Java Control Structures
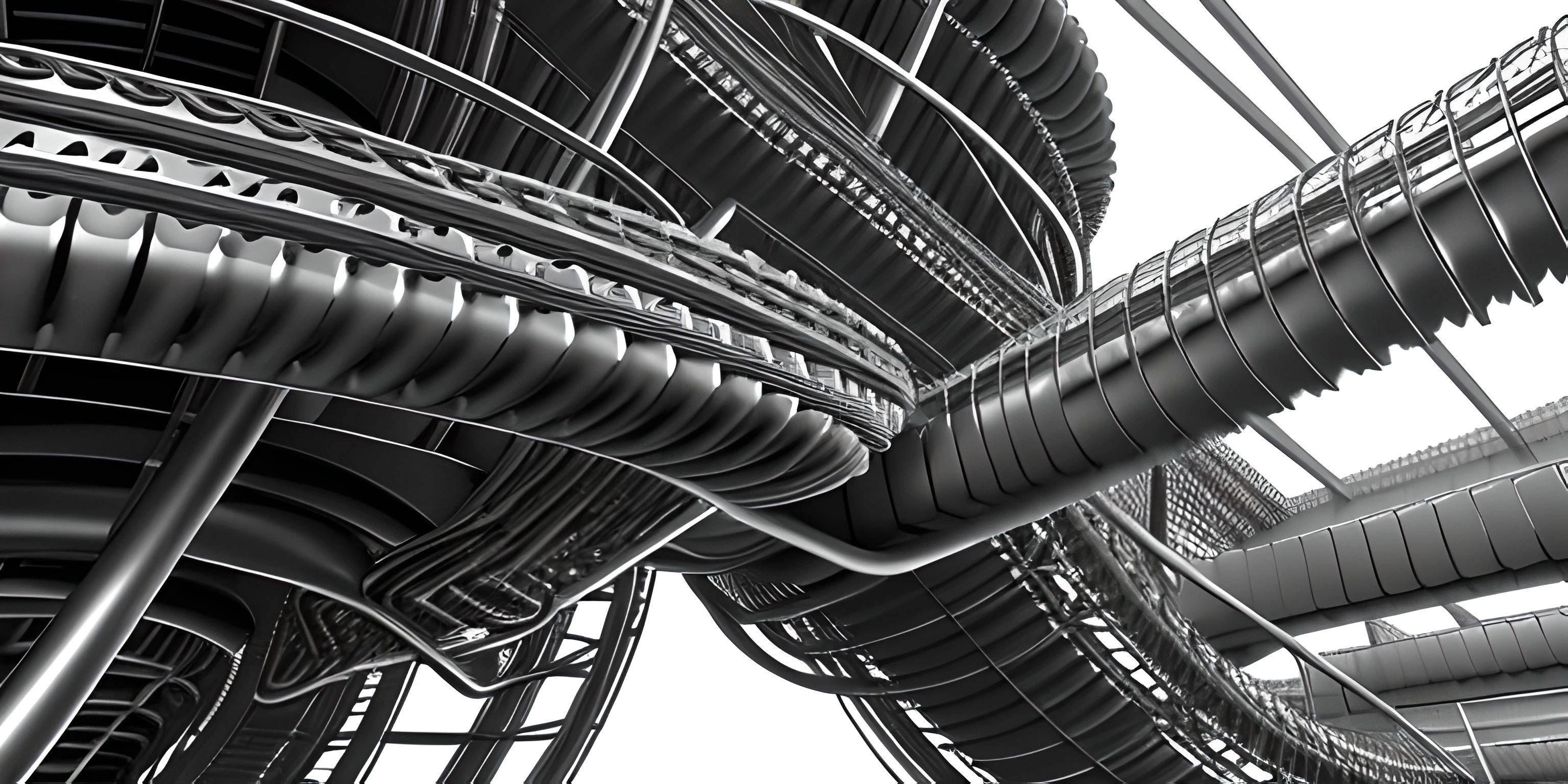
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When venturing into the world of Java programming, it's essential to understand how control structures work. They contribute to the flow of your code and determine how it behaves under different conditions. In this article, we'll explore conditional statements, such as if-else, as well as loops, including for, while, and do-while, in Java.
Conditional Statements
Conditional statements enable your code to make decisions based on specific conditions. The most common conditional statement in Java is the if-else statement.
If-Else Statements
The if-else statement evaluates a condition and executes a block of code if the condition is true. Optionally, an else block can be added to execute when the condition is false. Let's look at an example:
int age = 18; if (age >= 18) { System.out.println("You are an adult."); } else { System.out.println("You are not an adult."); }
In this example, we check if the age
variable is greater than or equal to 18. If it is, we print "You are an adult"; otherwise, we print "You are not an adult."
Loops
Loops are a fundamental part of programming, allowing you to repeat a block of code multiple times. Java offers three primary loop structures: for, while, and do-while loops.
For Loops
For loops are perfect for iterating through a known number of elements, such as arrays or lists. Here's a basic example:
for (int i = 0; i < 5; i++) { System.out.println("Iteration " + i); }
This loop iterates five times, printing "Iteration" followed by the current value of i
. The loop starts with i
equal to 0 and increments it by 1 (i++
) on each iteration until i
is no longer less than 5.
While Loops
While loops continue iterating as long as a specified condition is true. Let's look at an example:
int counter = 0; while (counter < 5) { System.out.println("Counter: " + counter); counter++; }
In this example, the loop will execute as long as the counter
variable is less than 5. It prints "Counter" followed by the current value of counter
and then increments counter
by 1 on each iteration.
Do-While Loops
A do-while loop is similar to a while loop, but it guarantees that the loop will execute at least once, even if the condition is false from the start. Here's an example:
int number = 10; do { System.out.println("Number: " + number); number++; } while (number < 5);
In this case, the loop will execute once, printing "Number: 10", even though number
is not less than 5. After the first iteration, the condition is checked, and the loop exits because the condition is false.
With a solid understanding of Java's control structures, you'll be better equipped to write efficient and effective code. Remember to use the appropriate loop or conditional statement based on your specific requirements, and your Java programs will be in tip-top shape!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).