconciliation?
The virtual DOM is an in-memory representation of the actual DOM. React uses it to calculate the minimal set of changes needed to update the real DOM, improving performance by avoiding unnecessary updates.
What is the diffing algorithm in React?
The diffing algorithm is the core of the reconciliation process. It compares the new virtual DOM tree with the old one to determine the minimal set of changes needed to update the real DOM. This process is also known as tree reconciliation.
How can you optimize reconciliation in React applications?
To optimize reconciliation, you can follow best practices such as using unique keys for elements in a collection, preventing unnecessary component updates with shouldComponentUpdate
or React.memo
, and creating pure components with no side effects.
Why is reconciliation important for React's performance?
Reconciliation is crucial for performance optimization because it minimizes the number of costly DOM updates. By calculating the minimal set of changes required to update the real DOM, React can avoid updating parts of the UI that haven't changed, significantly reducing rendering time and resources needed.