Rust Pattern Matching
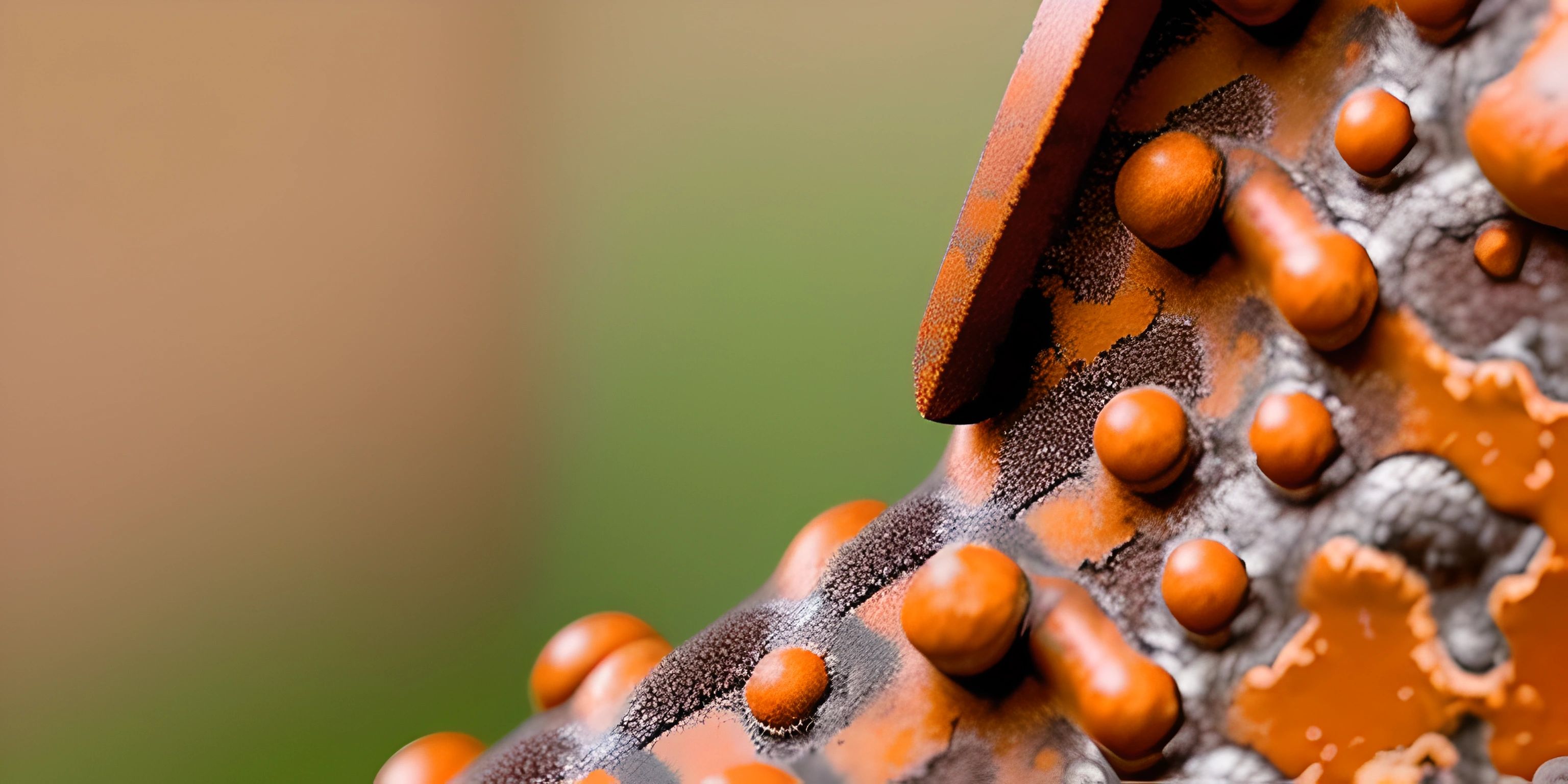
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever played with Rust, you might have noticed that it has a powerful feature called pattern matching. Pattern matching is a versatile and expressive way to control the flow of your program, allowing you to elegantly handle different scenarios. It's like having a Swiss Army knife in your coding toolbox – it can do a lot of things!
What is Pattern Matching?
In a nutshell, pattern matching is a concise way to destructure and compare values against specific patterns. Think of it as a souped-up version of the switch
statement available in other languages, but with more bells and whistles.
The match
Keyword
The heart of pattern matching in Rust is the match
keyword. It allows you to compare a value against a series of patterns and execute the associated code block when a match is found. Let's take a look at a simple example:
fn main() { let number = 42; match number { 0 => println!("The number is zero."), 1 => println!("The number is one."), 42 => println!("Ah, the answer to life, the universe, and everything!"), _ => println!("The number is something else."), } }
Here, we're matching the value of number
against several patterns. If number
is 0, it prints "The number is zero." If it's 1, it prints "The number is one." If it's 42, it prints the famous quote from The Hitchhiker's Guide to the Galaxy. The _
pattern is a catch-all that matches any value, so if number
is something other than 0, 1, or 42, it prints "The number is something else."
Destructuring and Matching
Pattern matching really shines when working with more complex data structures like enums, structs, and tuples. You can destructure and match values at the same time. Let's see it in action with a Rust enum:
enum Message { Quit, ChangeColor(u8, u8, u8), Move { x: i32, y: i32 }, Write(String), } fn main() { let message = Message::ChangeColor(255, 0, 0); match message { Message::Quit => println!("Quit message received."), Message::ChangeColor(r, g, b) => println!("Change color to ({}, {}, {}).", r, g, b), Message::Move { x, y } => println!("Move to ({}, {}).", x, y), Message::Write(text) => println!("Write the text: {}", text), } }
In this example, we have a Message
enum with four variants. The ChangeColor
variant holds a tuple of three u8
values, Move
holds a struct with two i32
values, and Write
holds a String
. The match
expression allows us to destructure and match the variants, giving us access to the inner values while also controlling the flow of the program. This makes for a clean and readable code.
When to Use Pattern Matching
Pattern matching is most useful when you need to destructure and match complex data structures or need a more expressive way to control the flow of your program. It's a powerful tool, but don't forget about other control flow structures like if
, if let
, and while let
, which might be more suitable for simpler scenarios.
With pattern matching in your Rust arsenal, you'll be ready to tackle more complex problems and write elegant, expressive code. So go on, wield the power of the match
keyword like a true Rustacean!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Enums (psst, it's free!).
FAQ
What is pattern matching in Rust?
Pattern matching in Rust is a powerful and flexible feature that allows you to destructure and match data structures, branching on various conditions. It can be used with match
expressions and in if let
, while let
, and for
loops. Pattern matching makes your code more concise and expressive, improving readability and maintainability.
How do I use the `match` expression for pattern matching in Rust?
The match
expression in Rust is used for pattern matching, allowing you to compare a value against different patterns and execute code branches based on the matching pattern. Here's a basic example:
fn main() { let number = 42; match number { 0 => println!("The number is zero"), 1 => println!("The number is one"), _ => println!("The number is something else"), } }
In this example, the match
expression first checks if number
is 0, then 1, and finally uses the wildcard pattern _
to match anything else.
Can I use pattern matching with enums in Rust?
Yes, pattern matching in Rust works well with enums, allowing you to destructure and match on the different variants. Here's an example using an enum with two variants:
enum Animal { Dog(String), Cat(String), } fn main() { let pet = Animal::Dog(String::from("Rover")); match pet { Animal::Dog(name) => println!("This is a dog named {}", name), Animal::Cat(name) => println!("This is a cat named {}", name), } }
In this example, the match
expression checks if pet
is a Dog
or a Cat
, and destructures the enum to get the name of the animal.
How can I use pattern matching in a `for` loop in Rust?
You can use pattern matching in a for
loop by destructuring the elements you're iterating over. For example, when iterating over a vector of tuples:
fn main() { let pairs = vec![(1, "one"), (2, "two"), (3, "three")]; for (number, name) in pairs { println!("{} is called {}", number, name); } }
In this example, the for
loop uses pattern matching to destructure each tuple into number
and name
, making it easy to access the individual elements.