Rust FFI with Vectors
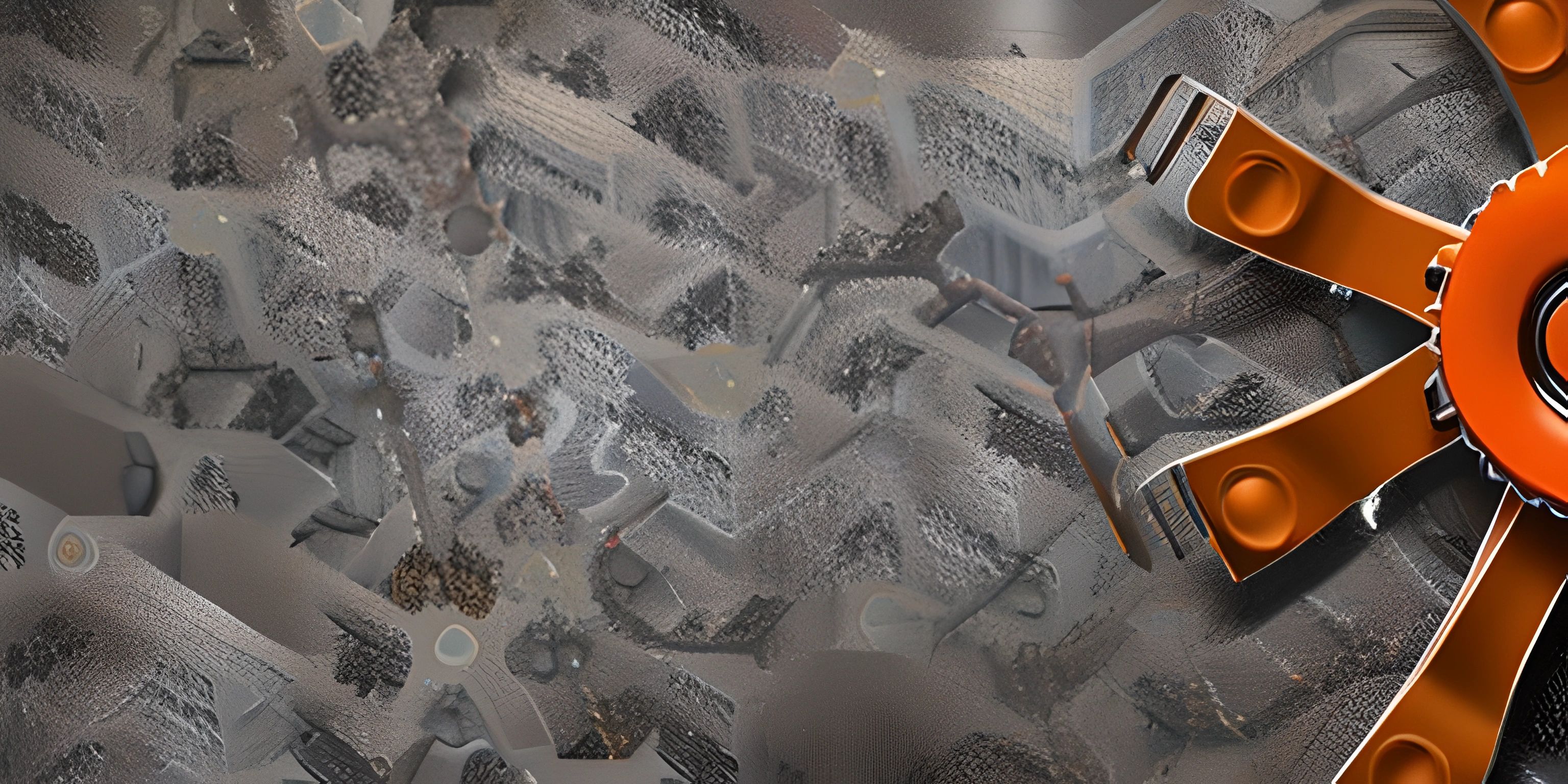
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When you want to dive into the Rust world, you'll probably find yourself wondering about its interoperability with other languages. That's where Rust's foreign function interface (FFI) comes in! FFI allows Rust code to interact with code written in other languages like C or C++. In this article, we'll explore how to use Rust FFI with vectors, one of the most commonly used data structures.
Understanding FFI
Before diving into the specifics of vectors, let's briefly discuss what FFI is. FFI enables communication between two programming languages, allowing them to call each other's functions and share data types. In Rust, the libc
and ctypes
crates are often used to facilitate this interaction.
Now, let's move on to the main focus of this article: working with vectors in Rust FFI.
Vectors in Rust
A vector is a dynamically-sized, contiguous array in Rust. It can grow or shrink in size as needed, and it's part of the standard library. To use vectors in Rust, you need to include the Vec<T>
type from the std::vec
module, where T
is the type of elements in the vector.
Here's an example of creating and manipulating a vector in Rust:
fn main() { let mut my_vec: Vec<i32> = Vec::new(); // Creation of an empty vector my_vec.push(42); // Adding an element to the vector my_vec.push(7); println!("{:?}", my_vec); // Printing the vector: [42, 7] }
Working with FFI and Vectors
To use a vector in Rust FFI, you'll need to expose it to the foreign language code. The common way to do this is by creating a struct that holds a pointer to the data and the length of the vector.
Let's create a simple FFI-compatible struct and a function to convert a Vec<i32>
into this struct:
use std::os::raw::c_void; #[repr(C)] pub struct FfiVector { data: *mut c_void, len: usize, } impl From<Vec<i32>> for FfiVector { fn from(vec: Vec<i32>) -> Self { let len = vec.len(); let data = vec.as_ptr() as *mut c_void; std::mem::forget(vec); // Prevent the vector from being dropped FfiVector { data, len } } }
The FfiVector
struct has a data
field that points to the first element in the array and a len
field that specifies the number of elements. The #[repr(C)]
attribute ensures that the struct has the same memory layout as a corresponding C struct.
The From<Vec<i32>>
implementation converts a Vec<i32>
to an FfiVector
. We cast the vector's pointer to a *mut c_void
and store it in the data
field. We also use std::mem::forget
to prevent Rust from dropping the vector when it goes out of scope, as the memory will be managed by the foreign code.
Now we can use this FfiVector
to share a Rust vector with C code:
// In Rust code extern "C" { fn use_vector(vec: FfiVector); } fn main() { let my_vec = vec![1, 2, 3, 4, 5]; let ffi_vec = FfiVector::from(my_vec); unsafe { use_vector(ffi_vec); } }
And on the C side:
// In C code #include <stdio.h> #include <stdint.h> typedef struct { void* data; size_t len; } FfiVector; void use_vector(FfiVector vec) { int32_t* data = (int32_t*)vec.data; for (size_t i = 0; i < vec.len; i++) { printf("%d\n", data[i]); } }
Keep in mind that memory management is crucial when working with FFI. In this example, the Rust code transfers ownership of the vector's memory to the C code. To avoid memory leaks, you should later deallocate the vector's memory on the Rust side or use a custom allocator that both Rust and C code can use.
And that's it! You now know how to work with Rust FFI and vectors. This knowledge can be a stepping stone to even more complex and powerful interactions between Rust and other languages. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Lifetimes (psst, it's free!).
FAQ
What is Rust's foreign function interface (FFI)?
Rust's foreign function interface (FFI) is a mechanism that allows Rust programs to interact with code written in other programming languages. This can be useful when you want to use a library written in another language or when you need to integrate Rust components into an existing project.
How do I use Rust FFI with vectors?
To use Rust FFI with vectors, you need to follow these steps:
- Create a Rust library with a public function that takes a vector as its input.
- Define the FFI in a C-compatible way using the
libc
crate. - Write a C or C++ program that uses the Rust library and passes a vector to the Rust function.
- Compile and link the C/C++ program with the Rust library. Here's a simple example: In Rust:
use libc::c_int; #[no_mangle] pub extern "C" fn sum_vec(input: *const c_int, len: usize) -> c_int { let slice = unsafe { std::slice::from_raw_parts(input, len) }; slice.iter().map(|&x| x as i32).sum() }
In C/C++:
#include <stdio.h> #include <stdint.h> extern "C" { int32_t sum_vec(const int32_t* input, size_t len); } int main() { int32_t numbers[] = {1, 2, 3, 4, 5}; size_t length = sizeof(numbers) / sizeof(numbers[0]); int32_t result = sum_vec(numbers, length); printf("Sum of the vector: %d\n", result); return 0; }
How can I ensure memory safety when using Rust FFI with vectors?
When using Rust FFI with vectors, memory safety can be a concern because you're dealing with raw pointers. To ensure memory safety, keep these points in mind:
- Always validate the input pointer and length before using them.
- Use
unsafe
blocks to work with raw pointers, but make sure to limit the scope of theunsafe
block as much as possible. - Be cautious when converting between C and Rust types, especially when dealing with signed and unsigned integers.
Can I return a Rust vector from an FFI function to C/C++ code?
Yes, you can return a Rust vector from an FFI function, but you need to convert it into a C-compatible representation first. Make sure to handle memory allocation and deallocation properly. Here's an example: In Rust:
use libc::{c_int, size_t}; #[no_mangle] pub extern "C" fn create_vec(len: size_t) -> *mut c_int { let mut v = Vec::with_capacity(len); v.extend((0..len).map(|_| 0)); let ptr = v.as_mut_ptr(); std::mem::forget(v); ptr } #[no_mangle] pub extern "C" fn free_vec(ptr: *mut c_int, len: size_t) { unsafe { let _ = Vec::from_raw_parts(ptr, len, len); } }
In C/C++:
#include <stdio.h> #include <stdint.h> extern "C" { int32_t* create_vec(size_t len); void free_vec(int32_t* ptr, size_t len); } int main() { size_t length = 5; int32_t* numbers = create_vec(length); // Use the vector here... free_vec(numbers, length); return 0; }
Remember that the C/C++ code is responsible for calling free_vec
to deallocate the memory when it's no longer needed.