Implementing Navigation in React Applications
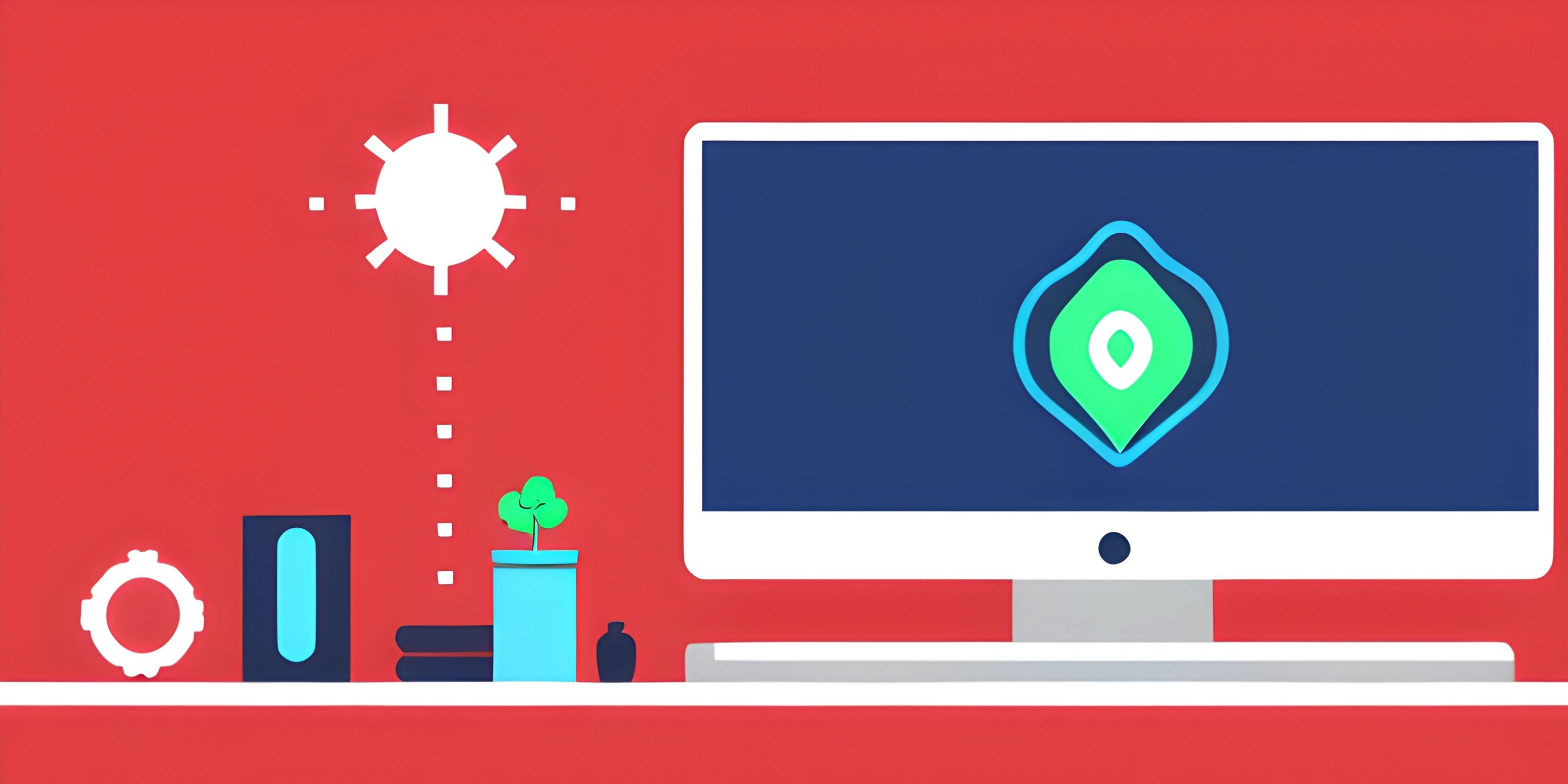
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Navigating through different pages or views is a core functionality of web applications. In the world of React, React Router is a popular choice to handle navigation. It allows you to create dynamic, single-page applications with ease.
Setting up React Router
Before diving into how React Router works, let's set it up in a React project. To do this, you need to install the react-router-dom
package.
npm install react-router-dom
Once the package is installed, you can start using it in your project by importing the required components.
Basic Routing
React Router uses a declarative approach to define routes, meaning you describe what should be displayed based on the current URL. It does so through a set of components, such as BrowserRouter
, Route
, Switch
, and Link
.
BrowserRouter
The BrowserRouter
component is the top-level component that wraps your entire application. It keeps track of the browser's history and provides the routing functionality. You can import it and wrap your app like this:
import { BrowserRouter } from "react-router-dom"; function App() { return ( <BrowserRouter> {/* Your app components go here */} </BrowserRouter> ); } export default App;
Route
The Route
component defines a single route in your application. It takes two main properties: path
and component
. The path
prop specifies the URL path, and the component
prop specifies the React component to render when the route is matched.
Here's an example of defining routes for a simple app with a home page and an about page:
import { BrowserRouter, Route } from "react-router-dom"; import HomePage from "./pages/HomePage"; import AboutPage from "./pages/AboutPage"; function App() { return ( <BrowserRouter> <Route path="/" component={HomePage} /> <Route path="/about" component={AboutPage} /> </BrowserRouter> ); } export default App;
Switch
The Switch
component is used to group multiple Route
components together. It ensures that only one route is rendered at a time, based on the first match it finds. To use Switch
, simply wrap your Route
components with it:
import { BrowserRouter, Route, Switch } from "react-router-dom"; import HomePage from "./pages/HomePage"; import AboutPage from "./pages/AboutPage"; function App() { return ( <BrowserRouter> <Switch> <Route path="/" component={HomePage} /> <Route path="/about" component={AboutPage} /> </Switch> </BrowserRouter> ); } export default App;
Link
The Link
component allows you to navigate between routes. It's similar to a regular <a>
tag, but it doesn't cause a full page refresh. Instead, it updates the URL and renders the matching route. Here's an example of a simple navigation menu:
import { Link } from "react-router-dom"; function Navigation() { return ( <nav> <Link to="/">Home</Link> <Link to="/about">About</Link> </nav> ); } export default Navigation;
Now you have a basic understanding of React Router and how to set up navigation in a React application. With these building blocks, you can create more complex navigation structures, such as nested routes and protected routes. Don't forget to dive deeper into the React Router documentation for additional features and best practices.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is React Router and why is it important for my React applications?
React Router is a powerful library that allows you to manage navigation within your React applications. It provides a clean, declarative way to handle URL routing and seamlessly integrate various components of your application. Using React Router, you can easily handle complex navigation structures and provide a better user experience.
How do I install and set up React Router in my project?
To install and set up React Router in your React project, follow these steps:
- Install the
react-router-dom
package using npm or yarn:
ornpm install react-router-dom
yarn add react-router-dom
- Import the required components from the
react-router-dom
package in your main application file (e.g.,index.js
orApp.js
):import { BrowserRouter as Router, Route, Switch } from "react-router-dom";
- Wrap your main application component with the
<Router>
component and define your routes using<Route>
and<Switch>
components:import React from "react"; import { BrowserRouter as Router, Route, Switch } from "react-router-dom"; import Home from "./components/Home"; import About from "./components/About"; import Contact from "./components/Contact"; function App() { return ( <Router> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> </Switch> </Router> ); } export default App;
How can I create navigation links using React Router?
To create navigation links using React Router, use the <Link>
component, which allows users to navigate between different routes without refreshing the page. Import the Link
component from the react-router-dom
package and use it to create navigation links in your component:
import { Link } from "react-router-dom"; function Navigation() { return ( <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> ); }
How can I handle dynamic routes and route parameters with React Router?
With React Router, you can handle dynamic routes by using route parameters. Route parameters are placeholders in the path that capture values from the URL. To define a route parameter, add a colon (:
) followed by the parameter name in the <Route>
component's path. Access the route parameters using the useParams
hook from the react-router-dom
package. Here's an example:
import { useParams } from "react-router-dom"; function UserProfile() { const { userId } = useParams(); return ( <div> <h2>User Profile</h2> <p>User ID: {userId}</p> </div> ); } // In your route definitions: <Route path="/user/:userId" component={UserProfile} />
In this example, the :userId
route parameter captures the user ID from the URL, and the useParams
hook allows you to access it within the UserProfile
component.