Rust Foreign Function Interface (FFI)
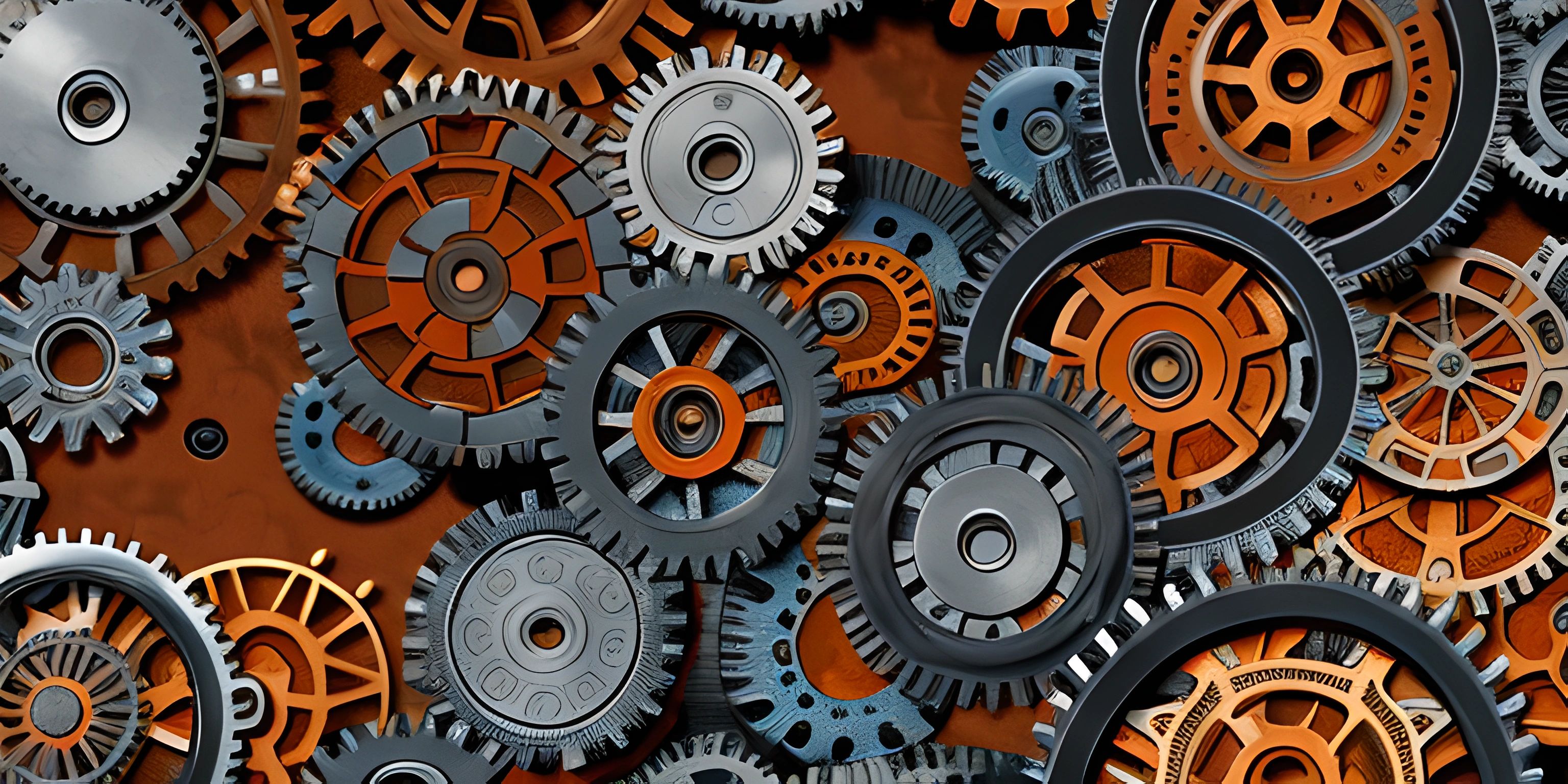
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming, there are times when we need to tap into the power of a different language or low-level assembly code. Luckily, Rust provides a mechanism for that, known as the Foreign Function Interface (FFI). In this article, we'll explore Rust FFI and see how it allows us to communicate with assembly code and other languages.
What is Rust FFI?
Rust FFI is a feature that allows Rust programs to interface with code written in other languages, such as C, C++, or assembly. This is particularly useful when you need to reuse existing code, leverage low-level optimizations, or access functionality not available directly in Rust.
Interfacing with Assembly Code
Let's begin by interfacing Rust with some assembly code. For this example, we'll use a simple assembly function that adds two numbers together.
Here's the assembly code, saved as addition.asm
:
global add_numbers section .text add_numbers: add rdi, rsi mov rax, rdi ret
Now, let's compile the assembly code into an object file:
nasm -f elf64 addition.asm -o addition.o
With the object file ready, it's time to interface with it using Rust FFI.
First, create a new Rust project:
cargo new rust_ffi_example cd rust_ffi_example
Next, open the src/main.rs
file in your favorite editor and modify it like this:
extern "C" { fn add_numbers(a: u64, b: u64) -> u64; } fn main() { let x = 5; let y = 7; let result: u64; unsafe { result = add_numbers(x, y); } println!("The sum of {} and {} is {}.", x, y, result); }
The extern "C"
block tells Rust to look for an external function named add_numbers
with C-compatible calling conventions. The unsafe
block is required because FFI can potentially lead to undefined behavior if used incorrectly.
Finally, link the object file to the Rust project:
rustc src/main.rs --extern add_numbers=addition.o -o rust_ffi_example
Now, when you run the rust_ffi_example
binary, you should see the following output:
The sum of 5 and 7 is 12.
That's it! You've successfully interfaced Rust with assembly code using FFI.
Interfacing with Other Languages
Rust FFI can also be used to interface with code written in other languages, such as C or C++. The process is similar to what we did with assembly code.
For example, let's create a simple C function in a file named multiplication.c
:
#include <stdint.h> uint64_t multiply_numbers(uint64_t a, uint64_t b) { return a * b; }
Compile the C code into an object file:
gcc -c multiplication.c -o multiplication.o
Modify the Rust code in src/main.rs
to interface with the C function using FFI:
extern "C" { fn add_numbers(a: u64, b: u64) -> u64; fn multiply_numbers(a: u64, b: u64) -> u64; } fn main() { let x = 5; let y = 7; let sum: u64; let product: u64; unsafe { sum = add_numbers(x, y); product = multiply_numbers(x, y); } println!("The sum of {} and {} is {}.", x, y, sum); println!("The product of {} and {} is {}.", x, y, product); }
Link the C object file to the Rust project:
rustc src/main.rs --extern add_numbers=addition.o --extern multiply_numbers=multiplication.o -o rust_ffi_example
Running the rust_ffi_example
binary will now show both the sum and product of the two numbers:
The sum of 5 and 7 is 12. The product of 5 and 7 is 35.
Congratulations! You've interfaced Rust with both assembly and C code using the Foreign Function Interface. This powerful feature opens up a world of possibilities for using existing code and libraries, optimizing performance, and extending Rust's capabilities in your projects.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).
FAQ
What is Rust's Foreign Function Interface (FFI)?
Rust's Foreign Function Interface (FFI) is a mechanism that allows Rust programs to interface with code written in other programming languages, including assembly code. This is useful when you want to use existing libraries or functions from other languages in your Rust project, or when you need to interface with low-level systems programming.
How do I use FFI to call a C function from Rust?
To call a C function from Rust using FFI, follow these steps:
- Declare the C function in your Rust code using the
extern
keyword and specify the C calling convention with"C"
. - Use the
unsafe
keyword when calling the C function since FFI operations are considered unsafe in Rust. Here's an example:
// Declare the C function extern "C" { fn my_c_function(arg1: i32, arg2: i32) -> i32; } fn main() { // Call the C function using the `unsafe` keyword let result = unsafe { my_c_function(1, 2) }; println!("The result is: {}", result); }
Make sure to also link the C library containing the function you want to call.
Can I expose Rust functions to C code using FFI?
Yes, you can expose Rust functions to C code using Rust's FFI. To do so, follow these steps:
- Define the Rust function with
pub
andextern
keywords using the"C"
calling convention. - Use the
#[no_mangle]
attribute to inform the Rust compiler not to mangle the function name. - In your C code, declare the Rust function and call it as you would any other C function.
Here's an example:
In Rust (
my_rust_function.rs
):
#[no_mangle] pub extern "C" fn my_rust_function(arg1: i32, arg2: i32) -> i32 { arg1 + arg2 }
In C (main.c
):
#include <stdio.h> // Declare the Rust function extern int my_rust_function(int arg1, int arg2); int main() { // Call the Rust function int result = my_rust_function(1, 2); printf("The result is: %d\n", result); return 0; }
What are some common challenges when working with Rust FFI?
Some common challenges when working with Rust FFI include:
- Ensuring that the Rust code follows the correct calling conventions for the target language.
- Properly handling memory management, especially when interfacing with languages that do not have Rust's automatic memory management.
- Dealing with the inherent
unsafe
nature of FFI operations, which can lead to undefined behavior if not handled correctly. - Ensuring that the data types used across the languages are compatible and can be safely converted without loss of information.