Rust FFI Array Data
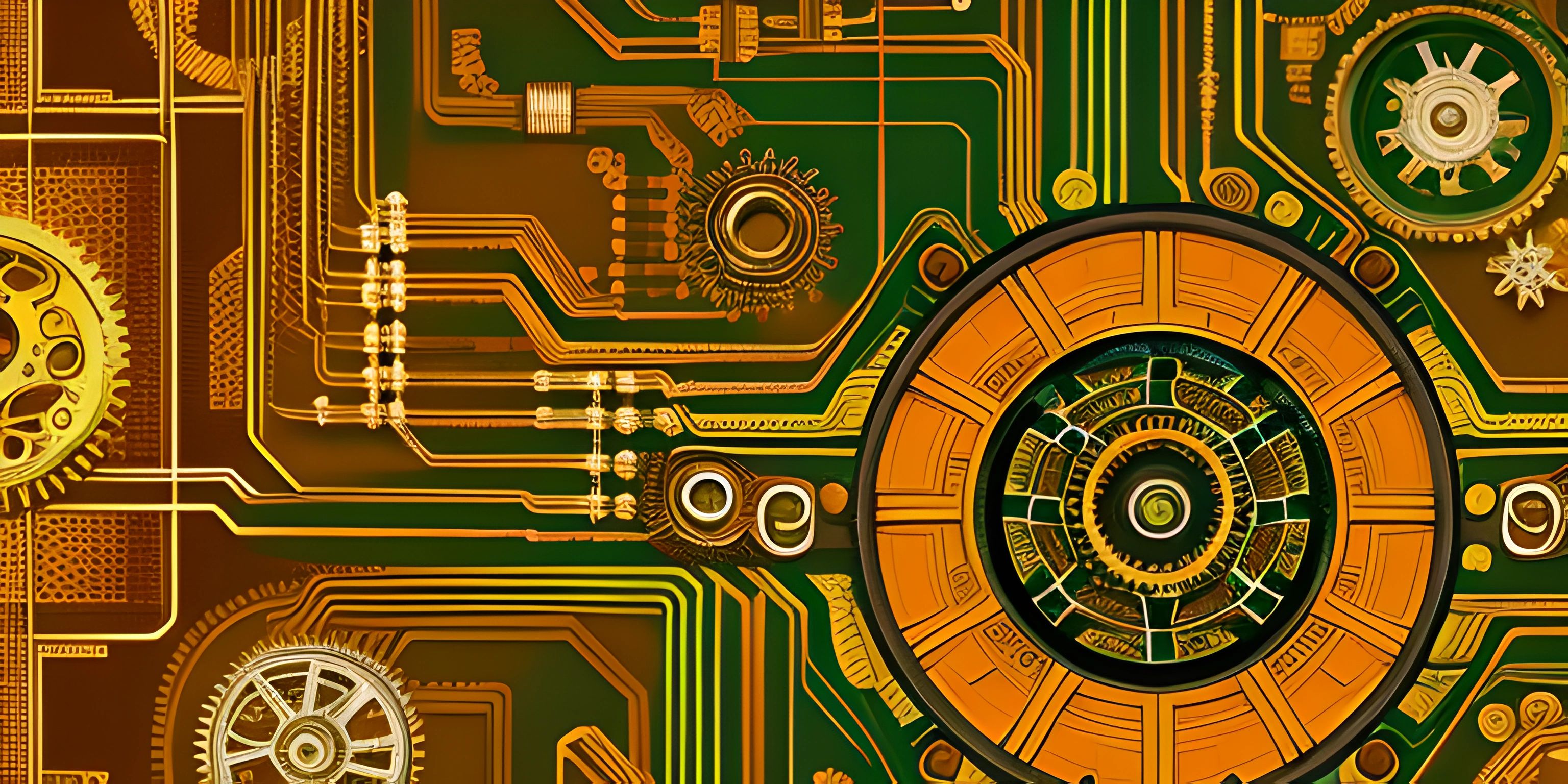
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Foreign Function Interface (FFI) allows Rust to interact with code written in other languages like C and C++. This is useful when you want to benefit from Rust's safety and performance while leveraging existing libraries or integrating with other systems.
In this tutorial, we'll explore a practical example of passing array data through Rust FFI.
Rust FFI Basics
Before diving into our example, let's briefly review some key concepts related to Rust FFI.
Extern Functions
To define an FFI function in Rust, you use the extern
keyword followed by the function signature. This tells the Rust compiler that this function is implemented in another language. Here's an example:
extern "C" { fn c_function(argument: i32) -> i32; }
This code defines a function named c_function
that takes an i32
argument and returns an i32
value. The "C"
string indicates that the function uses the C calling convention.
Unsafe Code
FFI functions are considered unsafe in Rust because the compiler can't guarantee that they follow Rust's safety rules. As such, you need to use the unsafe
keyword to call an FFI function, like this:
let result = unsafe { c_function(42) };
Passing Array Data Through Rust FFI
Now that we have a basic understanding of Rust FFI, let's dive into our example.
We'll start by creating a simple C library that takes an array of integers and returns the sum of its elements. Then, we'll write a Rust program that calls this library.
Creating the C Library
First, create a new directory for the C library and navigate to it:
mkdir c_library cd c_library
Next, create a new file named array_sum.c
and add the following code:
#include <stddef.h> int array_sum(const int* array, size_t length) { int sum = 0; for (size_t i = 0; i < length; i++) { sum += array[i]; } return sum; }
This code defines a function named array_sum
that takes a pointer to an integer array and its length, then calculates the sum of its elements.
Now, create another file named array_sum.h
and add the following code:
#include <stddef.h> int array_sum(const int* array, size_t length);
This header file contains the declaration of the array_sum
function.
Finally, compile the C library into a shared object:
gcc -shared -o libarray_sum.so array_sum.c
Creating the Rust Program
Now, let's create a new Rust project that will use our C library:
cargo new --bin rust_ffi_array_data cd rust_ffi_array_data
Open the Cargo.toml
file and add a build.rs
build script:
[package] # ... build = "build.rs"
Next, create a new file named build.rs
in the project root and add the following code:
fn main() { println!("cargo:rustc-link-search=../c_library"); println!("cargo:rustc-link-lib=dylib=array_sum"); }
This build script tells Cargo where to find the C library and which library to link.
Now, open the src/main.rs
file and add the following code:
use std::os::raw::{c_int, c_uint}; // Import the C function extern "C" { fn array_sum(array: *const c_int, length: c_uint) -> c_int; } fn main() { // Define an integer array let array = [1, 2, 3, 4, 5]; // Call the C function let sum = unsafe { array_sum(array.as_ptr() as *const c_int, array.len() as c_uint) }; // Print the result println!("The sum of the array is: {}", sum); }
This code imports the array_sum
function from the C library, defines an array of integers, and calls the C function to calculate the sum of the array elements. Finally, it prints the result.
Build and run the Rust program:
cargo build cargo run
You should see the following output:
The sum of the array is: 15
Congratulations! You've successfully passed array data through Rust FFI.
Conclusion
In this tutorial, we've explored a practical example of passing array data through Rust FFI. By combining Rust and C, you can leverage existing libraries and integrate with other systems while still benefiting from Rust's safety and performance features. Just remember to use the unsafe
keyword and be cautious when dealing with FFI functions to ensure you maintain Rust's safety guarantees.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Ownership and Borrowing (psst, it's free!).
FAQ
What is Rust FFI and why is it important?
Rust FFI (Foreign Function Interface) is a mechanism that allows Rust code to interact with code written in other programming languages, such as C or C++. This is important because it enables Rust programs to use existing libraries and functionality from other languages, and vice versa, increasing the interoperability and overall usefulness of Rust.
How can I pass array data through Rust FFI?
Passing array data through Rust FFI involves defining the appropriate data structures and functions in the Rust code, and then using the appropriate syntax and types in the other language for the interaction. Here's a simple example in Rust and C: In Rust:
pub extern "C" fn process_array(arr: *const u32, len: usize) { let input_array = unsafe { assert!(!arr.is_null()); slice::from_raw_parts(arr, len) }; // Process the array data here }
In C:
#include <stdint.h> #include <stddef.h> extern void process_array(const uint32_t *arr, size_t len); void main() { uint32_t arr[] = {1, 2, 3, 4}; size_t len = sizeof(arr) / sizeof(arr[0]); process_array(arr, len); }
What are the safety precautions to consider when passing array data through Rust FFI?
When working with Rust FFI, you need to be careful with safety and undefined behavior. Some safety precautions to consider are:
- Always make sure the pointer passed to Rust is not a null pointer.
- Ensure that the length of the array is accurate and not exceeding the actual length of the array.
- Use the
unsafe
keyword in Rust when working with raw pointers and foreign functions.
Can I use Rust FFI to pass array data between Rust and other languages besides C?
Yes, Rust FFI can be used to pass array data between Rust and other languages that support FFI, such as Python, Ruby, or JavaScript. The process is similar to the example given for C, but the syntax and types might vary depending on the specific language you're working with.
How do I handle arrays with different element types when passing data through Rust FFI?
When dealing with arrays of different element types, you'll need to ensure that both the Rust code and the foreign language code use the appropriate data types and conversions. For example, if you're working with an array of floating-point numbers, you'll need to use the corresponding Rust and foreign language types (f32
or f64
in Rust, float
or double
in C) and make sure the data is correctly converted when passing it between the two languages.