Best Programming Practices
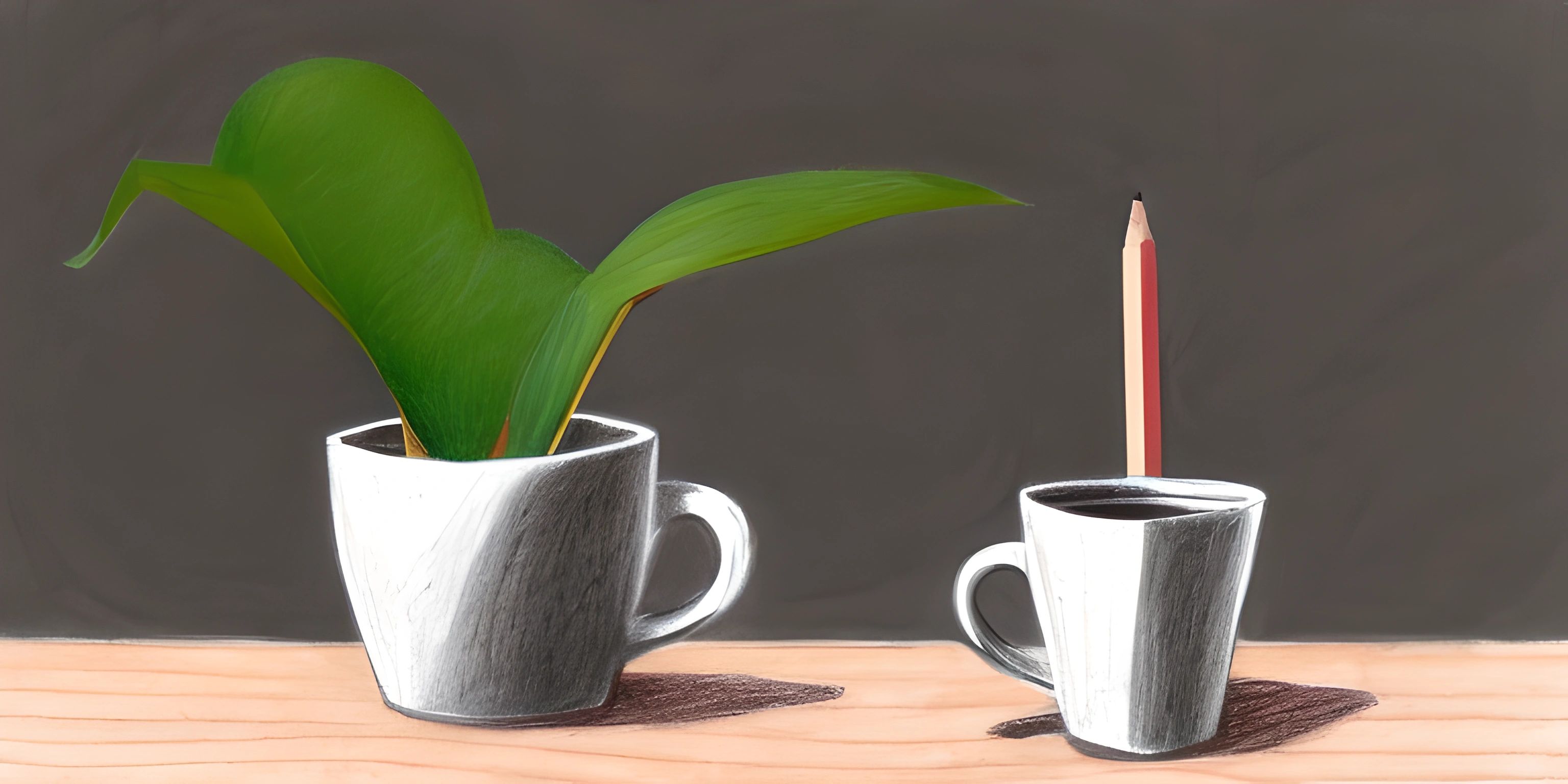
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Writing clean, readable, and scalable code is essential for every programmer. By following best programming practices, you can create code that is easy to understand, maintain, and extend. This article will provide you with some tips and tricks to help you write better code.
Write Meaningful Names
Choosing meaningful names for your variables, functions, and classes is crucial for readability. This will make it easier for others (and yourself) to understand what the code does without needing to read through comments or documentation.
// Bad example let x = 10; function f() { return x * 2; } // Good example let baseSalary = 10; function calculateDoubleSalary() { return baseSalary * 2; }
Keep Functions and Classes Simple
Try to keep your functions and classes small and focused on a single task. This makes them easier to understand, test, and maintain. If you find that a function is doing too many things, consider breaking it up into smaller functions.
// Bad example function processData(input) { // Step 1: Validate input // Step 2: Transform input // Step 3: Save input to database } // Good example function validateInput(input) { // Validate input } function transformInput(input) { // Transform input } function saveInputToDatabase(input) { // Save input to database } function processData(input) { validateInput(input); let transformedInput = transformInput(input); saveInputToDatabase(transformedInput); }
Write Comments
Write comments to explain the purpose of your code, especially when it's complex or not immediately obvious. However, avoid writing comments that simply repeat what the code does. Instead, focus on explaining why the code is doing something.
// Bad example let x = 10; // Set x to 10 // Good example let x = 10; // The base value used for calculations
Use Consistent Formatting
Consistent formatting, such as indentation and spacing, makes your code easier to read. Most programming languages have a style guide that you can follow. When working in a team, it's important to agree on a common style and stick to it.
// Bad example if(condition){ // Code }else{ // Code } // Good example if (condition) { // Code } else { // Code }
Write Tests
Writing tests for your code helps ensure that it works correctly and makes it easier to catch bugs before they become a problem. Automated tests also make it easier to refactor and extend your code without fear of breaking existing functionality.
function add(a, b) { return a + b; } // Test the "add" function console.assert(add(1, 2) === 3, "The add function should return the sum of its arguments");
By following these best programming practices, you will write code that is easier to understand, maintain, and extend. This will not only benefit you but also your teammates and future collaborators.
FAQ
What are some key aspects of writing clean, readable code?
Writing clean and readable code involves:
- Consistent indentation and spacing.
- Descriptive and concise variable and function names.
- Breaking down complex problems into smaller, manageable functions.
- Properly commenting and documenting your code.
- Organizing code into modules or classes, based on functionality.
How does following best programming practices improve code scalability?
Following best programming practices improves code scalability by:
- Making it easier for other developers to understand and maintain the code.
- Reducing the chances of introducing bugs when making changes.
- Allowing for easier implementation of new features.
- Encouraging modular design, which promotes reusability and adaptability.
What role do comments and documentation play in best programming practices?
Comments and documentation are crucial for providing context and explaining the purpose of your code, making it more understandable for others (or even yourself) in the future. They should explain the "why" behind your code, rather than just describing "what" the code does. Well-written comments and documentation can save time and effort when troubleshooting or updating the code later on.
Can you give an example of a best practice for naming variables and functions?
A best practice for naming variables and functions is to use descriptive, concise names that clearly indicate their purpose. For example, instead of naming a variable "x", name it "user_age". Similarly, instead of naming a function "func()", name it "calculate_average()". This makes the code more self-explanatory and easier to understand.
How can I ensure consistency in my code's indentation and spacing?
To ensure consistency in your code's indentation and spacing, follow a specific style guide or use a linter or formatter tool, like Prettier for JavaScript or Black for Python. These tools can be integrated with your code editor, automatically formatting your code according to the chosen style guide, which will help maintain consistency throughout your project.