Introduction to RSpec Testing Framework
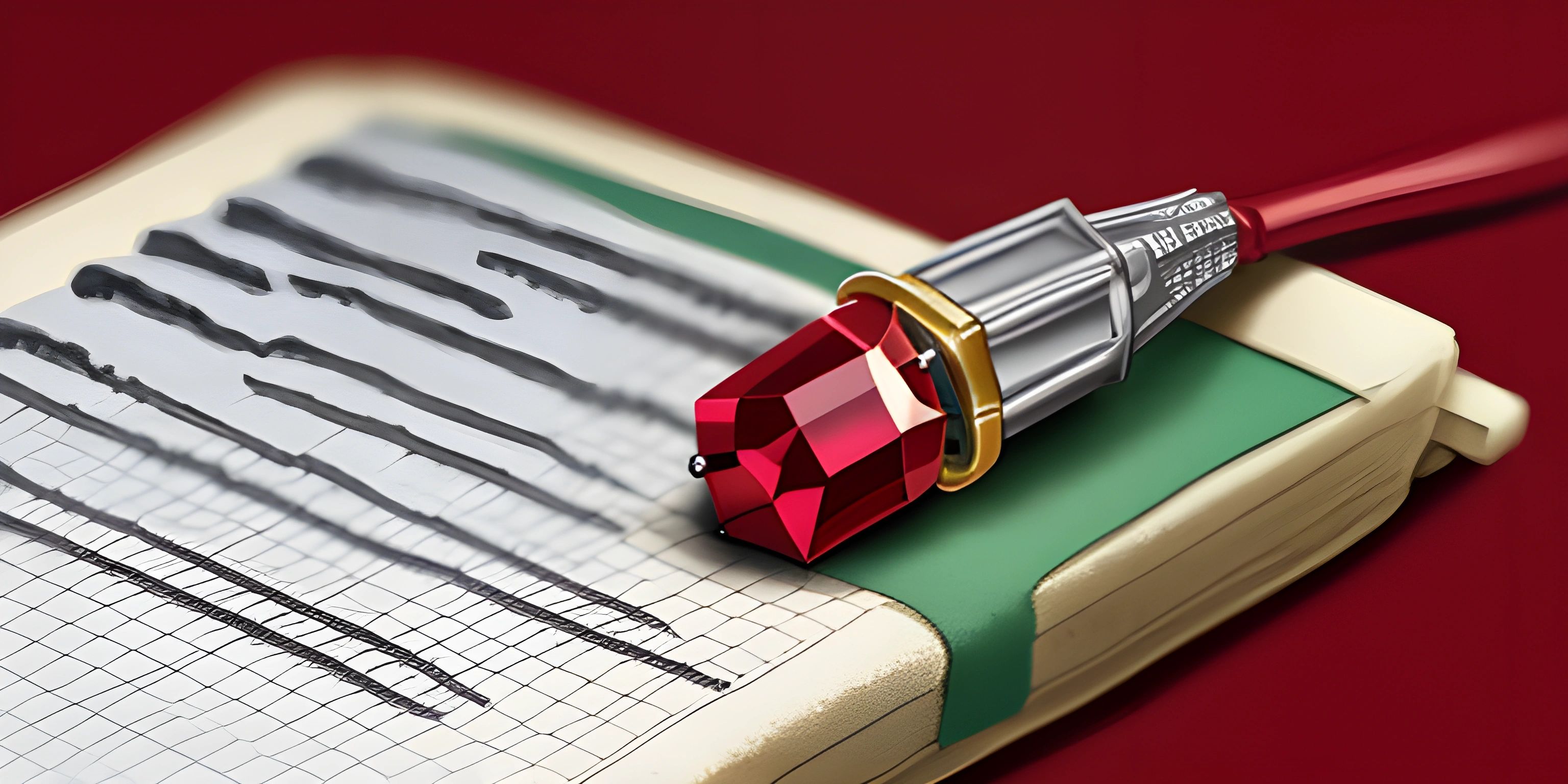
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming, testing is an essential part of the development process. It ensures that our code is reliable, maintainable, and behaves as expected. While there are many testing frameworks available, one of the most popular choices for Ruby developers is RSpec. It's a powerful, expressive, and flexible testing framework that will make your tests as enjoyable to write as the rest of your code. Let's dive into the basics of RSpec, and learn how to get started with your first tests.
Installing RSpec
Before we can start writing tests, we first need to install the RSpec gem. If you're using a Ruby project with a Gemfile, simply add the following line to it:
gem "rspec"
Then, run bundle install
to install the gem. If you're not using a Gemfile, you can install RSpec globally by running:
gem install rspec
With RSpec installed, you can initialize it in your project by running:
rspec --init
This command will create a .rspec
configuration file and a spec
directory in your project, where you will store your test files.
Anatomy of an RSpec Test
RSpec tests are written using a domain-specific language (DSL) that resembles natural language, making it easy to read and understand. Let's take a look at the basic structure of an RSpec test:
describe "A class or module" do context "with a specific condition or state" do it "does something specific" do # Test logic goes here end end end
describe
: This block groups tests related to a specific class, module, or feature. It's the top-level structure of your tests.context
: This block is used to group tests that share a specific condition or state.it
: This block represents an individual test case. It contains the test logic and a description of the expected behavior.
Let's create a simple example to illustrate these concepts. Suppose we have a Calculator
class with an add
method:
class Calculator def add(a, b) a + b end end
Here's how we could write a basic RSpec test for the add
method:
describe Calculator do context "when adding two numbers" do it "returns the correct sum" do calculator = Calculator.new result = calculator.add(2, 3) expect(result).to eq(5) end end end
In this test, we instantiate a new Calculator
object, call its add
method with the numbers 2 and 3, and then use RSpec's expect
method to check if the result equals 5.
Running Your Tests
To run your tests, simply execute the rspec
command followed by the path to your test file:
rspec spec/calculator_spec.rb
RSpec will then execute your tests and display the results, informing you whether your tests have passed or failed.
Conclusion
RSpec is a powerful and expressive testing framework that makes writing tests for your Ruby projects a breeze. With its natural language-like syntax and extensive documentation, you can ensure your code is reliable, maintainable, and behaves as expected. Now that you're familiar with RSpec's basics, it's time to start writing tests for your projects!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: AI Test Playground (psst, it's free!).
FAQ
What is RSpec?
RSpec is a popular testing framework for Ruby. It provides a domain-specific language (DSL) that allows developers to write expressive and readable tests, ensuring that their code is reliable, maintainable, and behaves as expected.
How do I install RSpec?
You can install RSpec by adding the gem "rspec"
line to your project's Gemfile and running bundle install
, or by running gem install rspec
to install it globally. After installation, run rspec --init
to set up RSpec in your project.
How do I run RSpec tests?
To run your RSpec tests, execute the rspec
command followed by the path to your test file, like rspec spec/calculator_spec.rb
. RSpec will then run your tests and display the results.
What are the main components of an RSpec test?
The main components of an RSpec test are describe
, context
, and it
blocks. describe
groups tests related to a specific class, module, or feature. context
groups tests that share a specific condition or state. it
represents an individual test case and contains the test logic and a description of the expected behavior.
Can you give an example of an RSpec test?
Here's an example of an RSpec test for a Calculator
class with an add
method:
describe Calculator do context "when adding two numbers" do it "returns the correct sum" do calculator = Calculator.new result = calculator.add(2, 3) expect(result).to eq(5) end end end