Rust FFI and C Interoperability
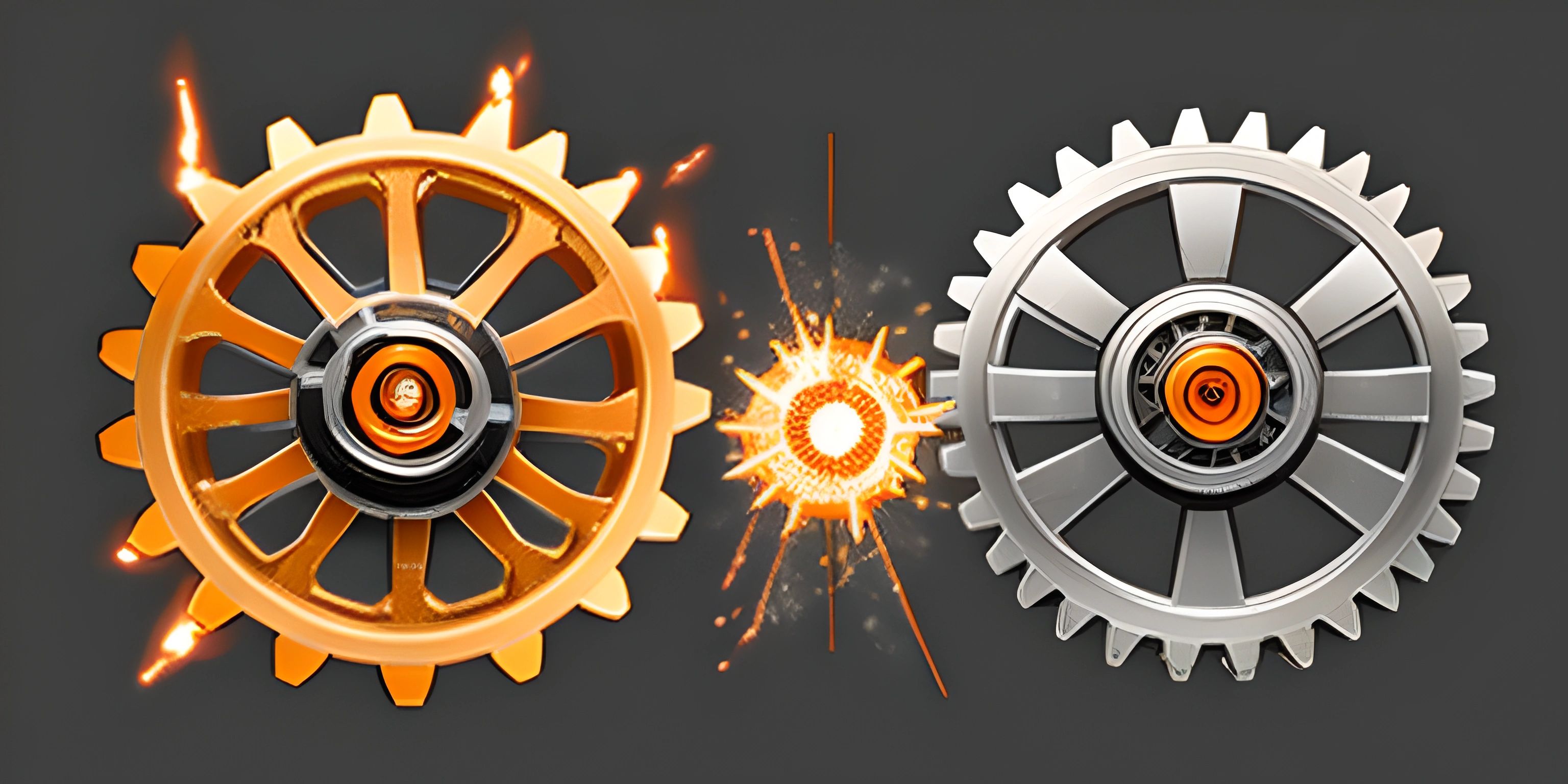
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
One of the strengths of Rust is its interoperability with C, as it allows developers to harness the power of both languages. In this article, we'll explore how Rust's Foreign Function Interface (FFI) enables communication with C code and how to wield this powerful tool effectively.
Rust's Foreign Function Interface (FFI)
Rust's FFI allows Rust programs to call functions written in other programming languages, such as C. This can be particularly useful when you want to leverage existing C libraries or optimize performance-critical parts of your code.
To create an FFI, you need to define an extern
block in your Rust code, which will declare the foreign functions you want to call. Here's an example:
extern "C" { fn my_c_function(arg: c_int) -> c_int; }
The extern "C"
syntax indicates that the functions inside the block are implemented in C. The function signature inside the block should match the C function's signature. In this case, we're declaring a C function called my_c_function
that takes an integer argument and returns an integer.
Working with C Types
When working with FFI, you'll often need to use C types in your Rust code. The libc
crate provides these types, so make sure to add it as a dependency in your Cargo.toml
file:
[dependencies] libc = "0.2"
You can now use the C types from the libc
crate in your Rust code:
use libc::{c_int, c_char}; extern "C" { fn my_c_function(arg: c_int) -> c_int; fn another_c_function(arg: *const c_char) -> *const c_char; }
Calling C Functions from Rust
Once you've declared the foreign functions using an extern
block, you can call them just like any other Rust functions. Let's assume you have a C function called add_numbers
that takes two integers and returns their sum. You can call it from Rust like this:
use libc::c_int; extern "C" { fn add_numbers(a: c_int, b: c_int) -> c_int; } fn main() { let result = unsafe { add_numbers(5, 7) }; println!("The sum is: {}", result); }
Note the use of the unsafe
keyword when calling the C function. This is because Rust can't guarantee the memory safety of the foreign code, so you need to acknowledge the potential risks.
Exposing Rust Functions to C
You can also expose Rust functions to be called from C code. To do this, you need to:
- Define the Rust function with the
pub
and#[no_mangle]
attributes. - Use the
extern
keyword with the desired C calling convention (usually "C"). - Make sure the function's name in the compiled binary matches the expected C function name.
Here's an example of a Rust function that can be called from C:
use libc::c_int; #[no_mangle] pub extern "C" fn rust_multiply(a: c_int, b: c_int) -> c_int { a * b }
To use this function in a C program, you need to declare it in your C code and link against the Rust library:
#include <stdio.h> extern int rust_multiply(int a, int b); int main() { int result = rust_multiply(3, 4); printf("The product is: %d\n", result); return 0; }
Now you have a good understanding of how Rust FFI and C interoperability work. This powerful feature allows you to combine the strengths of both languages and use existing C libraries in your Rust projects, making your development process smoother and more efficient.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Rust FFI and why do I need it for C interoperability?
Rust FFI (Foreign Function Interface) is a mechanism that allows Rust code to interact with code written in other languages, like C. It becomes essential for C interoperability because it enables you to call C functions from Rust and vice versa, allowing seamless integration of the two programming languages.
How do I call a C function from Rust?
To call a C function from Rust, you'll need to create an extern
block with the C function's signature in your Rust code. You'll also need to specify the correct ABI (Application Binary Interface). Here's an example:
extern "C" { fn c_function_name(arg1: type1, arg2: type2) -> return_type; } fn main() { let result = unsafe { c_function_name(arg1, arg2) }; }
Make sure to call the C function within an unsafe
block, as FFI operations are considered unsafe in Rust.
Can I expose Rust functions to be called from C?
Yes, you can expose Rust functions to be called from C by using the #[no_mangle]
attribute and declaring the function as pub extern "C"
. Here's an example:
#[no_mangle] pub extern "C" fn rust_function_name(arg1: type1, arg2: type2) -> return_type { // Your function implementation }
Make sure to include the Rust function in a C header file, so that your C code can reference it properly.
How do I handle strings and other complex types when working with Rust FFI and C interop?
Handling strings and other complex types requires careful management of memory, lifetime, and data representation. For strings, you can use C-compatible string types like CString
and CStr
from the Rust std::ffi
module. For complex data structures, consider using raw pointers and custom structs with #[repr(C)]
attribute to ensure the correct memory layout.
Are there any best practices or tools for working with Rust FFI and C interop?
Yes, some best practices for working with Rust FFI and C interop include:
- Always use the proper ABI and function signatures.
- Make sure to handle unsafe code properly.
- Be mindful of memory management and data representation.
- Write tests for the Rust and C code to ensure compatibility.
In addition to these practices, you can use tools like
bindgen
andcbindgen
to automatically generate Rust FFI bindings and C headers, respectively. These tools can help simplify the process and reduce the risk of errors.