Control Structures in Python
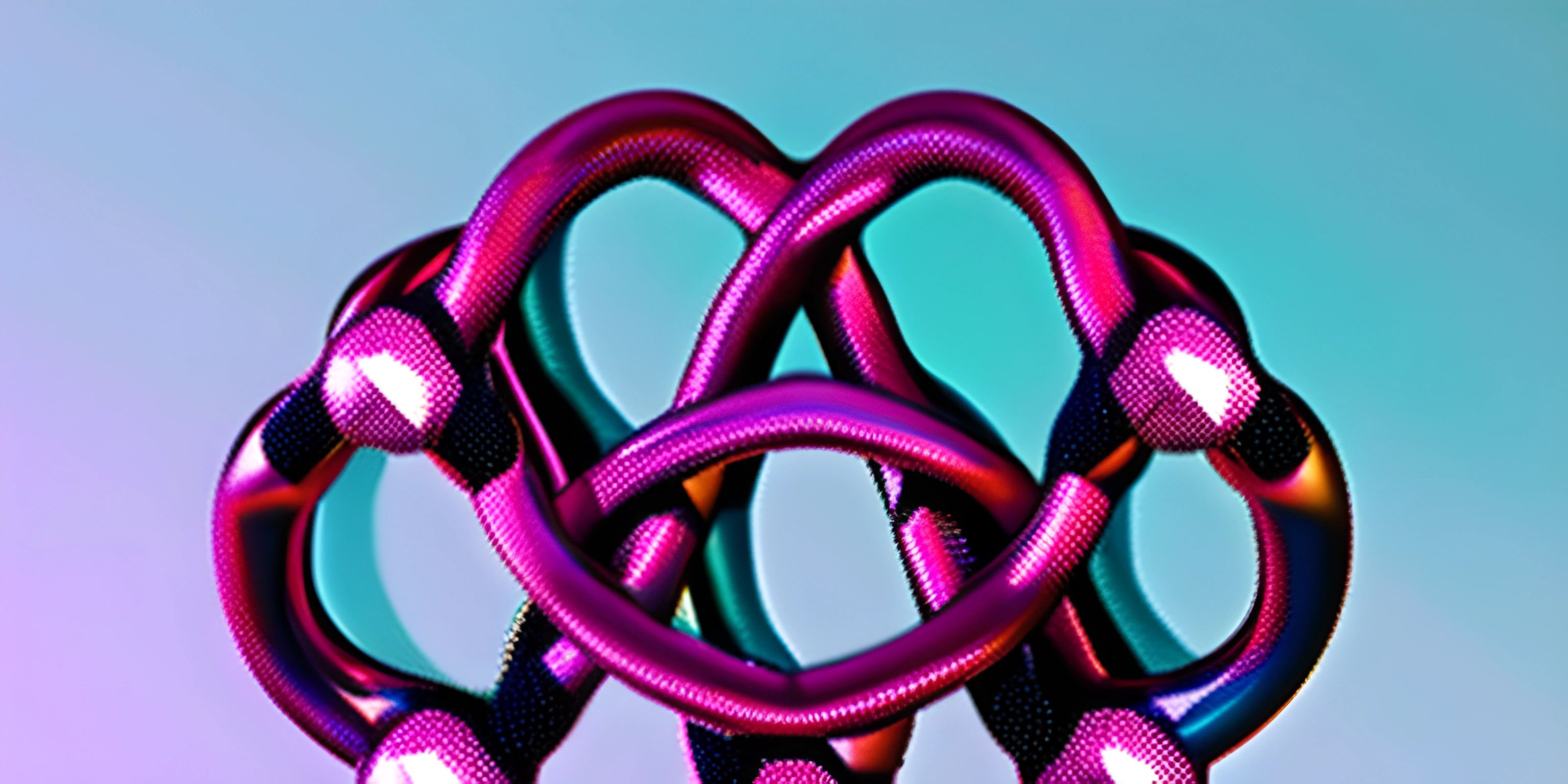
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a programming language that offers a variety of control structures to help you create efficient and organized code. These include loops, conditionals, and exception handling. Let's delve into these concepts and learn how to apply them in Python.
Loops
Loops are essential for performing repetitive tasks. Python provides two types of loops: for
and while
.
For Loops
For
loops are used to iterate over a sequence of elements, such as lists, tuples, or strings. In Python, a for
loop looks like this:
for element in sequence: # code to be executed
For example, let's print the elements of a list:
fruits = ["apple", "orange", "banana"] for fruit in fruits: print(fruit)
This code will output:
apple orange banana
While Loops
While
loops repeatedly execute a block of code as long as a given condition remains true. The syntax for a while
loop is:
while condition: # code to be executed
Here's an example that counts from 1 to 5:
count = 1 while count <= 5: print(count) count += 1
The output will be:
1 2 3 4 5
Conditionals
Conditionals allow you to execute different code based on certain conditions. In Python, you use if
, elif
, and else
statements to create conditional structures.
if condition1: # code to be executed if condition1 is true elif condition2: # code to be executed if condition1 is false and condition2 is true else: # code to be executed if both conditions are false
For example, let's determine if a number is positive, negative, or zero:
number = 0 if number > 0: print("Positive") elif number < 0: print("Negative") else: print("Zero")
This code will output:
Zero
Exception Handling
Exceptions are events that occur during the execution of a program, indicating that something unexpected has happened. Python provides try
, except
, and finally
statements to handle exceptions gracefully.
A try
block contains code that might raise an exception. An except
block catches the exception and determines the appropriate response. The optional finally
block executes code regardless of whether an exception occurred or not.
Here's an example of exception handling in Python:
try: result = 10 / 0 except ZeroDivisionError: print("Oops! You tried to divide by zero.") finally: print("This will always be executed.")
In this case, the output will be:
Oops! You tried to divide by zero. This will always be executed.
By understanding and utilizing Python's control structures, you'll be able to create more efficient and organized code, allowing you to tackle a wide range of programming tasks. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).